Troubleshooting Common Issues with Java 8 Lambdas
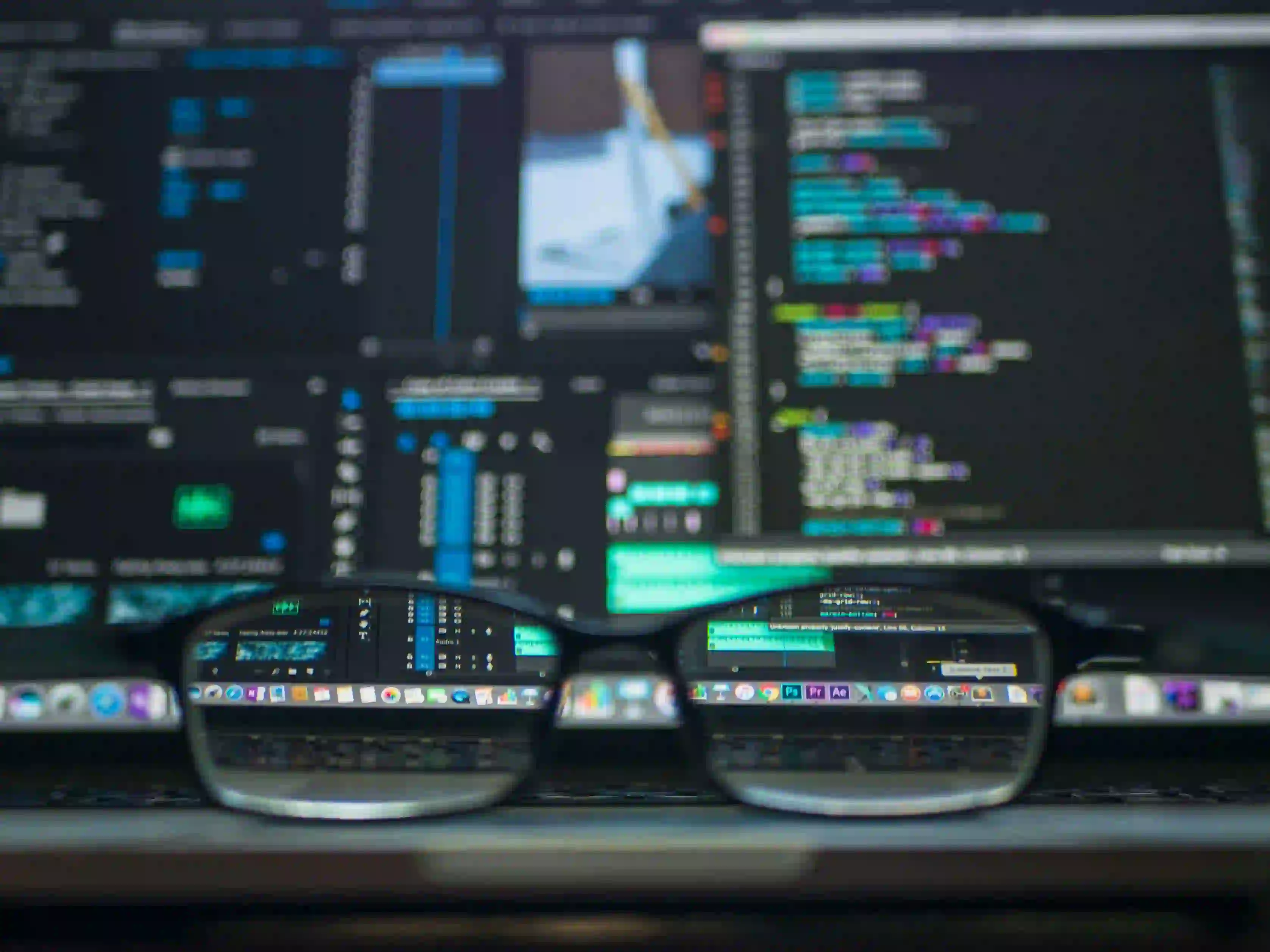
Troubleshooting Common Issues with Java 8 Lambdas
Java 8 introduced a powerful feature called Lambda expressions, which allows developers to write more concise and readable code. However, like any new feature, using Lambdas can sometimes lead to unexpected issues. In this post, we'll explore some common problems that developers might encounter when using Java 8 Lambdas and discuss how to troubleshoot and resolve them.
