Naming Individual Test Cases in a Parameterized Test
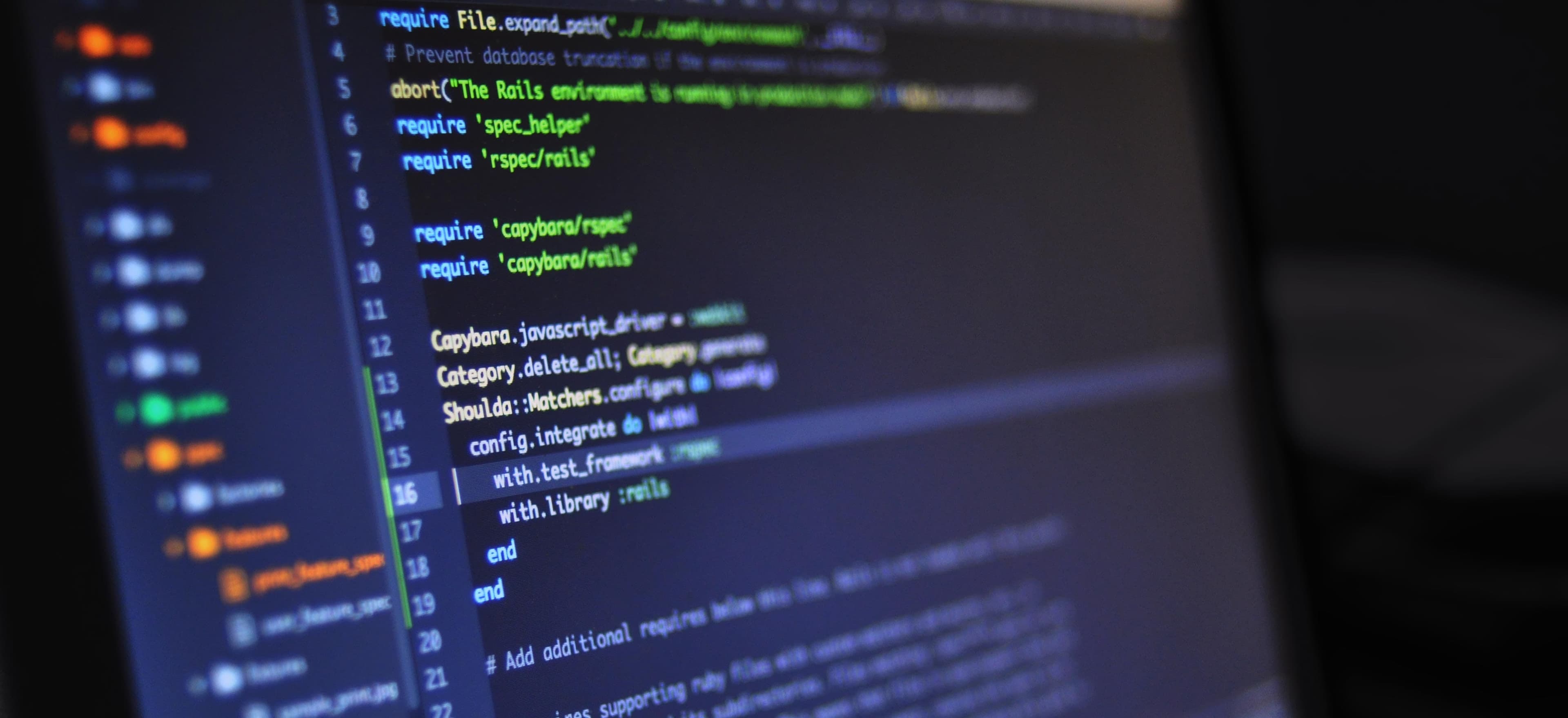
- Published on
Naming Individual Test Cases in a Parameterized Test
When writing parameterized tests in Java, it's crucial to create descriptive and meaningful names for individual test cases. This practice not only helps in clearly identifying the purpose of each test case but also aids in easier debugging and reporting.
