Troubleshooting Maven Build Failures
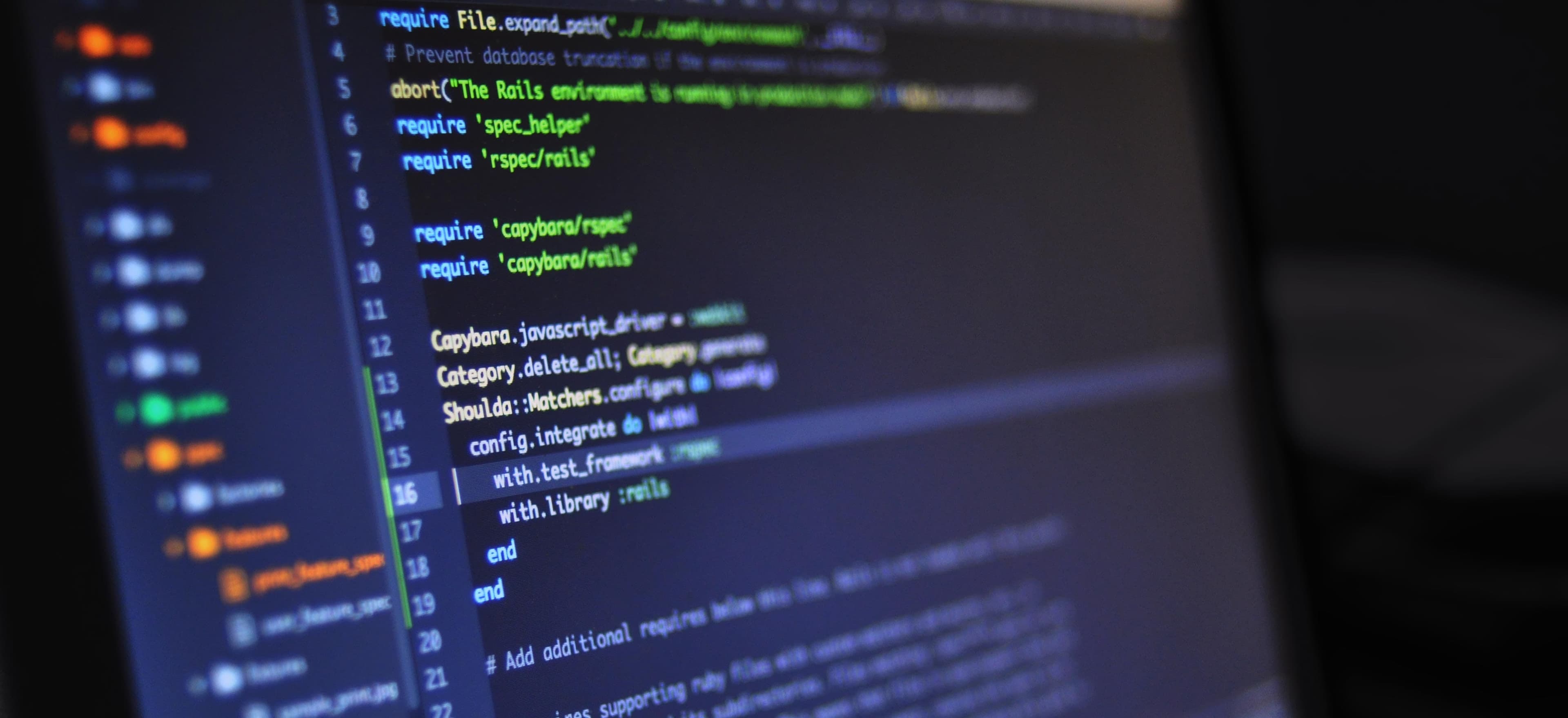
- Published on
Troubleshooting Maven Build Failures
Maven is a powerful build automation tool predominantly used for Java projects. Despite its efficiency, Maven build failures can be a frustrating hurdle in the development process. In this post, we will explore common Maven build failures and ways to troubleshoot them effectively.
1. Check for Internet Connectivity
Before delving into complex troubleshooting, ensure that your internet connection is stable. Maven downloads dependencies from remote repositories, and any network issues can lead to build failures. Once you've confirmed connectivity, proceed to the next steps.
2. Verify Maven and Java Installation
A frequent cause of build failures is an incorrect or incompatible installation of Maven and Java. Make sure you have the correct versions installed and that the environment variables are set up properly.
3. Review the POM file
The Project Object Model (POM) file serves as the backbone of a Maven project. It defines the project's configuration and dependencies. Incorrect or outdated POM configurations can lead to build failures. Verify the POM file for any discrepancies.
Example:
<dependencies>
<dependency>
<groupId>org.example</groupId>
<artifactId>sample-artifact</artifactId>
<version>1.0.0</version>
</dependency>
</dependencies>
In the example above, ensure that the artifact coordinates are correct and the versions are compatible with other dependencies.
4. Resolve Dependency Issues
Maven resolves dependencies transitively, meaning it downloads the project's dependencies and their respective dependencies. Conflicting versions of transitive dependencies often cause build failures. Use the mvn dependency:tree
command to analyze the dependency tree and identify conflicts.
5. Clean the Project
Build failures can sometimes result from a corrupted build. Cleaning the project with mvn clean
can resolve such issues by removing any existing build artifacts and starting afresh.
6. Update Maven Plugins
Outdated or incompatible Maven plugins can lead to failures. Update the plugins in the POM file to the latest versions and ensure compatibility with the current Maven version.
Example:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
</plugin>
</plugins>
</build>
7. Check for Compilation Errors
Compile errors are a common cause of build failures. Inspect the console output for any compilation errors and resolve them within the source code.
8. Analyze the Stack Trace
When a build failure occurs, Maven provides a detailed stack trace. Analyze this stack trace to identify the root cause of the failure, which can range from compilation errors to plugin issues.
9. Utilize Maven Debug Mode
Running Maven in debug mode (mvn -X
) provides detailed information about the build process, including plugin execution, dependency resolution, and more. This can aid in identifying the specific point of failure.
10. Seek Community Support
If you've exhausted all avenues and still can't resolve the build failure, don't hesitate to seek help from the Maven community. Platforms such as Stack Overflow and the Maven user mailing list are valuable resources for troubleshooting intricate build issues.
Wrapping Up
Maven build failures can stem from various sources, ranging from incorrect configurations to dependency conflicts. By systematically troubleshooting each potential cause, developers can effectively identify and resolve build failures, ensuring seamless project progression.
Remember, successful troubleshooting not only resolves the current issue but also enhances your understanding of Maven's intricacies, empowering you to tackle future challenges with confidence.
For more in-depth understanding, you can refer to the Maven Troubleshooting Guide and the official Maven Documentation.
Happy coding!