Performance Degradation in Spring Framework Usage
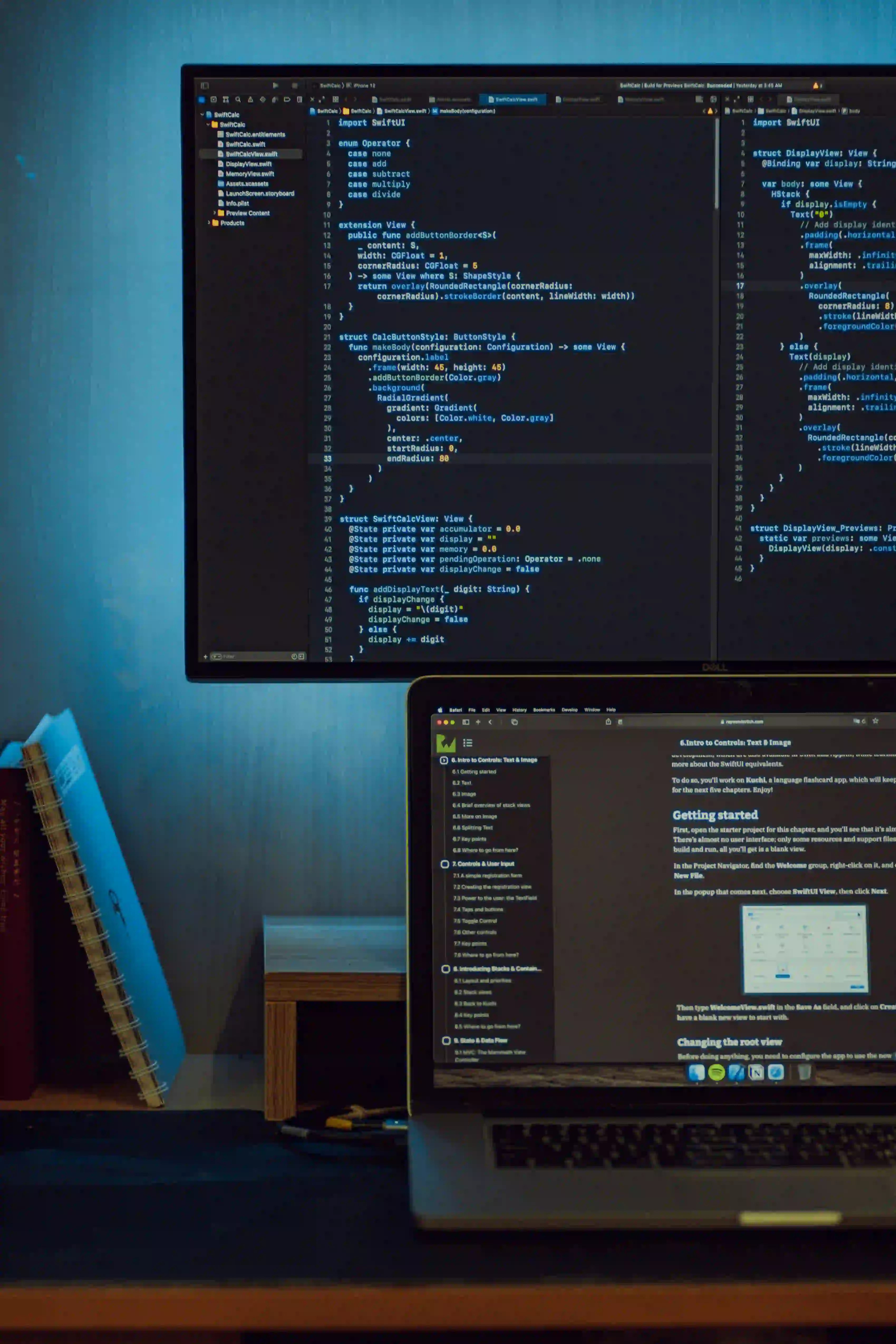
Understanding Performance Degradation in Spring Framework Usage
When developing Java applications, the Spring Framework has become a cornerstone for building robust, scalable, and maintainable systems. However, as with any powerful tool, the way it is used can impact the performance of the application. In this article, we'll delve into the common pitfalls that can lead to performance degradation in Spring Framework usage and discuss best practices to mitigate these issues.
