Troubleshooting Google Cloud Service Errors in Java
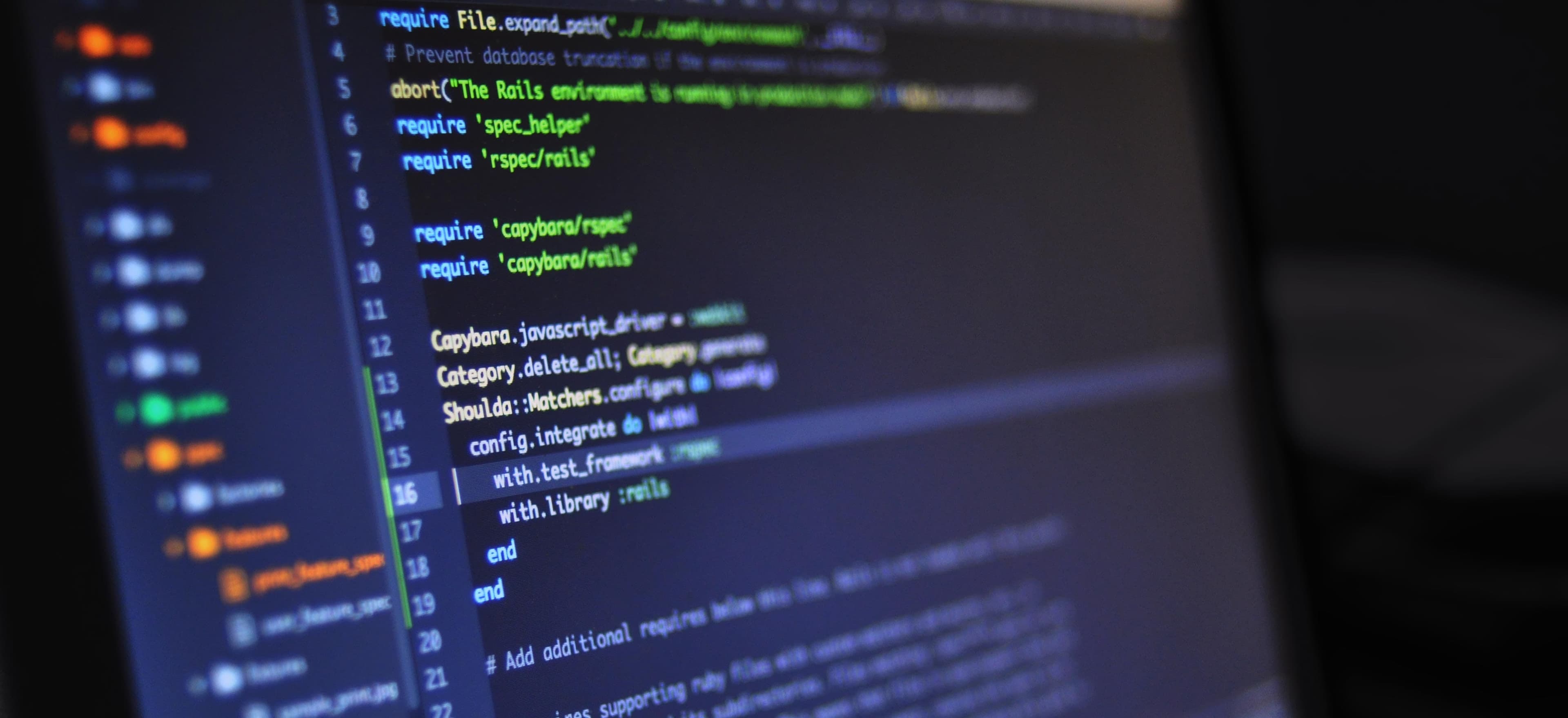
- Published on
Troubleshooting Google Cloud Service Errors in Java
When developing applications that leverage Google Cloud services in Java, encountering errors is an inevitable part of the process. While troubleshooting these errors may seem daunting at first, having a systematic approach and utilizing the right tools can make the task more manageable.
In this article, we will explore common errors that developers may encounter when working with Google Cloud services in Java and discuss effective troubleshooting techniques to resolve them.
Understanding Google Cloud Service Errors
Google Cloud Platform (GCP) offers a wide range of services, including Cloud Storage, Pub/Sub, BigQuery, and many more. When interacting with these services from a Java application, errors can occur due to various reasons such as network issues, authentication problems, or incorrect usage of the GCP client libraries.
Common error types that developers may encounter include:
-
Authentication Errors: These errors occur when there are issues with the authentication credentials used to access Google Cloud services.
