Enhancing Java Development with New Features
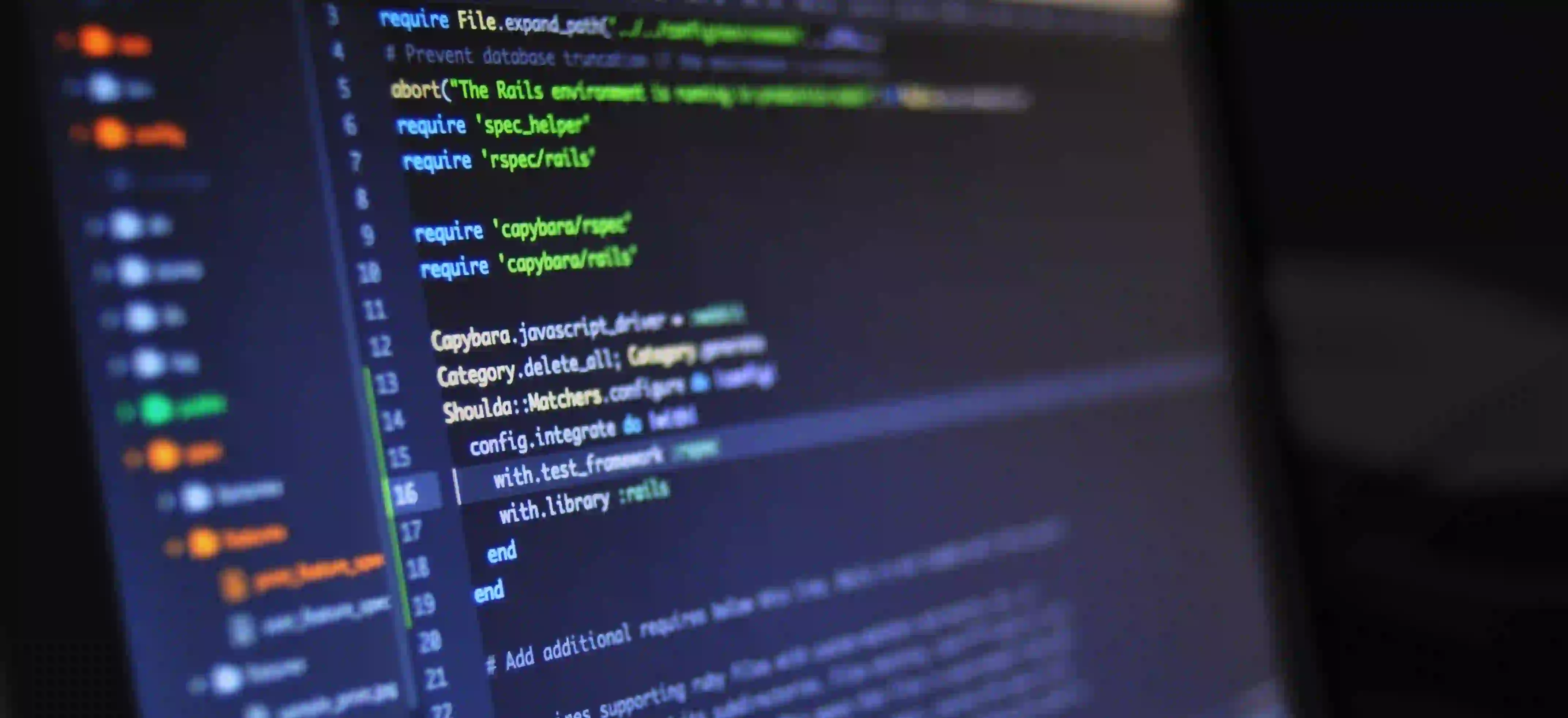
Embracing the Power of Java: New Features and Enhancements
Java, a versatile and robust programming language, has stood the test of time as a preferred choice for building enterprise-level applications. With its consistent evolution, Java has continuously introduced new features and enhancements, empowering developers to write efficient and maintainable code. In this article, we'll explore some of the latest features and enhancements in Java that significantly elevate the development experience.
1. Java Platform Module System (JPMS)
The Java Platform Module System, introduced in Java 9, revolutionized the way developers structure and maintain their code. By modularizing the JDK and enabling developers to create modular applications, JPMS facilitates better code organization, readability, and maintenance. With modules, developers can encapsulate code and specify explicit dependencies, thereby promoting better encapsulation and improving the overall scalability of applications.
// Example module declaration
module com.example.myapp {
requires transitive com.example.mylib;
exports com.example.myapp;
}
2. Local Variable Type Inference
Java 10 introduced the var
keyword, allowing developers to declare local variables with inferred types. This feature reduces boilerplate code and improves code readability without sacrificing the strong static typing that Java is known for.
// Before Java 10
Map<String, List<String>> map = new HashMap<>();
// With local variable type inference
var map = new HashMap<String, List<String>>();
3. Improved Garbage Collection
Java continuously enhances its Garbage Collection (GC) mechanism to efficiently manage memory. With features like G1GC (Garbage-First Garbage Collector) introduced in Java 9 and ongoing refinements in subsequent releases, Java offers better performance and allocation management, reducing latency and improving overall application responsiveness.
4. Reactive Programming with Java 9 Flow API
Java 9 introduced the Flow API, providing support for reactive streams. This enhancement enables developers to write asynchronous, non-blocking code that is more responsive and scalable, making it easier to build reactive applications with Java.
// Example of Publisher-Subscriber using Flow API
Publisher<Integer> publisher = ...
Subscriber<Integer> subscriber = ...
publisher.subscribe(subscriber);
5. Pattern Matching (Preview Feature in Java 12)
Java 12 introduced a preview feature for pattern matching, allowing developers to concisely and safely extract components from objects using patterns. This feature simplifies code, especially when working with complex data structures and nested objects.
// Example of pattern matching in Java
if (obj instanceof String s) {
System.out.println(s.toUpperCase());
}
6. Records (Preview Feature in Java 14)
Records, a preview feature in Java 14, provide a concise way to declare Plain Old Java Objects (POJOs) by reducing the ceremony of writing data-centric classes. Records offer automatic implementations of equals()
, hashCode()
, and toString()
, significantly improving code conciseness and readability.
// Example of a record in Java
public record Point(int x, int y) {}
7. Enhanced Switch Statement (Preview Feature in Java 12)
Java 12 introduced a preview feature to enhance the traditional switch statement. With features like "switch expressions" and "arrow case labels," the switch statement becomes more expressive and powerful, enabling developers to write more concise and readable code.
// Example of enhanced switch statement
int numLetters = switch (day) {
case "Monday", "Friday", "Sunday" -> 6;
case "Tuesday" -> 7;
default -> 5;
};
In Conclusion, Here is What Matters
Java's evolution through the introduction of new features and enhancements has significantly enriched the development experience. These additions have not only improved the language's expressiveness and readability but also bolstered its capabilities to build modern, scalable, and maintainable applications. As Java continues to advance, embracing these features will undoubtedly empower developers to elevate their coding prowess and deliver high-quality solutions.
Embrace the power of Java's new features and enhancements, and witness the transformation of your development journey. Happy coding!
Remember to subscribe to our newsletter for more insightful Java content.