Dealing with Circular Dependencies in Blueprint and Declarative Services
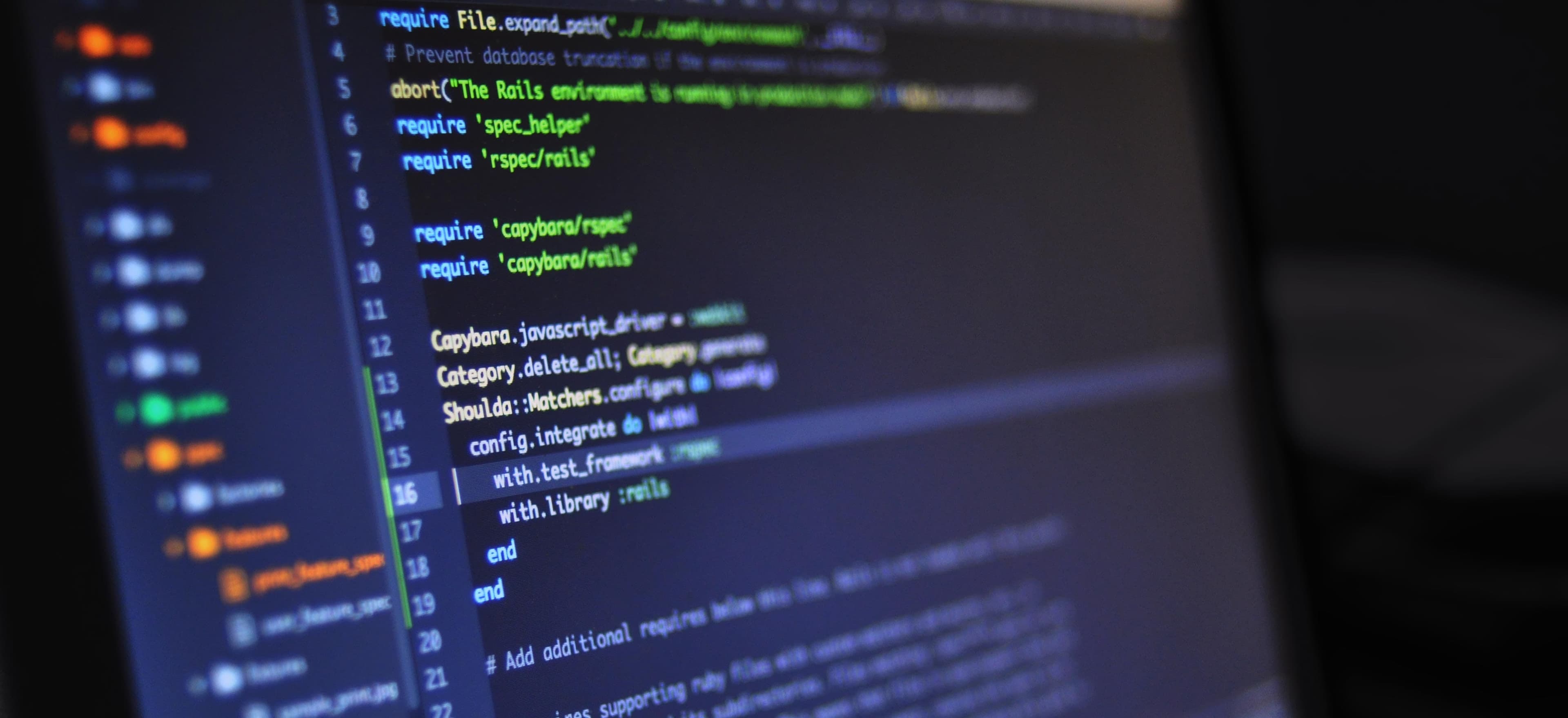
- Published on
Dealing with Circular Dependencies in Blueprint and Declarative Services
Circular dependencies occur when two or more components depend on each other directly or indirectly, causing a deadlock situation during component instantiation. In this blog post, we will discuss how to identify and resolve circular dependencies in Java applications using Blueprint and Declarative Services, and how to design components to minimize the risk of circular dependencies.
What are Blueprint and Declarative Services?
Blueprint is a service provided by the OSGi (Open Services Gateway initiative) specification that allows developers to define the structure and configuration of an application using XML files. It is commonly used in OSGi-based applications to define and manage the lifecycle of components and services.
Declarative Services is another OSGi-based service that provides a way to define and manage the dependencies between components using annotations and XML configuration. It simplifies the process of building OSGi applications by allowing components to be created and managed in a more declarative and less verbose way.
Identifying Circular Dependencies
Circular dependencies can often be difficult to spot in large projects, and they can lead to runtime exceptions or unexpected behavior. In OSGi-based applications, Blueprint and Declarative Services provide a structure to manage such dependencies, but it's still important for developers to be aware of potential circular dependencies and how to address them.
Here's an example of a simple circular dependency between two components:
public class ComponentA {
private ComponentB b;
public ComponentA(ComponentB b) {
this.b = b;
}
}
public class ComponentB {
private ComponentA a;
public ComponentB(ComponentA a) {
this.a = a;
}
}
In this example, ComponentA
depends on ComponentB
, and ComponentB
depends on ComponentA
, creating a circular reference.
Resolving Circular Dependencies in Blueprint
One way to resolve circular dependencies in Blueprint is by using callbacks. Callbacks allow components to be notified when their dependencies are satisfied, which can be used to break circular dependencies. Let's see how this can be achieved:
<bean id="componentA" class="com.example.ComponentA">
<property name="b" ref="componentB"/>
</bean>
<bean id="componentB" class="com.example.ComponentB">
<property name="a" ref="componentA"/>
</bean>
In this example, we have broken the circular dependency by not injecting the dependency directly into the constructor, but instead using a setter method to inject the dependency.
Resolving Circular Dependencies in Declarative Services
In Declarative Services, circular dependencies are resolved using a similar approach to the one used in Blueprint - by using service callbacks. Let's take a look at an example:
@Component
public class ComponentA {
@Reference
private ComponentB b;
@Activate
public void activate() {
b.setA(this);
}
}
@Component
public class ComponentB {
private ComponentA a;
@Reference
public void setA(ComponentA a) {
this.a = a;
}
}
In this example, we have used the @Activate
annotation to call a method when the component is activated, and we have used a setter method to inject the circular dependency.
Designing Components to Minimize Circular Dependencies
While resolving circular dependencies is important, it's equally important to design components in a way that minimizes the risk of circular dependencies.
One way to do this is by using interfaces to decouple components from each other. By defining interfaces for components and using them as the type of the dependencies, you can reduce the likelihood of circular dependencies.
public interface ComponentAService {
// methods
}
public interface ComponentBService {
// methods
}
@Component
public class ComponentA implements ComponentAService {
@Reference
private ComponentBService b;
}
@Component
public class ComponentB implements ComponentBService {
@Reference
private ComponentAService a;
}
By using interfaces, each component only depends on the interface of the other component, rather than the concrete implementation, which helps break the circular reference.
Final Thoughts
In OSGi-based applications, circular dependencies can lead to runtime issues and unexpected behavior. However, by understanding how to identify and resolve circular dependencies in Blueprint and Declarative Services, and by designing components to minimize the risk of circular dependencies, developers can build more robust and maintainable applications.
By using callbacks, service annotations, and interfaces, developers can manage dependencies more effectively and reduce the risk of circular dependencies in their Java applications.
For further reading on circular dependencies and OSGi-based services, you can refer to the OSGi Alliance website.
Checkout our other articles