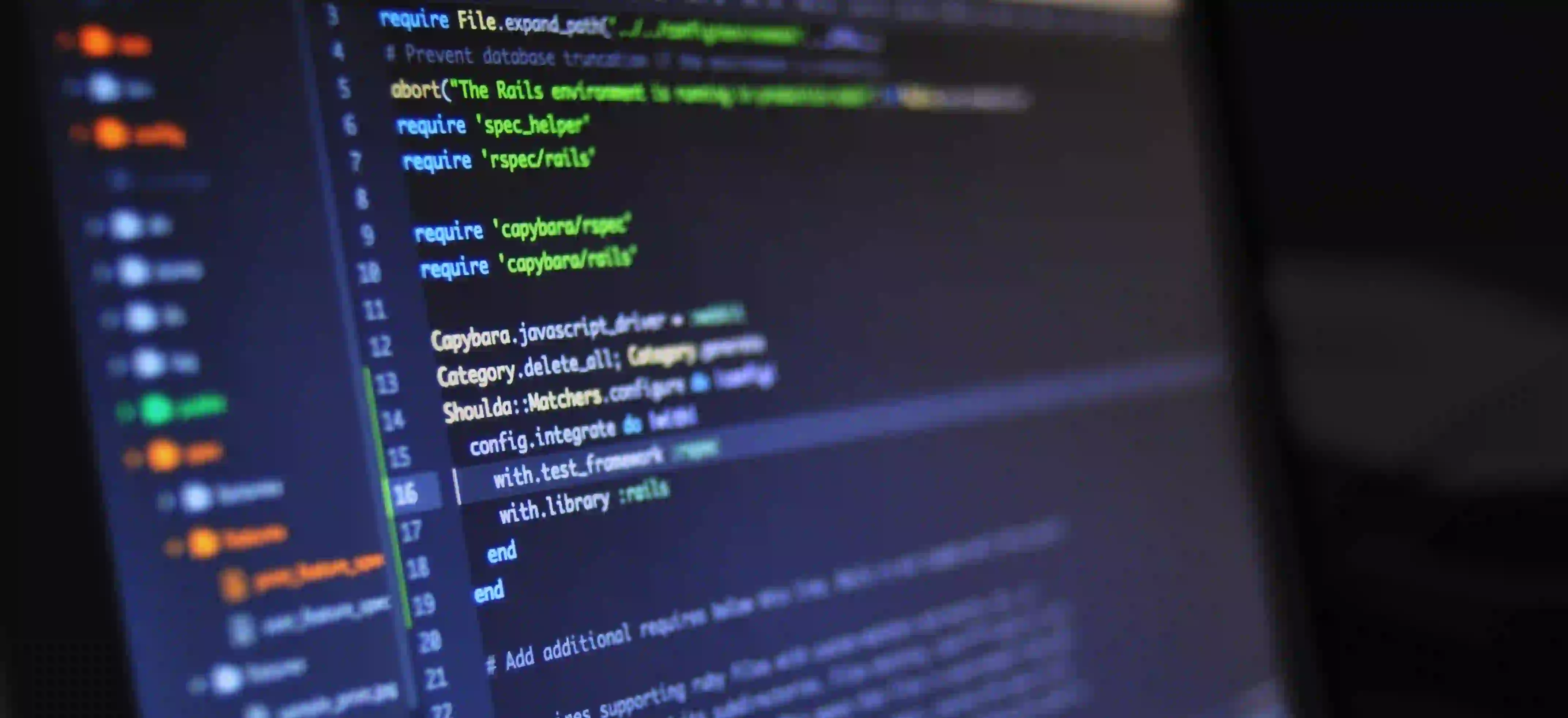
Building a Dynamic ListView with Multiple Row Layouts in Java
In Android development, a ListView is a fundamental UI component that displays a scrollable list of items. Oftentimes, you may need to create a ListView with multiple row layouts to accommodate different types of data. In this tutorial, we will explore how to achieve this in a seamless and efficient manner using Java.
Understanding the Use Case
Imagine an app that needs to display a list of products, where each product may have a different layout based on its type (e.g., electronics, clothing, accessories). Implementing multiple row layouts in a ListView allows for a visually appealing and organized representation of diverse data.
Setting Up the Project
Before diving into the code, make sure you have an Android project set up in Android Studio.
Creating the Layouts
First, let's create the layout files for the different row types that we want to display in the ListView. For example, we might have row_electronics.xml
, row_clothing.xml
, and row_accessories.xml
.
<!-- row_electronics.xml -->
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<!-- Add your electronic item layout components here -->
</RelativeLayout>
Repeat the above code for row_clothing.xml
and row_accessories.xml
, customizing the layout for each row type.
Creating the Custom Adapter
To handle the dynamic ListView with multiple row layouts, we need to create a custom adapter that inflates the appropriate layout for each row based on its data type. Below is an example of how this can be achieved.
public class CustomAdapter extends BaseAdapter {
private static final int TYPE_ELECTRONICS = 0;
private static final int TYPE_CLOTHING = 1;
private static final int TYPE_ACCESSORIES = 2;
private List<Object> mData;
private LayoutInflater mInflater;
public CustomAdapter(Context context, List<Object> data) {
mData = data;
mInflater = LayoutInflater.from(context);
}
@Override
public int getItemViewType(int position) {
// Return the type of the item at a given position
// Implement your logic to determine the type based on the data
}
@Override
public int getViewTypeCount() {
// Return the total number of row layouts
return 3; // In this case, we have 3 types: electronics, clothing, accessories
}
@Override
public int getCount() {
return mData.size();
}
@Override
public Object getItem(int position) {
return mData.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
int type = getItemViewType(position);
if (convertView == null) {
switch (type) {
case TYPE_ELECTRONICS:
convertView = mInflater.inflate(R.layout.row_electronics, parent, false);
// Populate the electronic item layout with data
break;
case TYPE_CLOTHING:
convertView = mInflater.inflate(R.layout.row_clothing, parent, false);
// Populate the clothing item layout with data
break;
case TYPE_ACCESSORIES:
convertView = mInflater.inflate(R.layout.row_accessories, parent, false);
// Populate the accessories item layout with data
break;
}
}
return convertView;
}
}
In the CustomAdapter
class, we define the different row types using constants and override methods to handle the multiple row layouts. The getItemViewType(int position)
method should implement your logic for determining the type of each item based on the data, while getView(int position, View convertView, ViewGroup parent)
inflates the appropriate layout for each row.
Implementing the Dynamic ListView
Now that we have our custom adapter ready, let's use it to populate the ListView in our activity or fragment.
public class DynamicListActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_dynamic_list);
List<Object> dataList = // Populate the list with your data
ListView listView = findViewById(R.id.listView);
CustomAdapter adapter = new CustomAdapter(this, dataList);
listView.setAdapter(adapter);
}
}
In the above code, we initialize the ListView and set its adapter to our custom adapter CustomAdapter
. This enables the ListView to dynamically display the multiple row layouts based on the provided data.
In Conclusion, Here is What Matters
In conclusion, implementing a dynamic ListView with multiple row layouts in Android using Java involves creating custom layouts for each row type, developing a custom adapter to handle the different row types, and populating the ListView with the adapter. This approach allows for a flexible and organized representation of diverse data in your Android app.
By following the steps outlined in this tutorial, you can seamlessly build a dynamic ListView with multiple row layouts to meet the specific UI requirements of your application.
Start experimenting with dynamic ListView layouts today and enhance the visual appeal of your Android apps!
For additional information on custom adapters and ListView management, refer to the Android Developer Documentation.
Happy coding!