Addressing Technical Debt in AppSec: A Strategic Approach
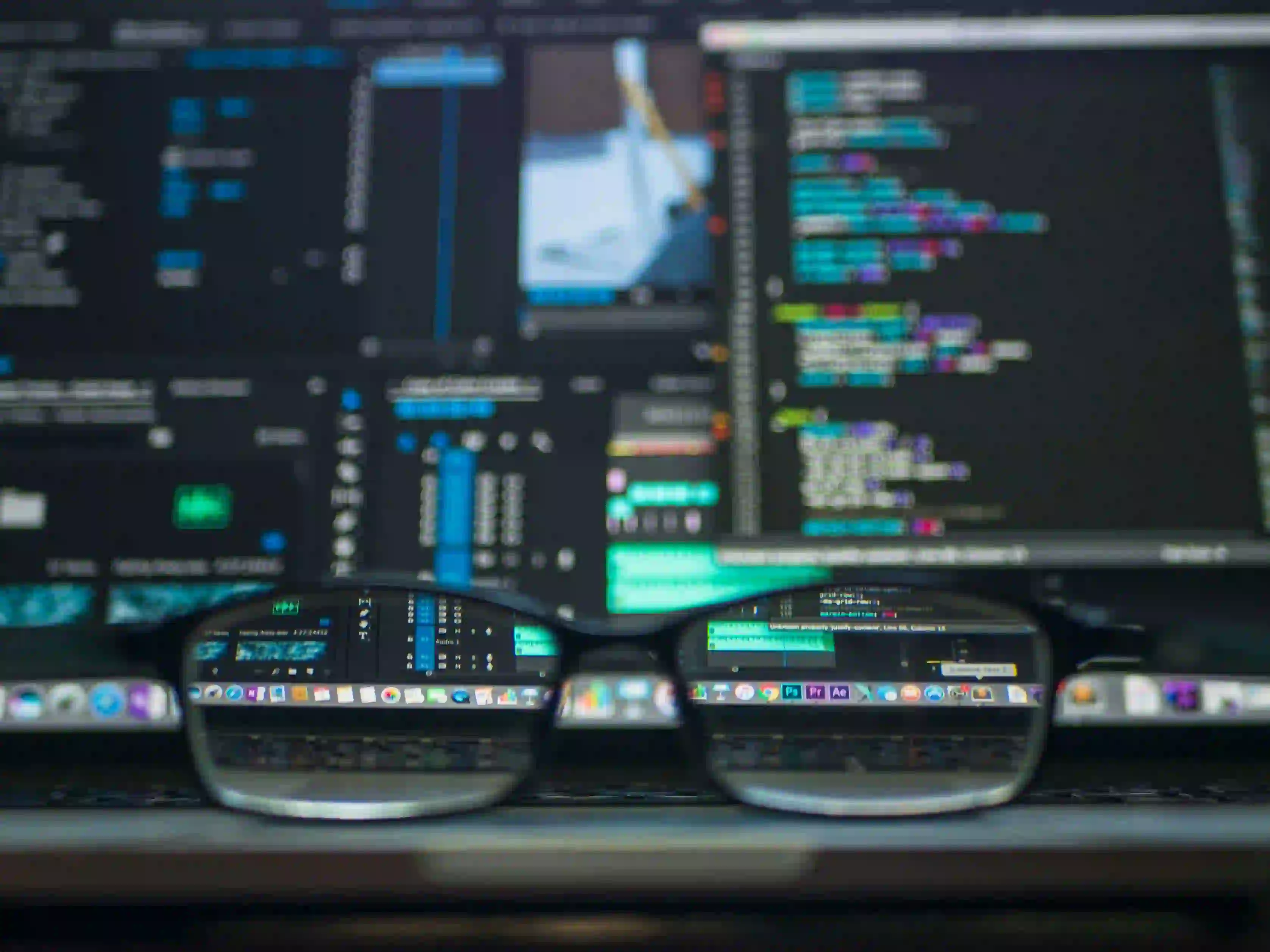
Addressing Technical Debt in AppSec: A Strategic Approach
Technical debt is a common issue in software development, and it also applies to application security (AppSec). Developers and security teams often have to balance between delivering new features and ensuring the security of the application. This delicate balance often leads to the accumulation of security-related technical debt, which can have severe consequences if not addressed promptly. In this article, we will discuss a strategic approach to addressing technical debt in AppSec, focusing on how Java developers can effectively tackle security-related technical debt in their applications.
