Implementing Server-Sent Events in JAX-RS: Troubleshooting Connection Delay
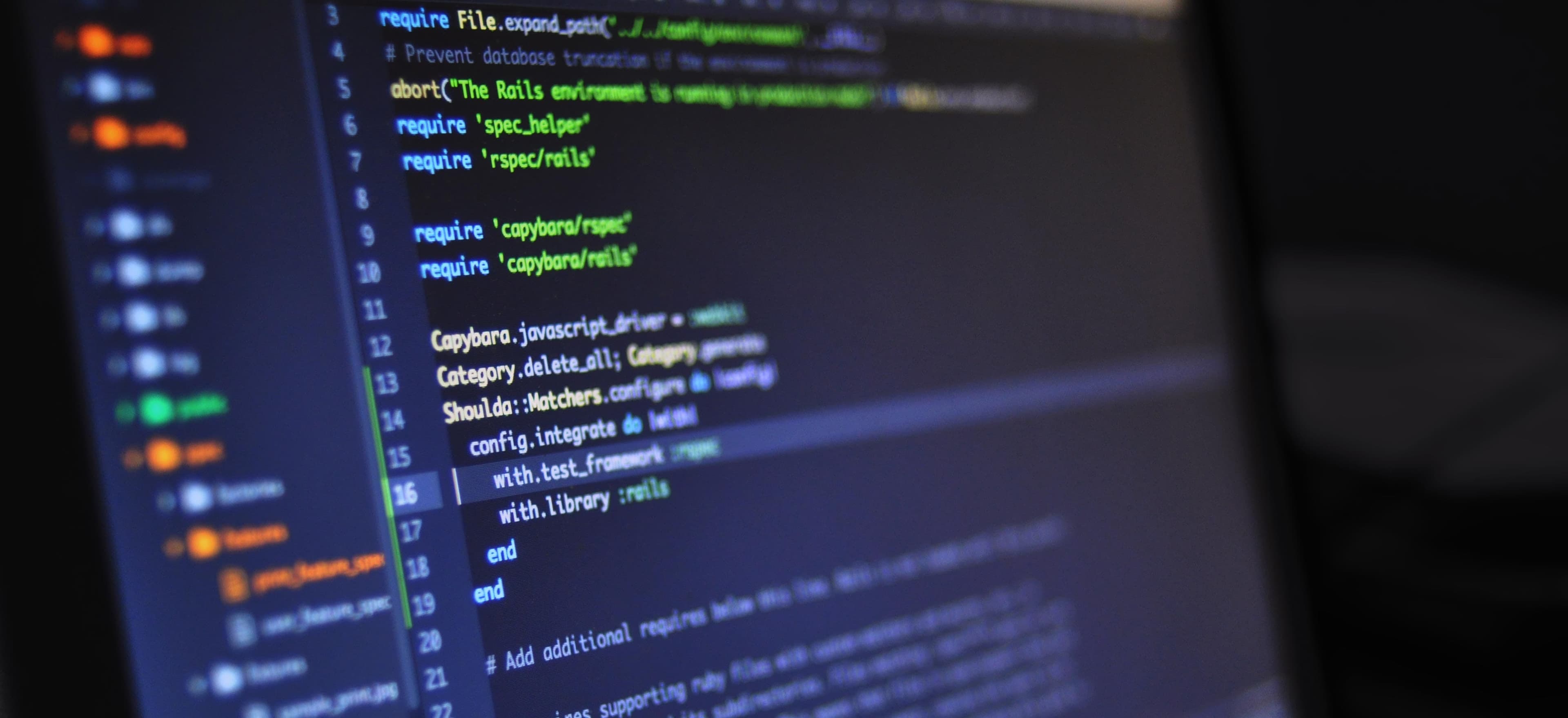
- Published on
Implementing Server-Sent Events in JAX-RS: Troubleshooting Connection Delay
Implementing Server-Sent Events (SSE) in Java API for RESTful Web Services (JAX-RS) can provide a real-time connection from the server to the client. SSE is particularly useful for scenarios where the server needs to push updates to the client without the client requesting them.
