Creating Eo Object Factories and Accessing Dictionaries
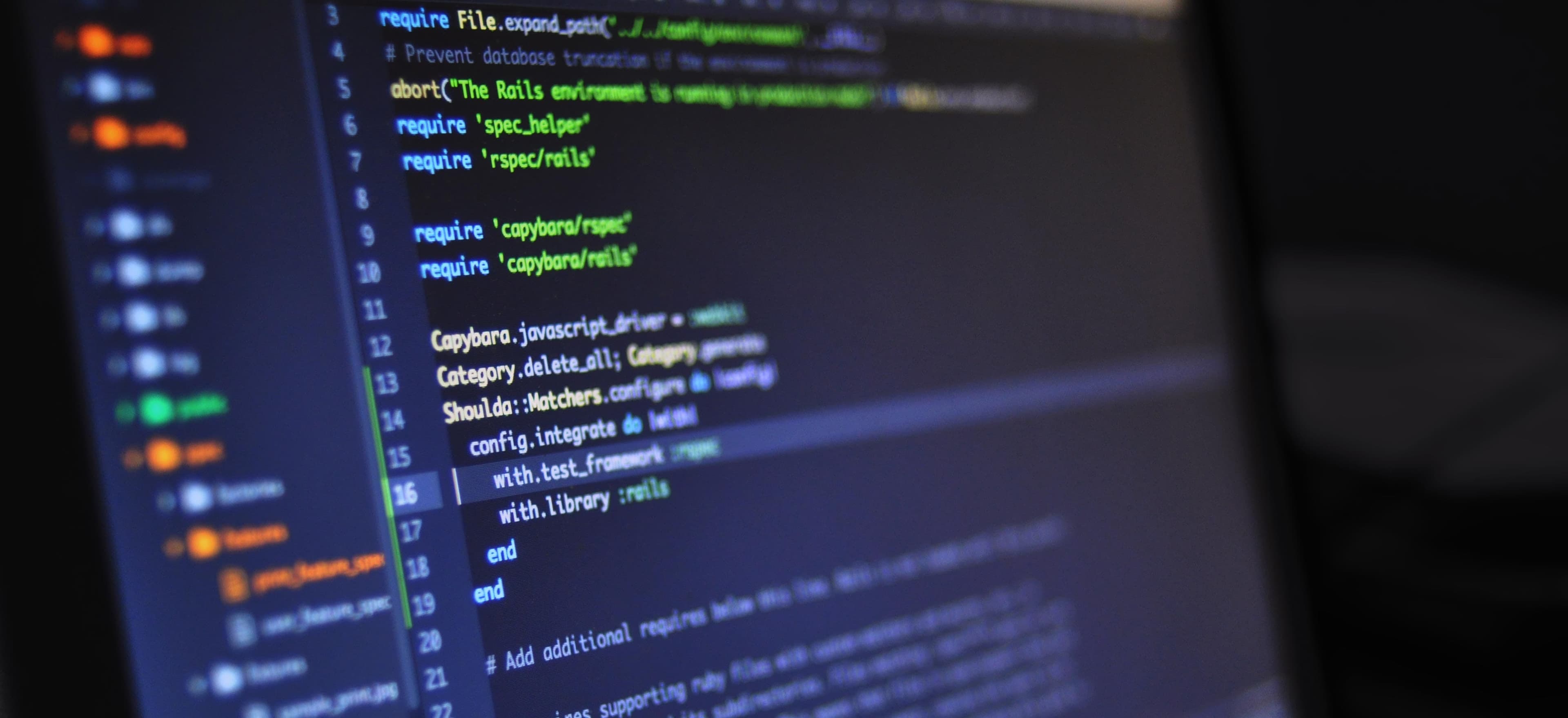
- Published on
Mastering Java: Creating Efficient Object Factories and Accessing Dictionaries
In the world of Java development, efficiency and flexibility are vital. When it comes to creating objects and accessing dictionaries, it's essential to implement best practices to optimize your code's performance. In this guide, we will explore how to create efficient object factories and efficiently access dictionaries in Java.
Creating Objects with Factories
Object creation in Java involves using constructors. However, as your codebase grows, managing object creation solely through constructors can become cumbersome. This is where object factories come into play. Object factories encapsulate the creation logic, providing a more flexible way to create objects.
The Factory Method Pattern
The factory method pattern is a creational pattern that uses factory methods to deal with the problem of creating objects without specifying the exact class of object that will be created. This pattern provides a way to delegate the object creation to subclasses.
