Java Program: Add Two Numbers Without Using Operator
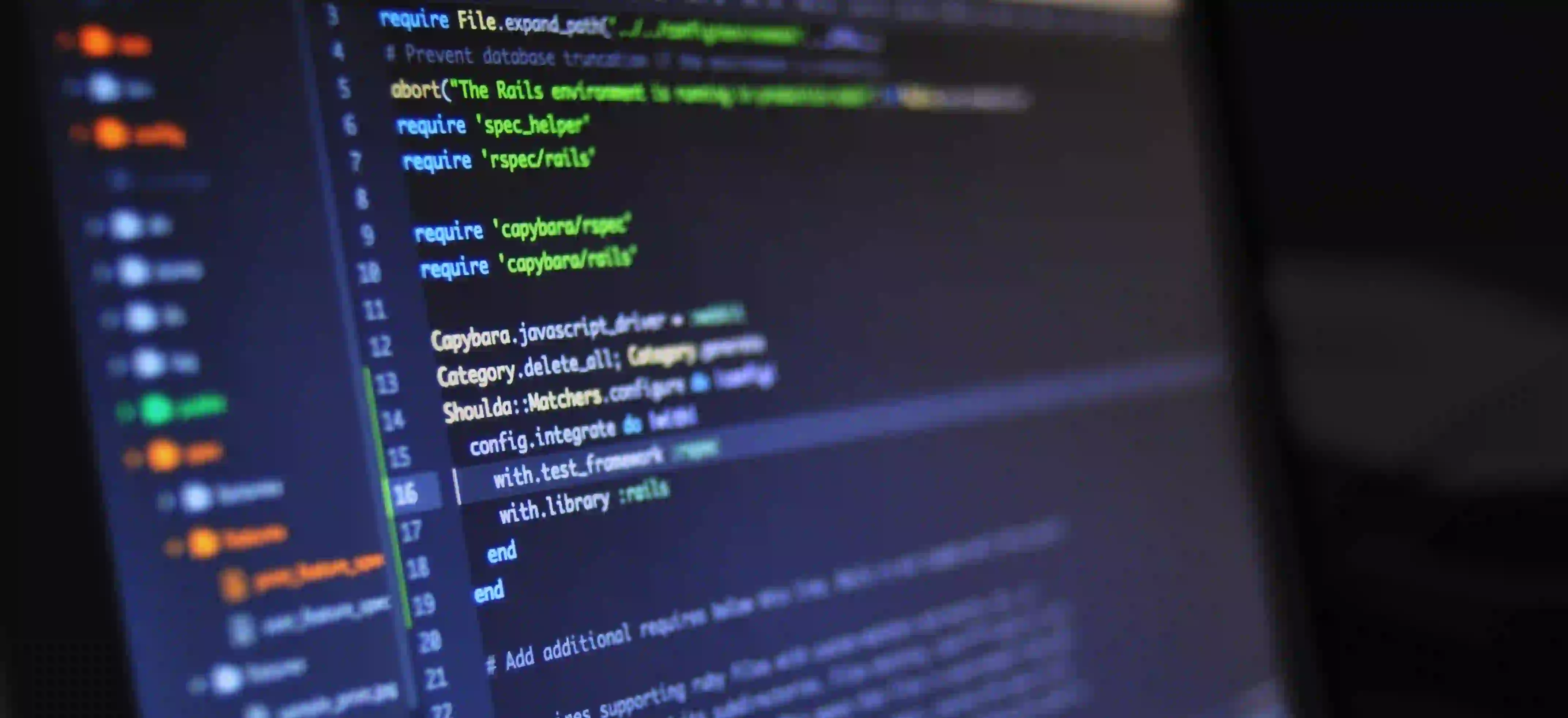
Adding Two Numbers without using an Operator in Java
In this blog post, we are going to explore an interesting Java program that adds two numbers without using the traditional +
operator. We will delve into the concept of bit manipulation, specifically the use of bitwise operators, to achieve this functionality.
Understanding Bitwise Operators
Bitwise operators in Java are used to perform manipulation of individual bits of binary numbers. The following are the bitwise operators used in Java:
&
(bitwise AND)|
(bitwise OR)^
(bitwise XOR)~
(bitwise complement)<<
(left shift)>>
(right shift)>>>
(zero fill right shift)
Adding Numbers Using Bitwise Operators
Let's consider the task of adding two numbers, a
and b
, without using the +
operator. We can achieve this by simulating the process of addition using bitwise operators.
Below is the Java program that accomplishes this:
public class AddWithoutOperator {
public static int add(int a, int b) {
while (b != 0) {
int carry = a & b;
a = a ^ b;
b = carry << 1;
}
return a;
}
public static void main(String[] args) {
int num1 = 25;
int num2 = 17;
System.out.println("Sum: " + add(num1, num2));
}
}
In this program, we define a method add
that takes two integers a
and b
as input and returns their sum. Inside the add
method, we use a while
loop to continue the addition process until there is no carry left.
Breaking Down the Code
Let's break down the code within the add
method to understand how it achieves the addition without using the +
operator:
-
Step 1:
carry = a & b;
- Here, we use the bitwise AND operator to calculate the bits that need to be carried over to the next position.
-
Step 2:
a = a ^ b;
- We use the bitwise XOR operator to perform the addition of
a
andb
without considering the carry.
- We use the bitwise XOR operator to perform the addition of
-
Step 3:
b = carry << 1;
- The carry obtained from the bitwise AND operation is left-shifted by 1 position and assigned to
b
, as it needs to be added to the next position.
- The carry obtained from the bitwise AND operation is left-shifted by 1 position and assigned to
By iterating through these steps, we simulate the process of addition without using the traditional +
operator.
Why Use Bitwise Operators for Addition?
You might be wondering, why go through the trouble of using bitwise operators for addition when the +
operator provides a simpler approach? While the +
operator is convenient, understanding bitwise operations and their applications is crucial for various programming scenarios, such as low-level system programming, cryptography, and optimization.
Additionally, in certain programming competitions and technical interviews, the ability to manipulate numbers at the bit level can be a valuable skill, showcasing a deep understanding of the underlying principles of computer arithmetic.
The Closing Argument
In this blog post, we explored the concept of adding two numbers without using the +
operator in Java. We harnessed the power of bitwise operators to achieve this functionality, providing insights into the application of bit manipulation in practical programming scenarios.
Understanding bitwise operations opens up a world of possibilities for solving complex problems and optimizing code for efficiency. While the example discussed here may seem simple, it serves as an introduction to the fascinating realm of bitwise manipulation in Java and beyond.
Now that you have a grasp of how to add numbers without using the traditional +
operator, consider exploring further applications of bitwise operators in your Java programming endeavors.
For further reading on bitwise operations in Java, consider referring to the Java Bitwise Operators documentation.
Happy coding!