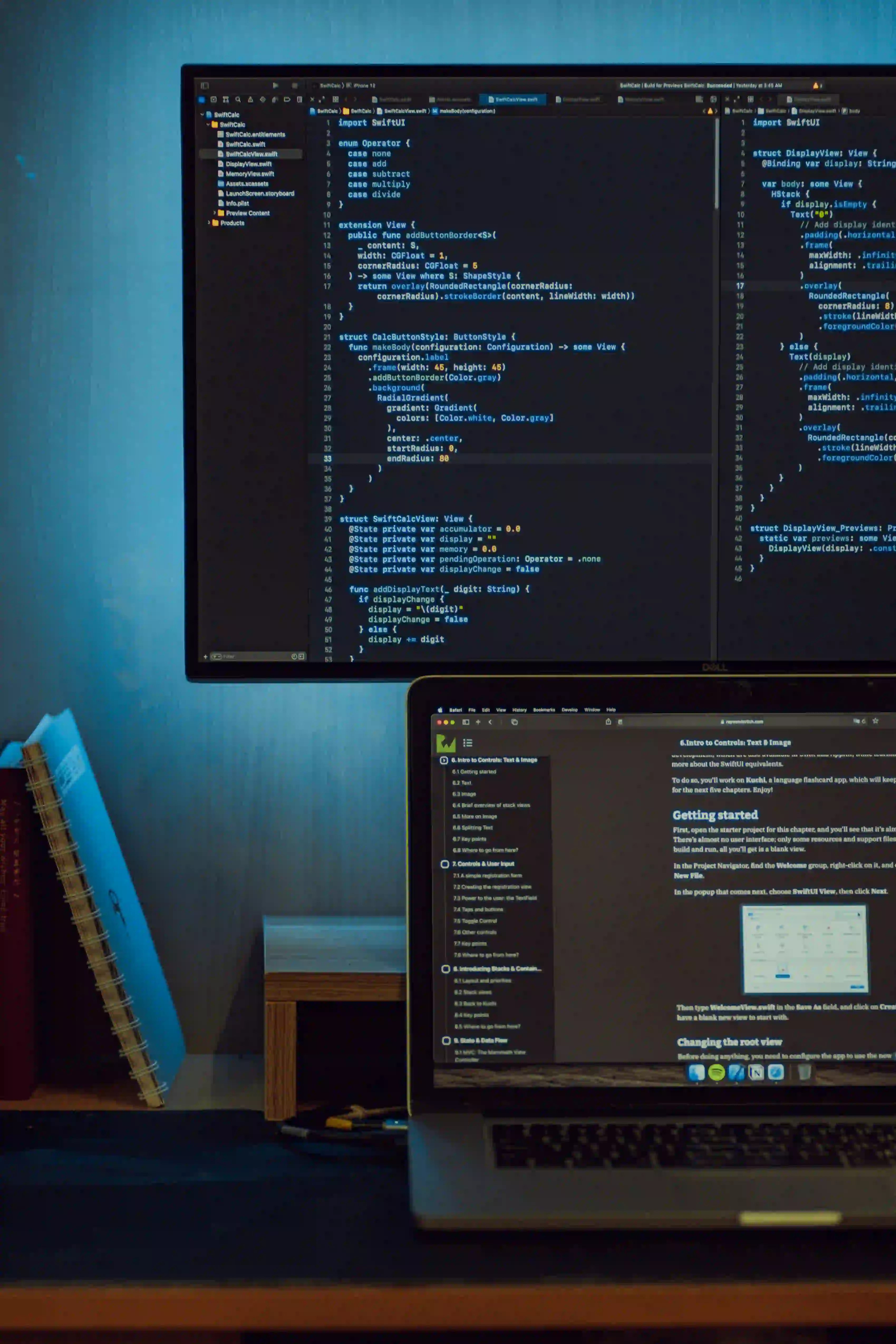
Handling Complex Web Service Security with Apache CXF
When it comes to building secure web services in Java, the Apache CXF framework is widely recognized for its robust security features. In this blog post, we will delve into the complexities of web service security and explore how Apache CXF can be used to handle these challenges effectively.
Understanding Web Service Security
Web service security is a critical aspect of modern application development. It involves ensuring that communication between web services and clients is protected from unauthorized access, data breaches, and other security threats. There are several dimensions to web service security, including:
- Authentication: Verifying the identity of clients and services.
- Authorization: Controlling access to resources based on the authenticated identity.
- Data Integrity: Ensuring that data exchanged between the client and the service remains unchanged.
- Confidentiality: Encrypting sensitive data to prevent unauthorized access.