Understanding Technical Debt in AppSec
Before diving into the strategies for addressing technical debt in AppSec, it's important to understand what technical debt in AppSec actually entails. Technical debt refers to the accumulated cost of additional rework caused by choosing an easy (and potentially insecure) solution now instead of using a better approach that would take longer. In the context of AppSec, technical debt can manifest as insecure coding practices, unpatched vulnerabilities, lack of input validation, inadequate authentication and authorization mechanisms, and more.
Implementing Secure Coding Practices in Java
Java is one of the most widely used programming languages for building enterprise-scale applications. When it comes to addressing technical debt in AppSec, implementing secure coding practices is paramount. By adhering to secure coding standards and best practices, developers can proactively mitigate security-related technical debt. Let's take a look at a few secure coding practices in Java:
Sanitizing User Input
public String sanitizeInput(String input) {
// Perform input validation and sanitization
// Return sanitized input
}
In the above code snippet, the sanitizeInput
method demonstrates the practice of input validation and sanitization. By validating and sanitizing user input, developers can prevent common vulnerabilities such as SQL injection and cross-site scripting (XSS) attacks.
Using Secure Communication
URL url = new URL("https://www.example.com");
HttpsURLConnection connection = (HttpsURLConnection) url.openConnection();
// Configure and make secure connection
Here, the code snippet illustrates how to establish a secure connection using HttpsURLConnection
in Java. Secure communication is essential for protecting sensitive data transmitted between the application and external systems.
Implementing Proper Error Handling
try {
// Code that may throw exceptions
} catch (IOException e) {
// Handle the exception gracefully
}
Proper error handling is crucial for identifying and mitigating potential security risks. By handling exceptions effectively, developers can prevent sensitive information leakage and ensure that the application remains robust in the face of unexpected errors.
Utilizing Automated Security Testing Tools
In the fast-paced world of software development, manual security testing is often not feasible for identifying and addressing security-related technical debt. This is where automated security testing tools come into play. By integrating automated security testing tools into the development pipeline, Java developers can proactively identify and fix security vulnerabilities before they escalate into technical debt. Tools such as OWASP ZAP and FindBugs Security Plugin provide automated scanning for common security issues, allowing developers to prioritize and address security-related technical debt efficiently.
Enforcing Secure Code Reviews
Code reviews are an integral part of the software development process, and when it comes to AppSec, they play a crucial role in addressing technical debt. By establishing a secure code review process, teams can ensure that security-related technical debt is identified and remediated early in the development lifecycle. During code reviews, developers can focus on reviewing the implementation of security controls, identifying insecure coding practices, and providing feedback on remediation strategies. This proactive approach significantly reduces the accumulation of security-related technical debt in Java applications.
Integrating Security Into the SDLC
The integration of security into the software development lifecycle (SDLC) is fundamental for addressing technical debt in AppSec. By including security activities such as threat modeling, security requirements analysis, and security testing in each phase of the SDLC, Java developers can proactively identify and mitigate security-related technical debt. This integrated approach ensures that security is not an afterthought but rather a fundamental aspect of the development process, leading to more secure and resilient applications.
A Final Look
In the realm of application security, addressing technical debt requires a strategic and proactive approach. Java developers can effectively tackle security-related technical debt by implementing secure coding practices, leveraging automated security testing tools, enforcing secure code reviews, and integrating security into the SDLC. By prioritizing security alongside feature development, teams can significantly reduce security-related technical debt and build more robust and secure Java applications.
Addressing technical debt in AppSec is an ongoing endeavor, and it requires a collective effort from developers, security teams, and organizational leadership. By adopting the strategies outlined in this article, Java developers can make significant strides in mitigating security-related technical debt and fortifying the security posture of their applications.
Remember, addressing technical debt in AppSec is not just about fixing existing issues; it's also about preventing them from occurring in the first place.
By investing in proactive security measures, Java developers can secure their applications while keeping pace with the demands of rapid software development.
Resources
- OWASP ZAP
- FindBugs Security Plugin