Common Pitfalls of @Value Annotation in Spring
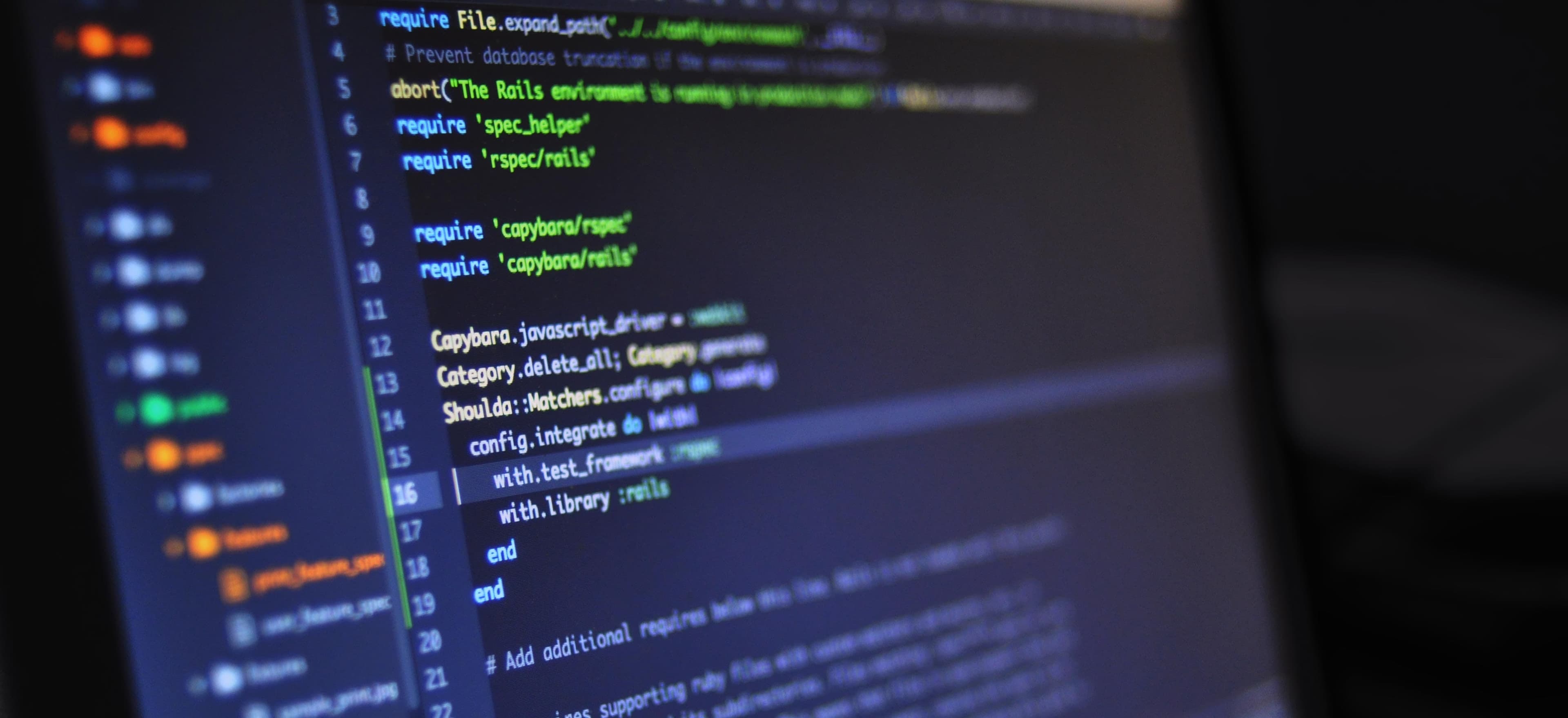
- Published on
Common Pitfalls of @Value Annotation in Spring
When working with the Spring Framework in Java, developers often rely on the @Value annotation to inject values into their beans. While this annotation provides a convenient way to externalize configuration, there are several common pitfalls that developers should be aware of. In this post, we'll explore these pitfalls and discuss best practices to avoid them.
Pitfall 1: Misusing Property Placeholders
One common mistake when using the @Value annotation is misusing property placeholders. Property placeholders allow developers to externalize configuration values into properties files. When using @Value, it's important to properly reference these property placeholders, as failing to do so can result in unexpected behavior.
@Value("${spring.datasource.url}")
private String databaseUrl;
In this example, the @Value annotation references the property placeholder spring.datasource.url defined in a properties file. It's crucial to ensure that the property placeholder is correctly referenced to avoid potential errors.
Pitfall 2: Missing Default Values
Another pitfall is not providing default values for the injected properties. When using @Value, it's important to consider scenarios where the property may not be defined, such as during development or testing. Failing to provide default values can lead to NullPointerExceptions or unexpected behavior in your application.
@Value("${app.mode:default}")
private String appMode;
In this example, default is provided as the default value for the app.mode property. This ensures that if the property is not defined, the application falls back to the default value.
Pitfall 3: Lack of Type Conversion
When using @Value, developers should be aware of the lack of implicit type conversion. While it's convenient to inject properties directly into fields, Spring does not perform type conversion for you. This can lead to type mismatch exceptions if the injected value cannot be converted to the field type.
@Value("${app.maxConnections}")
private int maxConnections;
In this example, if the property app.maxConnections is defined as a string in the properties file, attempting to inject it into an int field will result in a type mismatch exception. It's important to perform any necessary type conversion explicitly, such as by using Integer.parseInt().
Pitfall 4: Limited Support for Binding Collections
One limitation of the @Value annotation is its limited support for binding collections. While it's possible to inject comma-separated values into a List or Set, this approach has its limitations. For more complex configuration scenarios, developers may find that using the @ConfigurationProperties annotation provides better support for binding properties to collections.
@Value("${app.allowedOrigins}")
private List<String> allowedOrigins;
In this example, app.allowedOrigins is a comma-separated list of values defined in the properties file. While this approach works for simple cases, it may not suffice for more complex collection bindings.
Best Practices to Avoid Pitfalls
To mitigate the aforementioned pitfalls, consider the following best practices when using the @Value annotation in Spring:
- Properly document and organize property placeholders: Clearly document and organize your property placeholders to ensure they are correctly referenced.
- Provide meaningful default values: Always provide default values for injected properties to prevent unexpected behavior.
- Perform explicit type conversion: When injecting properties into fields, be mindful of type conversion and perform it explicitly as needed.
- Consider using @ConfigurationProperties for complex configurations: If you find yourself dealing with complex configurations or collections, consider using the @ConfigurationProperties annotation for better support.
By following these best practices, developers can effectively leverage the @Value annotation while avoiding common pitfalls in their Spring applications.
Final Considerations
The @Value annotation in Spring offers a convenient way to inject externalized configuration values into beans. However, it's important for developers to be aware of common pitfalls associated with its usage. By understanding these pitfalls and adopting best practices, developers can effectively utilize the @Value annotation in their Spring applications while writing robust and maintainable code.
To delve deeper into Spring annotations and best practices, check out the official Spring Documentation.
Checkout our other articles