Challenges in Web Service Security
Securing web services can be challenging, especially when dealing with complex scenarios such as:
- Multiple Authentication Mechanisms: Supporting various authentication methods, such as username/password, tokens, and digital certificates.
- Message-level Security: Encrypting and signing individual messages for end-to-end security.
- Interoperability: Ensuring that security measures are compatible with different platforms and technologies.
- Regulatory Compliance: Meeting the security standards and regulations specific to the industry or region.
Apache CXF for Web Service Security
Apache CXF is a powerful and flexible open-source web service framework that provides extensive support for building secure web services in Java. It offers a wide range of security features that can address the challenges mentioned above.
Integration with Standards-Based Security Protocols
CXF seamlessly integrates with standard security protocols such as WS-Security, WS-SecurityPolicy, and SAML, providing a comprehensive set of tools for securing web services. This allows developers to leverage industry-standard security mechanisms without reinventing the wheel.
Configurable Security Policies
CXF allows the definition of security policies declaratively using XML configuration. This enables fine-grained control over security settings such as encryption, digital signatures, and authentication requirements. By defining security policies, developers can ensure that their web services comply with specific security requirements.
Let's take a look at an example of configuring WS-SecurityPolicy in Apache CXF:
<jaxws:properties>
<entry key="ws-security.callback-handler" value="com.example.MyCallbackHandler"/>
<entry key="ws-security.encryption.properties" value="classpath:encryption.properties"/>
<entry key="ws-security.signature.properties" value="classpath:signature.properties"/>
<!-- Other security properties -->
</jaxws:properties>
In this configuration, we specify a callback handler for handling cryptographic keys and properties files for encryption and digital signatures. This demonstrates how CXF simplifies the management of security configurations.
Transport-Level Security
CXF provides built-in support for securing web services at the transport level, such as HTTPS. This ensures that data transmitted over the network is encrypted and protected from eavesdropping. By leveraging transport-level security, developers can establish a secure channel for communication without additional coding overhead.
Applying Apache CXF for Complex Web Service Security
Now, let's explore how Apache CXF can be applied to address complex web service security scenarios.
Implementing Mutual SSL Authentication
Mutual SSL authentication, also known as client certificate authentication, is a robust security measure that requires both the client and the server to present certificates to authenticate each other. CXF simplifies the implementation of mutual SSL authentication through its configuration options.
Here's an example of how mutual SSL authentication can be enabled in Apache CXF:
HTTPConduit conduit = (HTTPConduit) client.getConduit();
TLSClientParameters tlsParams = new TLSClientParameters();
KeyStore keyStore = KeyStore.getInstance("JKS");
keyStore.load(new FileInputStream("client.keystore"), "keystorePassword".toCharArray());
KeyManager[] myKeyManagers = getKeyManagers(keyStore, "keystorePassword");
tlsParams.setKeyManagers(myKeyManagers);
TrustManager[] myTrustStoreKeyManagers = getTrustManagers(keyStore);
tlsParams.setTrustManagers(myTrustStoreKeyManagers);
conduit.setTlsClientParameters(tlsParams);
In this snippet, we configure the client to present its keystore for mutual SSL authentication. CXF simplifies the integration of SSL/TLS capabilities into web service clients, making it easier to enforce strong mutual authentication.
Implementing Message-Level Security with WS-Security
Message-level security involves applying encryption and digital signatures to individual SOAP messages for end-to-end protection. Apache CXF provides robust support for message-level security through its integration with WS-Security.
Let's illustrate how to enable message-level security with WS-Security in Apache CXF:
<jaxws:properties>
<entry key="ws-security.callback-handler" value="com.example.MyCallbackHandler"/>
<entry key="ws-security.encryption.properties" value="classpath:encryption.properties"/>
<entry key="ws-security.signature.properties" value="classpath:signature.properties"/>
<entry key="ws-security.enable" value="true"/>
<!-- Other security properties -->
</jaxws:properties>
By configuring the WS-Security properties, developers can seamlessly enable message-level security within their web services. CXF takes care of the heavy lifting associated with message encryption and signature processing, allowing developers to focus on business logic.
The Bottom Line
Securing web services can be a daunting task, especially when dealing with complex security requirements. With its versatile security features, seamless protocol integration, and extensive customization options, Apache CXF emerges as a reliable choice for handling complex web service security in Java.
By leveraging Apache CXF, developers can build web services that adhere to stringent security standards, ensuring the confidentiality, integrity, and authenticity of data exchanged between clients and services. As the landscape of web service security continues to evolve, Apache CXF remains a steadfast ally for Java developers seeking to fortify their applications against security threats.
In conclusion, Apache CXF not only simplifies the implementation of web service security but also empowers developers to stay ahead in the realm of secure web service communication.
Start leveraging Apache CXF for robust web service security today!