Mastering Java: Tackling Memory Management Issues
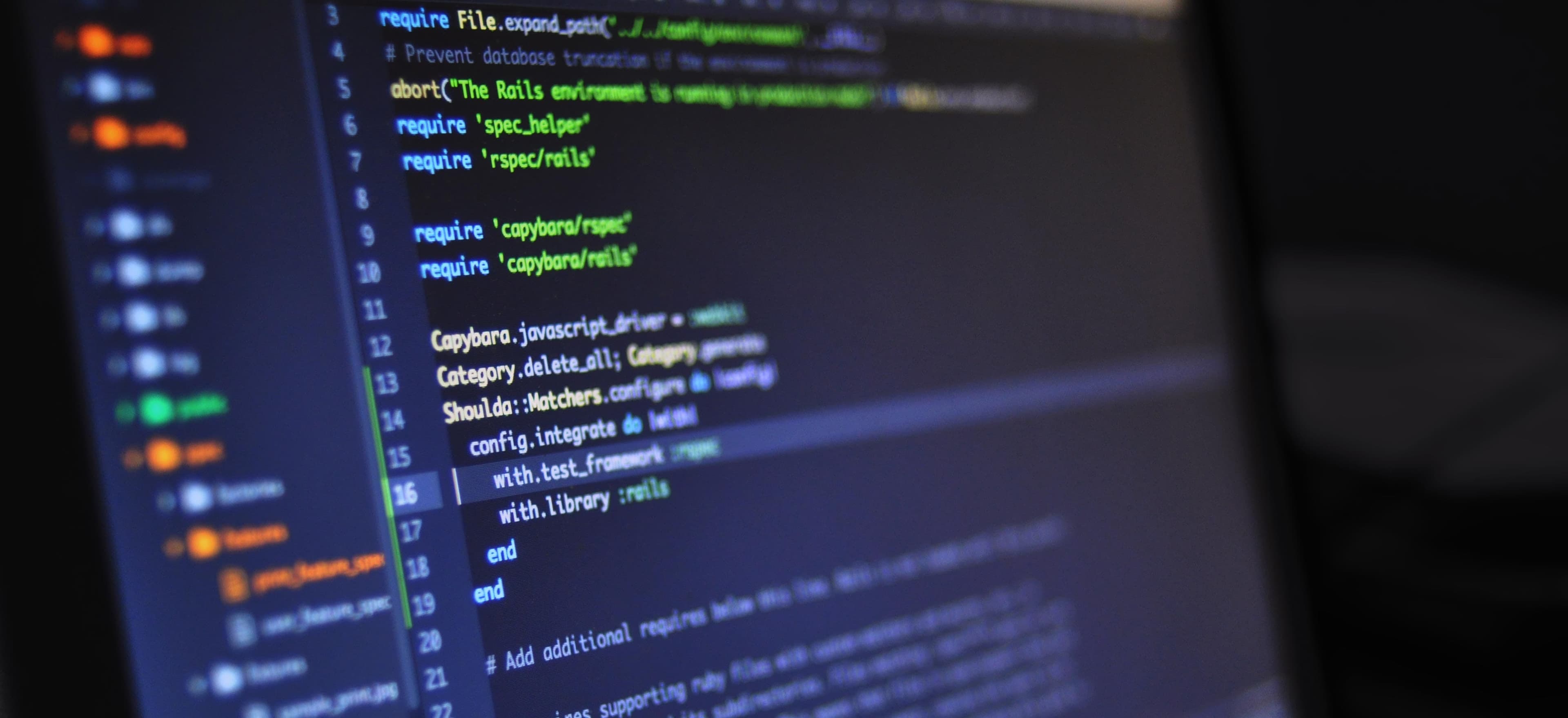
- Published on
Mastering Java: Tackling Memory Management Issues
When it comes to building robust and efficient Java applications, mastering memory management is crucial. Java, being a high-level language with automatic memory management, allows developers to focus on writing code without worrying about memory allocation and deallocation. However, this convenience comes with its own set of challenges. In this blog post, we will delve into memory management in Java, explore common memory-related issues, and discuss best practices to tackle them.