Let's consider an example where we have a Shape
interface and its implementations Circle
and Square
. We can create a ShapeFactory
to encapsulate the object creation logic.
public interface Shape {
void draw();
}
public class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing Circle");
}
}
public class Square implements Shape {
@Override
public void draw() {
System.out.println("Drawing Square");
}
}
public class ShapeFactory {
public Shape createShape(String type) {
if ("circle".equalsIgnoreCase(type)) {
return new Circle();
} else if ("square".equalsIgnoreCase(type)) {
return new Square();
}
return null;
}
}
In the above example, ShapeFactory
encapsulates the creation logic and provides a way to create different shapes based on the input.
The Builder Pattern
Another approach to creating objects is the builder pattern. It is used to construct a complex object step by step. This pattern allows you to produce different types and representations of an object using the same construction code.
Let’s take an example of a Person
class with several optional parameters. Using the builder pattern, we can cleanly create a Person
object, specifying only the attributes we need.
public class Person {
private String firstName;
private String lastName;
private int age;
public Person(PersonBuilder builder) {
this.firstName = builder.firstName;
this.lastName = builder.lastName;
this.age = builder.age;
}
public static class PersonBuilder {
private String firstName;
private String lastName;
private int age;
public PersonBuilder(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public PersonBuilder setAge(int age) {
this.age = age;
return this;
}
public Person build() {
return new Person(this);
}
}
}
Using the builder pattern, we can create a Person
object as follows:
Person person = new Person.PersonBuilder("John", "Doe")
.setAge(30)
.build();
This ensures a clean and readable way to create objects, especially when dealing with numerous optional parameters.
Accessing Dictionaries in Java
Dictionaries, or maps, are essential data structures in Java that store key-value pairs. Efficient access and manipulation of dictionaries are crucial for maintaining high-performance applications.
Using HashMap for Key-Value Storage
The HashMap
class in Java provides the basic implementation of the Map interface. It stores data in key-value pairs and allows the use of null
for both keys and values.
Let's see an example of how to use HashMap
to store and access key-value pairs:
import java.util.HashMap;
import java.util.Map;
public class HashMapExample {
public static void main(String[] args) {
Map<String, String> fruits = new HashMap<>();
fruits.put("apple", "red");
fruits.put("banana", "yellow");
System.out.println(fruits.get("apple")); // Output: red
}
}
In the above example, we create a HashMap
to store fruit colors and then access the value for the key "apple".
Immutable Dictionaries with Collections.unmodifiableMap
In scenarios where you need to ensure that a dictionary remains unmodifiable, you can make use of Collections.unmodifiableMap
to create an immutable view of the original map. This is particularly useful when you want to prevent external code from modifying the contents of the dictionary.
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
public class ImmutableMapExample {
public static void main(String[] args) {
Map<String, String> originalMap = new HashMap<>();
originalMap.put("key1", "value1");
originalMap.put("key2", "value2");
Map<String, String> immutableMap = Collections.unmodifiableMap(originalMap);
// Attempt to modify the immutable map will result in UnsupportedOperationException
// immutableMap.put("key3", "value3");
}
}
In the example above, we create an immutable view of the originalMap
using Collections.unmodifiableMap
. Any attempt to modify the immutableMap
will result in UnsupportedOperationException
.
Concurrent Access with ConcurrentHashMap
In multi-threaded applications, it's crucial to use data structures that support concurrent access. The ConcurrentHashMap
class provides a way to achieve thread-safety for dictionary operations without the need for explicit synchronization.
Here's an example of using ConcurrentHashMap
for concurrent access:
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentHashMapExample {
public static void main(String[] args) {
Map<String, String> concurrentMap = new ConcurrentHashMap<>();
concurrentMap.put("key1", "value1");
concurrentMap.put("key2", "value2");
// Thread-safe update
concurrentMap.compute("key1", (key, value) -> value + "_updated");
}
}
In the above example, we use ConcurrentHashMap
to safely perform concurrent updates to the map.
Lessons Learned
Efficient object creation and dictionary access are essential aspects of Java development. By leveraging object factories and choosing the right dictionary implementation based on your requirements, you can ensure that your Java applications are both performant and maintainable.
In conclusion, mastering these techniques will enable you to write cleaner, more efficient code, paving the way for scalable and robust Java applications.
So, start integrating these best practices into your Java development process and witness the improvement in your code's performance and flexibility!
Checkout our other articles