Overlooked Coding Pitfalls: When Comments Backfire
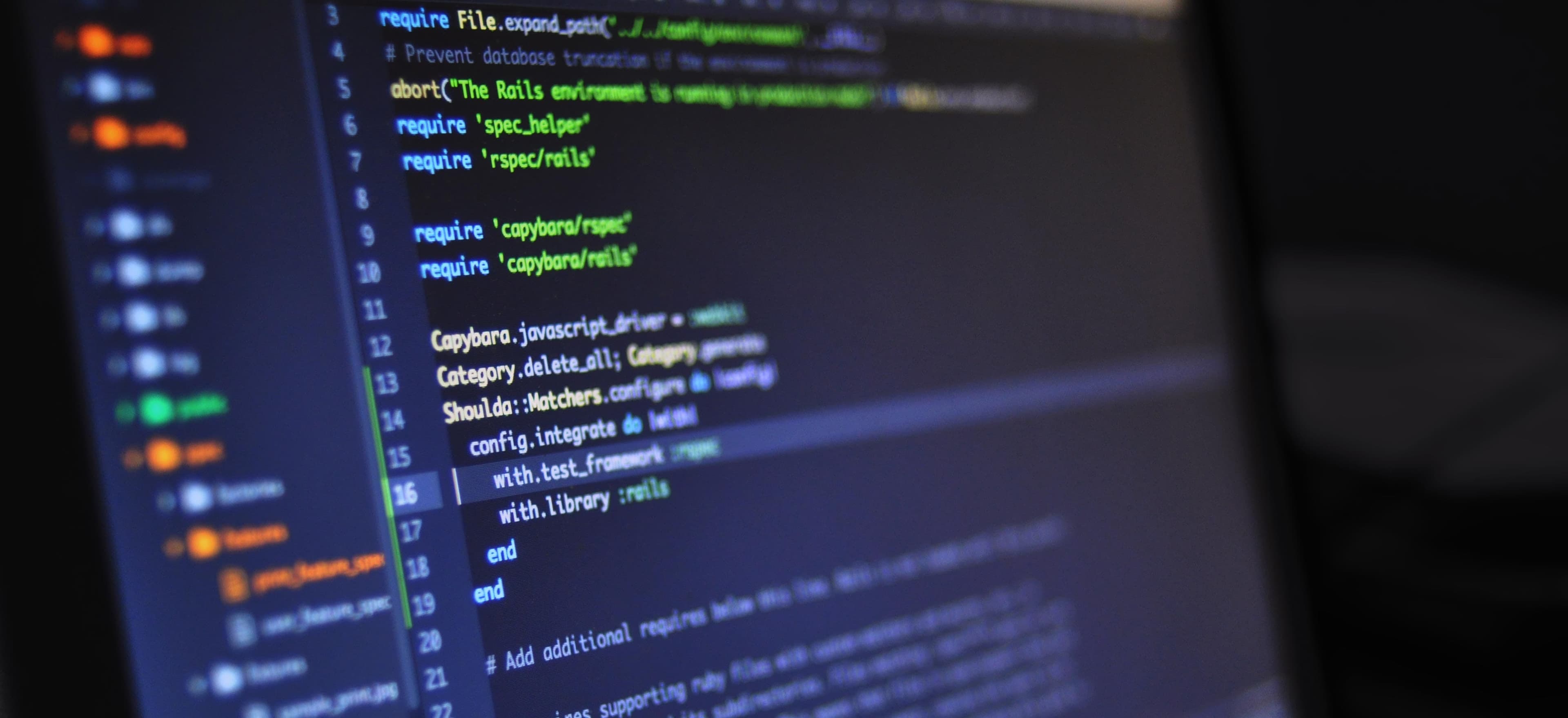
- Published on
Overlooked Coding Pitfalls: When Comments Backfire
When it comes to writing code, comments are often hailed as a best practice. They guide developers through the logic, provide clarity, and make it easier to maintain the codebase. However, there are situations where comments can have the opposite effect, leading to confusion and inefficiency. In this post, we'll explore some common pitfalls associated with comments in Java programming and how to avoid them.
The Pitfall of Outdated Comments
One of the most common issues with comments is their tendency to become outdated. Consider the following example:
// Increment the counter by 1
counter = counter + 1;
At first glance, the comment seems harmless and helpful. However, if the code evolves and the increment operation is changed, the comment becomes misleading. Developers may rely on the comment and miss the updated logic, leading to unintended consequences. Instead, writing self-explanatory code can mitigate the need for such comments:
counter++;
In this case, the intention is clear, eliminating the risk of outdated comments.
The Ambiguity of Explanatory Comments
Explanatory comments, while well-intentioned, can sometimes introduce ambiguity rather than clarity. For instance:
// Calculate the final price after applying the discount
double finalPrice = calculateFinalPrice(initialPrice, discount);
This comment aims to clarify the purpose of the code, but it doesn't actually explain how the final price is calculated. As a result, it adds little value and may even confuse readers. A better approach is to write expressive code and use meaningful method and variable names:
double finalPrice = applyDiscount(initialPrice, discount);
By naming the method and variables descriptively, the need for an explanatory comment diminishes, leading to more understandable and maintainable code.
The Trap of Redundant Comments
Redundant comments are another pitfall that can clutter the codebase without providing any real insight. Consider the following example:
// Validate the input
if (input != null) {
// Process the input
processInput(input);
}
In this case, the comments merely echo the code, offering no additional information. Such redundant comments create visual noise and can make the code harder to read. Instead, focus on writing concise and expressive code that speaks for itself:
if (input != null) {
processInput(input);
}
By removing redundant comments, the code becomes cleaner and more focused.
The Misuse of Block Comments
Block comments are essential for providing context at the file or method level. However, misuse of block comments within the code can be detrimental. Consider the following example:
/*
* This method calculates the total price
* It takes the initial price and applies the discount
*/
double calculateTotalPrice(double initialPrice, double discount) {
// ...
}
While the block comment conveys the purpose of the method, it adds little value inside the method. In such cases, it's better to rely on method signatures, expressive method names, and inline comments for any specific details within the method body:
double calculateTotalPrice(double initialPrice, double discount) {
// Apply discount to the initial price
// ...
}
By using block comments judiciously and preferring inline comments for specific details, the code remains focused and easier to comprehend.
Final Considerations
Comments in Java programming, when misused or overused, can lead to confusion and inefficiency. By being mindful of the pitfalls associated with comments and focusing on writing expressive and self-explanatory code, developers can create more maintainable and readable codebases. Remember, while comments can be valuable, the ultimate goal is to write code that communicates its intent clearly on its own.
In conclusion, always strive to write code that minimizes the need for comments, making them supplemental rather than essential for understanding the logic. This approach not only reduces the risk of outdated, ambiguous, and redundant comments but also fosters a more streamlined and efficient coding experience.
For further reading on Java best practices and code optimization, consider this insightful article on Java Performance Optimization.
As you continue to enhance your Java programming skills, keep in mind the impact of comments on code readability and maintenance, and strive for a balanced approach that prioritizes clear, concise, and expressive code.
Happy coding!
Checkout our other articles