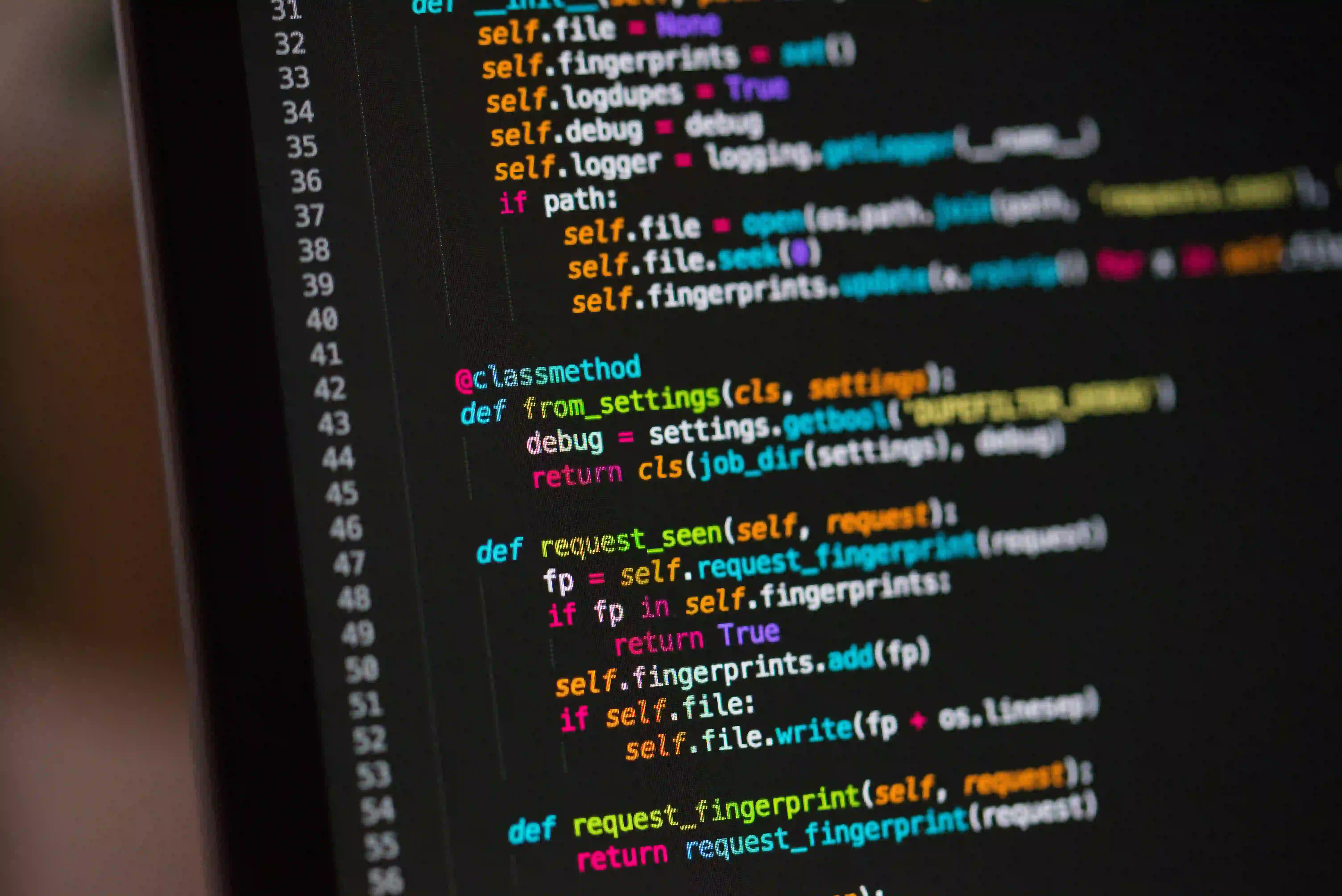
Secure Your Spring Boot Web App with Stormpath
In today's digital age, where cybersecurity is a constant concern, securing web applications is of utmost importance. When it comes to Java web development, Spring Boot has become one of the most popular frameworks. In this blog post, we will walk through the process of securing a Spring Boot web application using Stormpath, a user management and authentication service.