Understanding Java Memory Management
In Java, memory management revolves around the concepts of the stack and the heap. The stack is where method invocations and local variables are stored, while the heap is where objects and their instance variables reside.
The Stack
The stack in Java is a LIFO (Last-In, First-Out) data structure that stores method invocations and local variables. When a method is invoked, a new stack frame is pushed onto the stack. This stack frame contains the method's parameters, local variables, and the return address. Once the method execution is complete, the stack frame is popped off the stack.
The Heap
In Java, objects and their instance variables are allocated memory on the heap. The heap is a more dynamic area of memory and is managed by the garbage collector. When an object is no longer referenced, the garbage collector deallocates the memory occupied by that object, freeing up resources for reuse.
Common Memory Management Issues
Despite the automatic memory management in Java, several common issues can still arise, impacting the performance and stability of Java applications.
Memory Leaks
Memory leaks occur when objects are no longer in use but are still referenced, preventing the garbage collector from reclaiming their memory. This can lead to a gradual increase in memory consumption, eventually causing an OutOfMemoryError.
Garbage Collection Overhead
While the garbage collector automates memory deallocation, inefficient memory management can lead to increased garbage collection overhead. Frequent garbage collection cycles can result in degraded application performance as the system spends more time collecting and compacting memory.
Best Practices for Effective Memory Management
To address these memory management issues and ensure optimal application performance, Java developers can follow best practices focused on efficient memory allocation and deallocation, minimizing memory leaks, and reducing garbage collection overhead.
1. Use Proper Data Structures
Choosing the right data structures can have a significant impact on memory management. For example, using ArrayList
where a LinkedList
would be more appropriate can lead to unnecessary memory overhead. Understanding the differences between data structures and their memory implications is crucial for efficient memory management.
// Good practice: Using LinkedList for frequent insertions and deletions
List<String> linkedList = new LinkedList<>();
// Avoid: Using ArrayList for frequent insertions and deletions
List<String> arrayList = new ArrayList<>();
2. Manage Object References
Carefully managing object references is essential to prevent memory leaks. Nullifying object references when they are no longer needed can allow the garbage collector to reclaim memory efficiently.
// Good practice: Nullifying object reference when no longer needed
SomeObject obj = new SomeObject();
// ...
obj = null; // All references to obj are removed
3. Limit Object Creation
Frequent object creation can lead to increased memory consumption and more frequent garbage collection cycles. Reusing objects where possible can help reduce memory overhead.
// Good practice: Reusing StringBuilder instance
StringBuilder sb = new StringBuilder();
sb.append("Hello");
sb.append("World");
String result = sb.toString();
4. Tune Garbage Collection
Understanding and tuning the garbage collection process can significantly impact memory management. Configuring garbage collection parameters, such as heap size, collection algorithms, and collection frequency, can help minimize garbage collection overhead.
5. Use Memory Profiling Tools
Utilizing memory profiling tools such as VisualVM, YourKit, or Java Mission Control can provide valuable insights into memory usage, allocation patterns, and potential memory leaks. These tools enable developers to identify and address memory management issues effectively.
Closing Remarks
Mastering memory management in Java is essential for building high-performance and scalable applications. By understanding the underlying memory management principles, recognizing common memory-related issues, and following best practices, developers can optimize memory usage, minimize memory leaks, and reduce garbage collection overhead. Effective memory management not only improves application performance but also contributes to a more stable and reliable software environment.
In conclusion, mastering memory management in Java requires a thorough understanding of the stack and heap, awareness of common memory-related issues, and the implementation of best practices to mitigate these challenges. By implementing proper data structures, managing object references, limiting object creation, tuning garbage collection, and utilizing memory profiling tools, developers can ensure efficient memory management and elevate the performance of their Java applications.
Learning to navigate and optimize memory management in Java is a crucial skill for any developer aiming to build high-quality and efficient software.
Remember, effective memory management is not just about writing code; it's about engineering robust and efficient solutions that can stand the test of time. So keep learning, exploring, and mastering the art of memory management in Java!