However, implementing SSE in JAX-RS can sometimes come with challenges. One common issue that developers face is the delay in establishing a connection between the server and the client. In this article, we'll explore some potential causes of connection delay when implementing SSE in JAX-RS and discuss troubleshooting techniques to address the issue.
Understanding the Issue
Before delving into troubleshooting, it's essential to understand why connection delay may occur when implementing SSE in JAX-RS. SSE operates over a single, long-lived HTTP connection, and the server uses this connection to send data to the client intermittently. While SSE provides a persistent connection, certain factors can lead to delays in establishing and maintaining this connection.
Potential Causes of Connection Delay
Several factors can contribute to connection delay when implementing SSE in JAX-RS. Understanding these factors can help in identifying the root cause of the issue. Some potential causes include:
Server Configuration
The server's configuration, such as the maximum number of concurrent connections or the timeout settings, can impact the SSE connection establishment. If the server is configured with overly restrictive settings, it may cause delays in establishing SSE connections.
Network Latency
Network latency, including issues such as packet loss, high latency, or network congestion, can impact the SSE connection's responsiveness. When dealing with a high-latency network, the establishment of SSE connections may experience delays.
Client-Side Implementation
The client's SSE implementation, including the handling of incoming data and connection error scenarios, can also contribute to connection delays. If the client is not efficiently processing incoming SSE data, it may lead to delay in establishing subsequent connections.
Troubleshooting Connection Delay
Now that we've identified potential causes of connection delay when implementing SSE in JAX-RS, let's explore troubleshooting techniques to address the issue.
Increase Server Timeout Settings
One way to address connection delay is by increasing the server's timeout settings for HTTP connections. When the server has a low timeout threshold, it may prematurely terminate SSE connections, leading to delays in re-establishing the connection. By increasing the timeout settings, such as the maximum connection and read timeouts, the server allows more time for the SSE connection to be established and maintained.
@Path("/sse-endpoint")
public class SSEEndpoint {
@GET
@Produces(MediaType.SERVER_SENT_EVENTS)
public void subscribeToEvents(@Context SseEventSink eventSink) {
// Increase the server timeout settings
eventSink.send(sse.newEvent("Connection established"));
}
}
In the above code snippet, we can see that the server's timeout settings for HTTP connections are increased, allowing more time for the SSE connection to be established and maintained.
Monitor Network Latency
Monitoring and addressing network latency issues can significantly improve the responsiveness of SSE connections. By employing network monitoring tools and addressing latency issues, such as optimizing network configurations or addressing network congestion, the delay in establishing SSE connections can be mitigated.
Optimize Client-Side Implementation
Addressing potential delays in the client's SSE implementation is crucial for improving connection responsiveness. This includes optimizing the client's handling of incoming SSE data, ensuring efficient error handling, and implementing strategies to re-establish SSE connections promptly in the event of a connection failure.
The Bottom Line
Implementing SSE in JAX-RS provides a powerful mechanism for real-time data updates from the server to the client. However, connection delay issues can pose challenges in ensuring a responsive SSE connection. By understanding the potential causes of connection delay and employing troubleshooting techniques such as increasing server timeout settings, monitoring network latency, and optimizing the client-side implementation, developers can address and mitigate connection delay when implementing SSE in JAX-RS.
In conclusion, addressing connection delay in SSE implementation not only enhances the real-time capabilities of the application but also improves the overall user experience.
For further reading on JAX-RS and SSE, you can refer to the official Jersey documentation, which provides comprehensive insights into JAX-RS features and best practices for implementing SSE.
Implementing SSE in JAX-RS offers a seamless way to establish real-time connections between the server and client, and by troubleshooting connection delay effectively, developers can ensure a smooth and responsive user experience.