Creating Transparent JFrame Using JNA
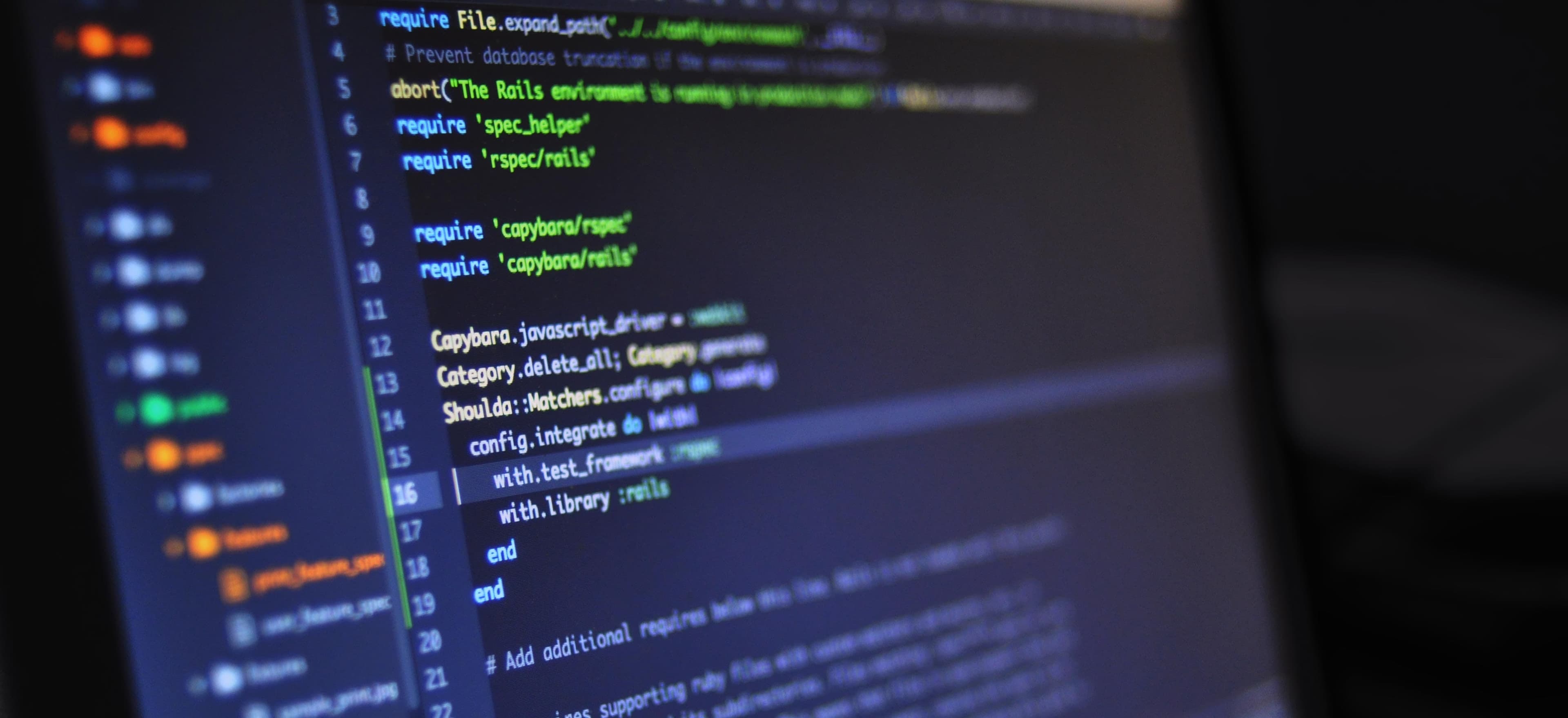
- Published on
Creating Transparent JFrame Using JNA
In Java, creating a transparent JFrame
can be an interesting task. You may want to achieve this to create a modern user interface or to overlay a transparent window on top of another application. In this tutorial, we'll explore how to create a transparent JFrame
using the Java Native Access (JNA) library.
What is JNA?
JNA is a Java library that provides access to native libraries without the need for JNI (Java Native Interface) code. It allows Java applications to access and call functions in native libraries.
Why Use JNA for Creating Transparent JFrame?
When attempting to create a transparent JFrame
in Java, you'll find that it's not directly supported by the standard Swing API. JNA provides a way to access functionality in the native windowing system, which allows us to achieve transparency.
Let's dive into the code to see how this is done.
Setting Up the Project
Before we start, make sure to have JNA added to your project's dependencies. You can add it manually or use a build tool such as Maven or Gradle. Here's the Maven dependency for JNA:
<dependency>
<groupId>net.java.dev.jna</groupId>
<artifactId>jna</artifactId>
<version>5.9.0</version>
</dependency>
Creating a Transparent JFrame
First, we create a new Java class called TransparentJFrame
:
import com.sun.jna.Native;
import com.sun.jna.platform.win32.User32;
import com.sun.jna.platform.win32.WinDef.HWND;
import javax.swing.*;
import java.awt.*;
public class TransparentJFrame extends JFrame {
public TransparentJFrame() {
this.setUndecorated(true);
this.setBackground(new Color(0, 0, 0, 0)); // Full transparency
}
public void makeTransparent() {
HWND hwnd = new HWND(Native.getWindowPointer(this));
User32.INSTANCE.SetWindowLong(hwnd, User32.GWL_EXSTYLE,
User32.INSTANCE.GetWindowLong(hwnd, User32.GWL_EXSTYLE) | User32.WS_EX_LAYERED);
User32.INSTANCE.SetLayeredWindowAttributes(hwnd, 0, (byte) 255, User32.LWA_ALPHA);
}
public static void main(String[] args) {
TransparentJFrame frame = new TransparentJFrame();
frame.setSize(400, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
frame.makeTransparent();
}
}
In this code, we define a class TransparentJFrame
that extends JFrame
. In the constructor, we set the frame to be undecorated and set its background color to fully transparent using new Color(0, 0, 0, 0)
. This creates a transparent window with no borders.
The makeTransparent
method uses JNA to set the window style to layered and apply the alpha value for transparency.
Explanation of the Code
Setting the Frame as Undecorated
By calling setUndecorated(true)
, we remove the window decorations such as the title bar and borders, allowing us to have a truly custom window appearance.
Setting Background Color to Transparent
The background color is set using new Color(0, 0, 0, 0)
, where the first three parameters represent the RGB values (in this case, all zeros for black) and the last parameter represents the alpha value, which controls the transparency (0 being fully transparent and 255 being fully opaque).
Using JNA to Make the Frame Transparent
The makeTransparent
method uses JNA to access native platform functions. It obtains the window handle (HWND) using Native.getWindowPointer(this)
, then uses JNA's User32
interface to set the window style to layered and apply the alpha value using SetLayeredWindowAttributes
.
Final Considerations
In this tutorial, we explored how to create a transparent JFrame
in Java using the JNA library. We leveraged JNA to interact with native platform functions and achieve the desired transparency effect. This technique can be useful for creating custom and modern user interfaces or for overlaying transparent windows in desktop applications.
Now that you have a transparent JFrame
, you can further enhance it by adding graphics, animations, or integrating it with other GUI components.
For more information on JNA and its capabilities, you can visit the JNA documentation.
Give this approach a try and see how transparent frames can elevate your Java GUI applications!
Checkout our other articles