Improving Product Search Clicks Collection
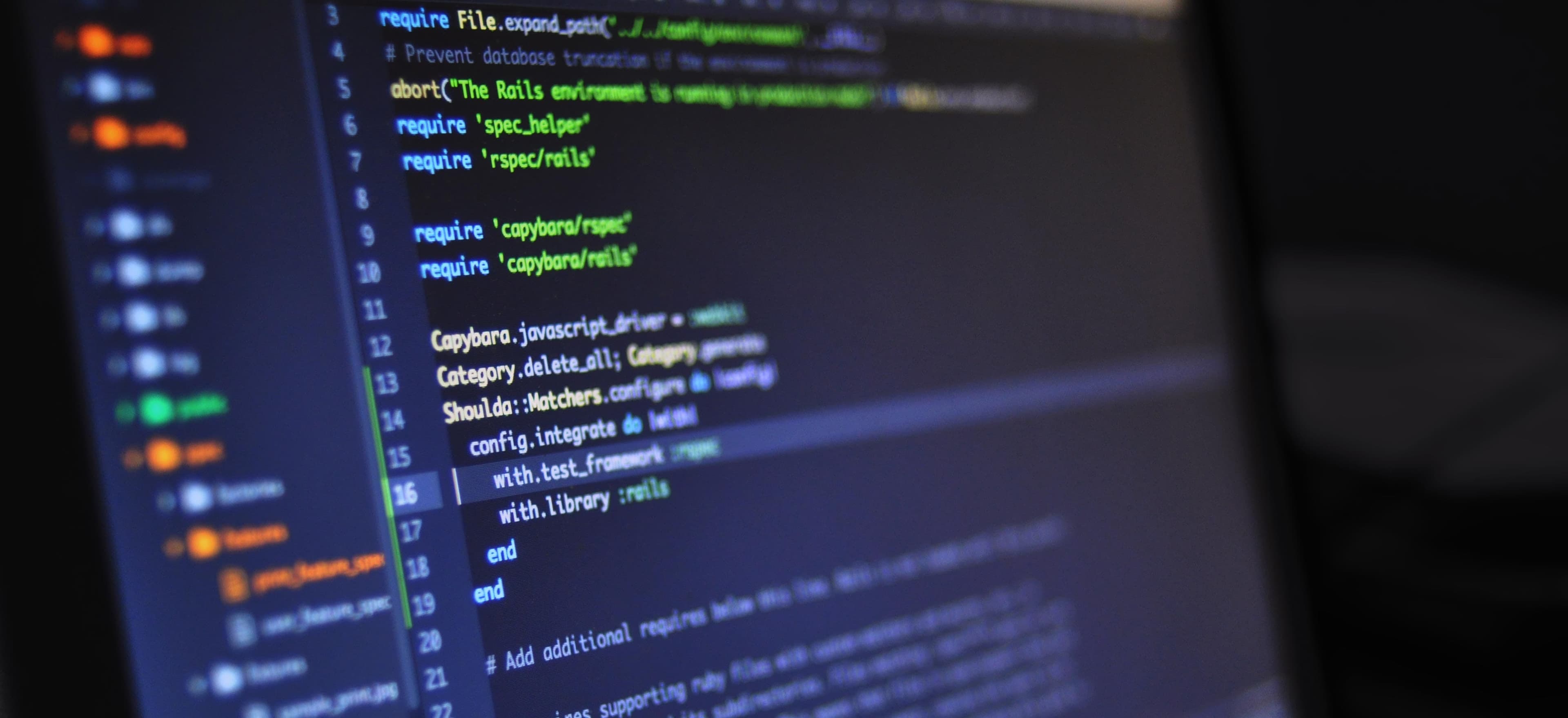
- Published on
Boosting Product Search Clicks Collection in Java: A Comprehensive Guide
In the realm of e-commerce, efficient search functionalities are pivotal in providing users with a seamless experience. In Java applications, optimizing product search clicks collection can significantly enhance the overall user experience. This guide delves into strategies for improving product search clicks collection in Java, embracing best practices rooted in performance, scalability, and maintainability.
Identifying the Need for Enhancing Product Search Clicks Collection
Effective collection and analysis of product search clicks offer insights that can fuel enhancements in search relevance, user experience, and marketing strategies. Capturing user interactions, such as clicks on search results, empowers businesses to iteratively refine the search algorithms and personalize the user experience. By improving the collection of product search clicks, businesses can glean actionable insights and drive informed decision-making processes.
