Dealing with Ambiguity: Solving CDI Qualifiers in Java EE
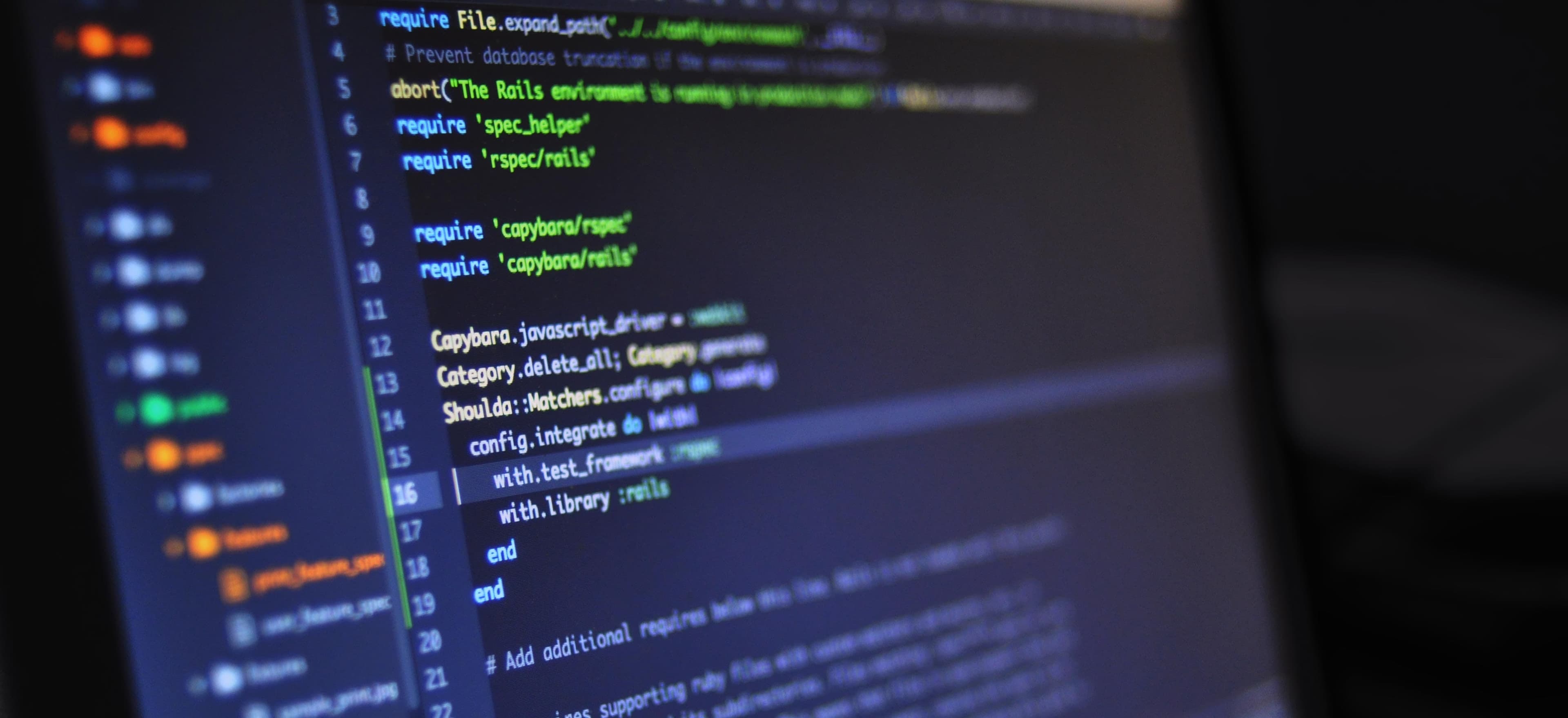
- Published on
Dealing with Ambiguity: Solving CDI Qualifiers in Java EE
When working with large and complex codebases in Java EE, you might encounter situations where the CDI (Context and Dependency Injection) container struggles to identify the right bean to inject due to the presence of multiple beans of the same type. This is known as the CDI qualifier ambiguity problem.
In this article, we will delve into the world of CDI qualifiers and how to effectively solve ambiguity issues. By the end of this post, you will have a comprehensive understanding of CDI qualifiers and be equipped with the knowledge to tackle ambiguity with confidence.
Understanding CDI Qualifiers
In Java EE, CDI qualifiers are used to disambiguate between multiple beans of the same type. Qualifiers are annotations that can be applied to beans to specify their intended use or purpose. When an injection point is encountered, the CDI container uses the qualifiers to determine the exact bean to be injected.
Let's consider a simple example where we have two beans of the same type Message
:
@Default
@ApplicationScoped
public class DefaultMessage implements Message {
// Implementation
}
@Special
@ApplicationScoped
public class SpecialMessage implements Message {
// Implementation
}
public interface Message {
String getMessage();
}
In the above example, @Default
and @Special
are CDI qualifiers applied to the beans DefaultMessage
and SpecialMessage
respectively.
Resolving Ambiguity with Qualifiers
1. Using Qualifiers at Injection Points
When injecting a bean, we can specify the qualifiers to be considered using the @Inject
annotation. By providing the qualifiers at the injection point, we explicitly tell the CDI container which bean to inject.
@Inject
@Default
private Message defaultMessage;
In the above code, the @Default
qualifier is used to resolve the ambiguity, indicating that the defaultMessage
should be injected with the DefaultMessage
bean.
2. Creating Custom Qualifiers
In scenarios where built-in qualifiers such as @Default
and @Any
are insufficient, we can create custom qualifiers by defining our own annotation types. This allows for more fine-grained control over the qualifiers and helps to differentiate between beans based on specific attributes.
@Qualifier
@Target({ElementType.TYPE, ElementType.FIELD, ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
public @interface Priority {
int value();
}
In the above example, we have defined a custom qualifier @Priority
that takes an integer value. We can then apply this qualifier to our beans and injection points, enabling precise resolution of ambiguity based on priorities.
3. Qualifier Annotation Inheritance
Qualifiers can also be inherited, allowing a bean with a specific qualifier to fulfill the needs of another. By leveraging this feature, we can effectively reuse qualifiers and prevent repetitive qualifier declarations across the codebase.
@Inherited
@Qualifier
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.TYPE, ElementType.FIELD, ElementType.METHOD})
public @interface HighPriority {
}
In the above example, @HighPriority
is a qualifier that inherits from a parent qualifier, allowing any bean annotated with @HighPriority
to also satisfy injection points expecting its parent qualifier.
A Final Look
In Java EE, tackling CDI qualifier ambiguity is crucial for maintaining a robust and manageable codebase. By understanding the nuances of CDI qualifiers and employing the techniques discussed in this article, you can effectively resolve ambiguity issues and ensure precise bean injection within your applications.
As you continue to work with Java EE and CDI, remember that clear and consistent qualifier usage not only eliminates ambiguity but also enhances the readability and maintainability of your code.
Now that you have a solid grasp of CDI qualifiers and ambiguity resolution, you are well-equipped to navigate the complex landscape of dependency injection within Java EE applications.
For further exploration, dive into the official Java EE documentation on CDI Qualifiers and broaden your understanding of this essential aspect of Java EE development.
Happy coding!