-
Network Errors: Problems related to network connectivity or timeouts when trying to communicate with Google Cloud services.
-
Permission Errors: Occur when the application does not have the necessary permissions to perform certain actions within GCP.
-
Client Library Errors: Issues related to incorrect usage of Google Cloud client libraries or outdated library versions.
Troubleshooting Common Errors
1. Authentication Errors
Authentication is a critical aspect of accessing Google Cloud services. When facing authentication errors, it's important to ensure that the correct credentials are being used and that the application has the necessary permissions.
Validate Service Account Credentials
import com.google.auth.oauth2.GoogleCredentials;
import com.google.auth.oauth2.ServiceAccountCredentials;
// Load the credentials from the JSON key file
GoogleCredentials credentials = ServiceAccountCredentials.fromStream(new FileInputStream("path/to/credentials.json"));
// Validate the loaded credentials
if (credentials != null) {
// Use the credentials to access Google Cloud services
} else {
// Handle invalid credentials error
}
Grant Sufficient Permissions
Ensure that the service account used by the application has been granted the required IAM roles and permissions in the Google Cloud Console.
2. Network Errors
Network errors can occur due to factors such as connectivity issues or timeouts when communicating with Google Cloud services. When troubleshooting network errors, it's essential to consider both the client-side and server-side aspects of the communication.
Retry Logic for Network Errors
import com.google.api.gax.rpc.FixedHeaderProvider;
import com.google.api.gax.rpc.TransportChannelProvider;
import com.google.api.gax.rpc.TransportChannelProvider.Builder;
import com.google.api.gax.rpc.StatusCode;
import com.google.api.gax.rpc.StatusCode.Code;
import com.google.api.gax.rpc.RetrySettings;
import com.google.api.gax.rpc.FixedHeaderProvider;
// Configure a custom TransportChannelProvider with retry settings
Builder channelBuilder = FixedTransportChannelProvider.create()
.setRetrySettings(
RetrySettings.newBuilder()
.setMaxAttempts(3)
.setTotalTimeout(Duration.ofSeconds(30))
.build());
// Use the custom TransportChannelProvider when creating the client
MyServiceClient client = MyServiceClient.create(
MyServiceSettings.newBuilder().setTransportChannelProvider(channelBuilder.build()).build());
By configuring retry settings, the application can automatically retry requests in case of transient network errors.
3. Permission Errors
Permission errors arise when the application lacks the necessary permissions to perform specific actions within Google Cloud services. It's essential to verify that the service account used has been granted the appropriate IAM roles and permissions.
Check IAM Role Assignments
import com.google.cloud.Identity;
import com.google.cloud.Role;
import com.google.cloud.Policy;
import com.google.cloud.ServiceOptions;
import com.google.cloud.StorageOptions;
import com.google.cloud.storage.BlobId;
import com.google.cloud.storage.Storage;
import com.google.cloud.storage.StorageOptions;
// Get the current policy for a bucket
Storage storage = StorageOptions.getDefaultInstance().getService();
String bucketName = "your-bucket-name";
Policy policy = storage.getIamPolicy(bucketName);
// Check if the service account has the required role
Role role = Role.of("roles/storage.objectCreator");
boolean hasRole = policy.getBindings()
.stream()
.anyMatch(binding -> binding.getMembers().contains(Identity.serviceAccount("your-service-account@project-id.iam.gserviceaccount.com")));
By checking the IAM role assignments, the application can ensure that the necessary permissions are in place for the service account.
4. Client Library Errors
Client library errors can stem from issues such as deprecated methods, outdated library versions, or incorrect usage of the client libraries provided by Google Cloud. When encountering client library errors, it's crucial to review the documentation and ensure that the correct usage patterns are being followed.
Update to the Latest Client Library Version
Ensure that the Java application is using the latest version of the Google Cloud client libraries to leverage bug fixes and new features. Review the release notes for the library to identify any breaking changes or migration steps when updating to a new version.
My Closing Thoughts on the Matter
Troubleshooting Google Cloud service errors in Java requires a methodical approach that encompasses authentication, network connectivity, permissions, and client library usage. By understanding the common error types and applying the appropriate troubleshooting techniques, developers can effectively diagnose and resolve issues encountered when integrating with Google Cloud Platform.
Remember to leverage the official Google Cloud documentation and Stack Overflow as valuable resources when troubleshooting specific error scenarios.
By mastering the art of troubleshooting, developers can build robust and reliable Java applications that seamlessly interact with Google Cloud services.