Leveraging Java for Robust Clicks Collection
Java, renowned for its performance, scalability, and cross-platform capabilities, serves as an excellent framework for implementing a robust product search clicks collection system. Leveraging Java's extensive libraries, strong type system, and support for concurrent programming, engineers can build a high-performance, reliable clicks collection module.
Employing Data Structures: The Foundation of Efficient Clicks Collection
Within the domain of product search clicks collection, employing efficient data structures is critical for managing and analyzing user interactions. In Java, leveraging data structures such as HashMaps, ArrayLists, and LinkedLists can expedite the aggregation and processing of click data. Let's explore a snippet illustrating the use of HashMaps to store and retrieve click data efficiently:
// Creating a HashMap to store product search clicks
HashMap<String, Integer> clickMap = new HashMap<>();
// Storing click count for a specific product
String productId = "ABC123";
int currentClickCount = clickMap.getOrDefault(productId, 0);
clickMap.put(productId, currentClickCount + 1);
In this snippet, the HashMap efficiently maps product IDs to their respective click counts, facilitating quick retrieval and updates.
Asynchronous Data Collection for Enhanced Performance
In scenarios necessitating high throughput and responsiveness, asynchronous data collection mechanisms can augment the performance of product search clicks collection. Leveraging Java's CompletableFuture, engineers can orchestrate non-blocking, concurrent data collection processes. The code snippet below demonstrates the implementation of asynchronous click data collection using CompletableFuture:
// Asynchronously collect product search clicks
public CompletableFuture<Void> collectClick(String productId) {
return CompletableFuture.runAsync(() -> {
// Perform click data collection
// ...
});
}
// Invoking the async click collection
String productId = "XYZ789";
collectClick(productId);
By embracing asynchronous data collection, Java applications can sustain responsiveness while seamlessly capturing user interactions.
Ensuring Scalability through Distributed Data Collection
In scenarios demanding scalability and fault tolerance, distributed data collection mechanisms become imperative. Leveraging Apache Kafka, a distributed streaming platform, facilitates the seamless scaling and fault tolerance of product search clicks collection. The following code snippet exemplifies the integration of Apache Kafka for distributed click data collection in Java:
// Initializing Kafka producer
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
// Sending click data to Kafka topic
String topic = "clicksTopic";
String productId = "PQR456";
producer.send(new ProducerRecord<>(topic, productId, "click"));
// Closing the Kafka producer
producer.close();
By integrating Apache Kafka, Java applications can seamlessly handle the scaling and resiliency aspects of product search clicks collection.
Incorporating Automated Data Quality Checks
The accuracy and integrity of collected product search clicks are paramount. Java applications can integrate automated data quality checks to validate and cleanse incoming click data. Implementing data validation routines within the clicks collection module ensures that only accurate and consistent click data are stored and analyzed.
Embracing Observability for Insights and Debugging
Incorporating observability tools and techniques within the clicks collection module enables engineers to gain valuable insights into system behavior and performance. Integrating logging, metrics, and tracing mechanisms empowers developers to efficiently debug, optimize, and fine-tune the product search clicks collection system in Java.
Lessons Learned
Enhancing product search clicks collection in Java transcends mere data accumulation — it embodies the pursuit of actionable insights, user-centric improvements, and data-driven decision-making. By leveraging Java's robust features, asynchronous patterns, distributed systems, and observability tools, engineers can fortify product search clicks collection systems, fostering enhanced search relevance and user experiences.
In essence, the optimization of product search clicks collection in Java embodies a commitment to continual improvement, responsiveness, and scalability amid the dynamic e-commerce landscape.
Incorporating the mentioned concepts and methodologies can provide a potent foundation for engineers aiming to improve product search clicks collection in Java, ultimately enriching user experiences and propelling strategic business growth.
By embracing these strategies, Java applications can lay the groundwork for a resilient, responsive, and insightful product search clicks collection system, thereby fostering enhanced user experiences and informed decision-making processes.
To delve deeper into building robust Java applications and optimizing data collection, explore Java's official documentation and the comprehensive resources offered by Baeldung.
Checkout our other articles