Issue 1: Variable Capture
Lambda expressions capture variables from their enclosing scope. When dealing with local variables or parameters, they must be effectively final, meaning their value cannot be changed after it has been assigned. If this rule is violated, it can lead to compiler errors.
Example of Variable Capture Issue:
public void captureVariableIssue() {
int x = 10;
Runnable r = () -> {
System.out.println(x);
// x++; // Trying to modify x will result in a compilation error
};
}
In this example, if you try to modify the value of x
inside the lambda expression, a compilation error will occur. This is because x
is not effectively final.
Solution:
To avoid this issue, ensure that the variables captured by the Lambda expressions are effectively final. If you need to modify a variable's value, consider using a different approach, such as using a mutable object like AtomicInteger
.
Issue 2: Functional Interface Compatibility
Lambda expressions in Java are only compatible with functional interfaces, which have a single abstract method. If a functional interface is modified and a second abstract method is added, it can break compatibility with existing Lambda expressions.
Example of Functional Interface Compatibility Issue:
@FunctionalInterface
interface Calculator {
int calculate(int x, int y);
// Adding a second abstract method will break compatibility with existing Lambda expressions
// int multiply(int x, int y);
}
In this case, adding the multiply
method will cause a compilation error for any existing Lambda expressions that implement the Calculator
interface.
Solution:
When working with functional interfaces, always ensure that they have only one abstract method. If you need to add more functionality, consider creating a new functional interface or using default methods to provide additional behavior.
Issue 3: Target Typing
Java 8 introduced a concept called target typing, which allows the compiler to infer the type of a Lambda expression based on the context in which it is used. However, there are scenarios where the compiler may struggle to infer the correct type, leading to compilation errors.
Example of Target Typing Issue:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.stream()
.map(name -> name.length()) // Compilation error: Required type: Function<? super String, ? extends R>
.forEach(System.out::println);
In this example, the map
function expects a Function
that accepts a String
and returns a result of type R
. However, the compiler struggles to infer the correct type for the lambda expression name -> name.length()
, resulting in a compilation error.
Solution:
To resolve this issue, provide explicit type information for the Lambda expression, or use method references where possible. In this example, the issue can be resolved by using a method reference String::length
instead of a Lambda expression.
Issue 4: Capturing this
Reference
When using Lambdas within an instance method of a class, it's important to be aware of how the this
reference is captured. If not used correctly, capturing the this
reference within a Lambda expression can lead to unexpected behavior.
Example of Capturing this
Reference Issue:
public class MyClass {
private int value;
public void myMethod() {
Runnable r = () -> {
// Attempting to modify the 'value' will result in a compilation error
// this.value = 10;
};
}
}
In this example, if you try to modify the value
field within the Lambda expression, a compilation error will occur. This is because the this
reference within the Lambda expression does not refer to an instance of MyClass
.
Solution:
To access the this
reference of the enclosing instance within a Lambda expression, use the syntax MyClass.this
to make it explicit. For example, MyClass.this.value = 10;
.
Key Takeaways
Java 8 Lambdas bring a powerful new way of writing concise and readable code. However, they also introduce new challenges and potential pitfalls. By understanding and addressing these common issues, developers can make the most of Lambda expressions while avoiding unexpected problems.
In summary, pay attention to variable capture, ensure compatibility with functional interfaces, be mindful of target typing, and properly handle the this
reference when using Lambdas. By following these best practices, developers can troubleshoot and resolve common issues encountered when working with Java 8 Lambdas.
Remember, practice and experience are key to mastering complex concepts like Lambdas. So, keep experimenting and learning to become more confident in using this powerful feature.
For further reading on Java 8 Lambdas and functional programming concepts, check out these resources:
- Oracle's official documentation on Lambda Expressions
- Functional Programming in Java: Harnessing the Power of Java 8 Lambda Expressions
Happy coding!