Understanding Parameterized Tests
In Java, parameterized tests allow us to run the same test logic with different inputs. This is particularly useful when we want to test a piece of code with multiple sets of input data. By using parameterized tests, we can avoid duplicating test code while covering a variety of scenarios.
One of the most commonly used libraries for writing parameterized tests in Java is JUnit. JUnit 5, the latest version of the framework, provides robust support for parameterized tests through the use of @ParameterizedTest
and @CsvSource
(among other annotations).
Importance of Naming Test Cases
When working with parameterized tests, each set of input data corresponds to a distinct test case. Although JUnit assigns default names to parameterized test cases, these names may not be informative enough, especially when dealing with a large number of test cases. Assigning meaningful names to individual test cases makes it easier to identify the specific scenario being tested.
Best Practices for Naming Test Cases
1. Use Descriptive Names
Each test case name should clearly describe the input data or scenario being tested. Aim for names that provide meaningful context without the need to delve into the test code.
2. Include Pertinent Details
Ensure that the test case names include relevant details such as parameter values or specific conditions being tested. This helps in differentiating between various test cases and understanding their purpose at a glance.
3. Maintain Consistency
Follow a consistent naming convention across all test cases within a test class. Consistency promotes clarity and predictability, making it easier for developers to understand and maintain the test suite.
4. Keep it Concise
While it's important to be descriptive, avoid overly lengthy test case names. Concise names are easier to read and comprehend, especially when reviewing test reports or logs.
5. Avoid Ambiguity
Steer clear of generic or ambiguous test case names. The name should clearly convey the intent of the test without room for misinterpretation.
Example of Naming Individual Test Cases
Let's consider a simple parameterized test using JUnit 5 and the @CsvSource
annotation to test a hypothetical Calculator
class that performs addition. In this example, we'll demonstrate how to apply the best practices for naming individual test cases.
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.CsvSource;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorParameterizedTest {
@ParameterizedTest(name = "shouldAdd_{0}_and_{1}_to_return_{2}")
@CsvSource({
"2, 3, 5",
"5, 5, 10",
"0, 0, 0"
})
void testAddition(int num1, int num2, int expectedResult) {
Calculator calculator = new Calculator();
assertEquals(expectedResult, calculator.add(num1, num2));
}
}
In the above example, the @ParameterizedTest
annotation includes the name
attribute, which allows us to specify the naming pattern for the individual test cases. The placeholders {0}
, {1}
, and {2}
correspond to the values provided in the @CsvSource
.
By using this naming strategy, each test case will have a descriptive name that clearly indicates the input values and expected outcome. For instance, the test case for adding 2 and 3 will be named shouldAdd_2_and_3_to_return_5
.
Benefits of Well-Named Test Cases
Improved Readability
Descriptive test case names enhance the readability of test reports and make it easier for developers to comprehend the purpose of each test without delving into the test code.
Clear Identification of Failures
When a test case fails, a well-named test case provides immediate insight into the specific scenario that caused the failure, facilitating faster debugging and resolution.
Serves as Documentation
Meaningful test case names can serve as a form of documentation, especially for developers who may be unfamiliar with the test code or the functionality being tested.
Incorporating Naming Best Practices
By incorporating the best practices for naming individual test cases in parameterized tests, developers can significantly improve the maintainability, readability, and effectiveness of their test suites. Additionally, leveraging the built-in capabilities of testing frameworks like JUnit 5 allows for seamless implementation of these practices.
Remember, investing time in creating descriptive and meaningful test case names not only benefits the current development cycle but also contributes to the long-term maintainability and reliability of the codebase.
In conclusion, by adhering to the best practices outlined in this article, developers can harness the full potential of parameterized tests, ensuring clarity, efficiency, and robustness in their test suites.
For further understanding, you can delve deeper into parameterized tests by referencing the official JUnit 5 documentation and exploring advanced strategies for test case naming.