What is Stormpath?
Stormpath is a user management and authentication service that provides a robust set of features for securing web applications. It offers user authentication, authorization, user data storage, and social login integration, making it a comprehensive solution for securing your Spring Boot web application.
Prerequisites
To follow along with this tutorial, you should have:
- Basic knowledge of Spring Boot
- Java Development Kit (JDK) installed on your machine
- Maven or Gradle installed for dependency management
- An IDE such as IntelliJ IDEA or Eclipse
Setting Up the Project
To get started, let's create a new Spring Boot web application. You can use Spring Initializr to generate a new project with the necessary dependencies. For this tutorial, we will use Maven as the build tool.
Add the following dependencies to your pom.xml
file:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.stormpath.spring</groupId>
<artifactId>stormpath-spring-boot-starter</artifactId>
<version>2.1.1</version>
</dependency>
After adding the dependencies, you can create a simple Spring Boot application with a REST controller, such as:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HomeController {
@GetMapping("/")
public String home() {
return "Welcome to the secure home page!";
}
}
Configuring Stormpath
Next, we need to configure Stormpath in our application. Create a new file named application.properties
in the src/main/resources
directory and add the following configuration:
stormpath.client.cacheManagerEnabled=false
stormpath.web.login.enabled=true
stormpath.web.forgot.enabled=true
stormpath.web.change.enabled=true
stormpath.web.register.enabled=true
stormpath.web.idSite.enabled=true
stormpath.web.oauth2.enabled=true
This configuration sets up Stormpath for user authentication, registration, and other features within our Spring Boot application.
Integrating Stormpath for User Authentication
Now, let's integrate Stormpath for user authentication within our Spring Boot application. We can achieve this by creating a new controller to handle login requests.
import com.stormpath.sdk.account.Account;
import com.stormpath.sdk.servlet.account.AccountResolver;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletRequest;
@RestController
@RequestMapping("/auth")
public class AuthController {
@GetMapping("/login")
public String login(HttpServletRequest request) {
Account account = AccountResolver.INSTANCE.getAccount(request);
if (account != null) {
return "Logged in as: " + account.getFullName();
} else {
return "Please log in to access this page";
}
}
}
In this controller, we use Stormpath's AccountResolver
to authenticate the user and retrieve their account information. If the user is logged in, we display a welcome message with their full name; otherwise, we prompt them to log in.
Building and Running the Application
With the project set up and the integration with Stormpath completed, we can now build and run the Spring Boot application. Open a terminal at the project's root directory and execute the following command:
mvn spring-boot:run
Once the application is running, open a web browser and navigate to http://localhost:8080
. You should see the "Welcome to the secure home page!" message. To test the user authentication, navigate to http://localhost:8080/auth/login
, and you will be prompted to log in using Stormpath's authentication flow.
Key Takeaways
In this tutorial, we've covered the process of securing a Spring Boot web application using Stormpath for user authentication. By integrating Stormpath into your application, you can leverage its powerful features for user management, authentication, and social login integration, thereby enhancing the security and user experience of your web application.
By following the steps outlined in this tutorial, you've taken a proactive step towards strengthening the security of your Spring Boot web application. Embracing best practices for user authentication and integration of robust security solutions will undoubtedly fortify your application against potential cybersecurity threats.
Now that you have a foundational understanding of securing Spring Boot web applications with Stormpath, you can explore further customization and expand your knowledge of user management and authentication in Java web development.
In the increasingly complex landscape of cybersecurity, continuous learning and proactive security measures are crucial for safeguarding web applications and protecting user data. By remaining informed about the latest security trends and leveraging advanced tools and services like Stormpath, you can fortify your applications against evolving threats and build trust with your users.
Embark on your journey to bolstering the security of your Spring Boot web applications with Stormpath, and elevate your capabilities in delivering secure, user-centric web experiences.
So, why wait? Secure your Spring Boot web app with Stormpath and fortify your web application in just 15 minutes!