Streamlining User Entity Access Control with Automation
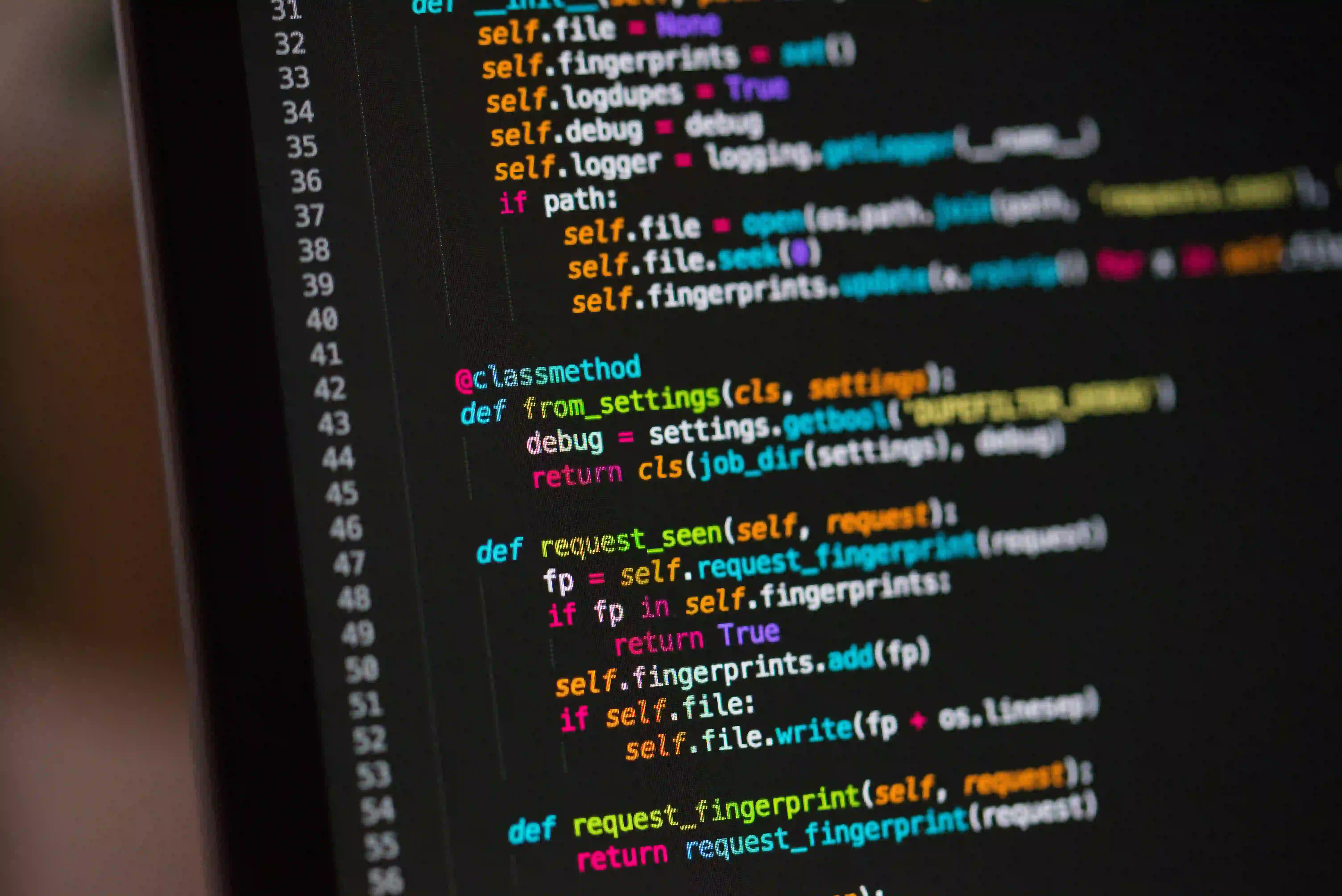
Streamlining User Entity Access Control with Automation
In today's digital landscape, where data privacy and security are of paramount importance, managing user entity access control is a critical aspect of application development. While Java provides robust security features, manual management of user entity access control can be cumbersome and prone to human errors. This is where automation comes into play. By automating user entity access control, developers can ensure a more efficient and secure system.
In this article, we will explore how to streamline user entity access control using automation in Java, leveraging the power of tools such as Spring Security and Apache Shiro.
Understanding User Entity Access Control
Before diving into automation, let's first grasp the concept of user entity access control. In a typical application, users are granted access to various resources based on their roles and permissions. This includes restricting access to certain endpoints, data, or functionalities within the application. Managing these access controls manually can be complex and error-prone, especially as the application grows in size and complexity.
Leveraging Spring Security for User Entity Access Control
One of the most widely used frameworks for implementing security features in Java applications is Spring Security. Spring Security provides comprehensive security services for Java EE-based enterprise software applications. It offers built-in support for user authentication, authorization, and protection against common security exploits.
Configuring Role-Based Access Control
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth
.inMemoryAuthentication()
.withUser("user").password(passwordEncoder().encode("password")).roles("USER")
.and()
.withUser("admin").password(passwordEncoder().encode("password")).roles("ADMIN");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
In the above Spring Security configuration, we define role-based access control rules using antMatchers
to restrict access to specific URLs based on the user's role. We also configure in-memory authentication with predefined user roles using configureGlobal
.
This approach simplifies user entity access control by clearly defining access rules based on user roles, making it easier to manage and maintain as the application scales.
Simplifying Authorization with Apache Shiro
Another valuable tool for implementing user entity access control in Java applications is Apache Shiro. Apache Shiro is a powerful and easy-to-use Java security framework that performs authentication, authorization, cryptography, and session management.
Defining Permissions in Shiro
public class UserEntityAccessControl {
public void checkPermission(User user, String entity, String permission) throws UnauthorizedAccessException {
Subject subject = SecurityUtils.getSubject();
if (!subject.isPermitted(entity + ":" + permission)) {
throw new UnauthorizedAccessException("User " + user.getUsername() + " does not have permission to " + permission + " " + entity);
}
}
}
In the above code snippet, we define a UserEntityAccessControl
class that uses Apache Shiro's Subject
to check if a user has the required permission for a specific entity. This approach centralizes the permission logic, making it easier to manage and modify access control rules.
Automating User Entity Access Control
While the manual configuration of access control rules works well for smaller applications, maintaining and updating these rules as the application grows can become a significant overhead. Automation plays a crucial role in simplifying this process.
Dynamic Permission Management
One way to automate user entity access control is by implementing dynamic permission management. This involves storing access control rules in a database or external configuration, allowing administrators to modify user permissions without having to modify the application code.
public class PermissionService {
public List<String> getUserPermissions(User user) {
// Logic to fetch user permissions from database or external configuration
}
}
By fetching user permissions from a centralized data source, such as a database, and dynamically updating them based on administrative actions, the system becomes more scalable and adaptable to changing access control requirements.
Key Takeaways
In conclusion, implementing user entity access control is a crucial aspect of application security, and automating this process can greatly enhance the efficiency and scalability of the system. By leveraging tools such as Spring Security and Apache Shiro, developers can simplify the management of access control rules and ensure a more secure application.
Automation not only reduces the likelihood of human errors but also enables dynamic and scalable permission management, making it easier to adapt to evolving security requirements.
By harnessing the power of automation, developers can streamline user entity access control, ultimately contributing to a more robust and secure application environment.
In the ever-evolving landscape of cybersecurity, the need for efficient and reliable user entity access control has never been more critical. With the right tools and strategies in place, developers can fortify their applications against potential security threats, while also optimizing the management of access control rules.