1. Overusing Dependency Injection
The Problem:
While dependency injection is a core feature of the Spring Framework, over-reliance on constructor or setter injection can lead to performance issues. Each injection point introduces overhead, and an excessive number of dependencies can significantly impact the application's startup time.
The Solution:
Be mindful of the number of dependencies being injected and consider using @Autowired
sparingly. Utilize the @PostConstruct
annotation for expensive initialization routines that can be deferred until the application context is fully established.
Example:
@Component
public class MyService {
private final Dependency1 dependency1;
private final Dependency2 dependency2;
@Autowired
public MyService(Dependency1 dependency1, Dependency2 dependency2) {
this.dependency1 = dependency1;
this.dependency2 = dependency2;
}
@PostConstruct
public void initialize() {
// Expensive initialization code
}
}
2. Inefficient Database Access
The Problem:
Inadequate management of database interactions, such as excessive use of object-relational mapping (ORM) or inefficient query construction, can significantly impact the application's performance, leading to increased response times and decreased throughput.
The Solution:
Utilize database connection pooling to minimize the overhead of establishing new connections. Optimize database queries to fetch only the required data and consider employing caching strategies for frequently accessed information.
Example:
@Service
public class ProductService {
@Autowired
private ProductRepository productRepository;
public Product getProductByName(String name) {
return productRepository.findByName(name);
// Consider adding caching for improved performance
}
}
3. Improper Resource Management
The Problem:
Failing to release resources properly, such as open file handles, network connections, or database connections, can lead to resource exhaustion and performance degradation over time.
The Solution:
Utilize try-with-resources statements or the @PreDestroy
annotation to ensure proper release of resources such as file handles, database connections, and network sockets.
Example:
@Component
public class MyResourceProcessor {
@Value("${file.path}")
private String filePath;
public void processResource() {
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
// Process the resource
} catch (IOException e) {
// Handle the exception
}
}
@PreDestroy
public void cleanUp() {
// Perform cleanup operations, release connections, etc.
}
}
4. Inefficient Exception Handling
The Problem:
Inefficient exception handling can introduce unnecessary overhead, especially when exceptions are used for control flow or are overly verbose, leading to degraded performance.
The Solution:
Adopt a streamlined approach to exception handling, focusing on meaningful, targeted exception handling rather than excessive verbosity. Leverage Spring's exception handling mechanisms, such as @ControllerAdvice
, to centralize exception handling logic.
Example:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(DatabaseException.class)
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERROR)
public ResponseEntity<String> handleDatabaseException(DatabaseException ex) {
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body("An error occurred while processing the request");
}
}
5. Suboptimal Caching Strategies
The Problem:
Inadequate caching strategies or the absence of caching mechanisms can result in increased response times and decreased scalability, especially when dealing with frequently accessed or computationally expensive data.
The Solution:
Implement caching at various levels, including method-level caching using @Cacheable
and result-set caching for database queries. Utilize an appropriate caching provider, such as Ehcache or Redis, depending on the requirements of the application.
Example:
@Service
public class ProductService {
@Autowired
private ProductRepository productRepository;
@Cacheable("products")
public Product getProductById(Long id) {
return productRepository.findById(id).orElse(null);
}
}
A Final Look
The Spring Framework offers a wealth of features and functionalities that contribute to the rapid development of enterprise-grade applications. However, ensuring optimal performance requires a keen understanding of potential areas of degradation and the adoption of best practices to mitigate them.
By being mindful of dependency injection, database interactions, resource management, exception handling, and caching strategies, developers can harness the full power of the Spring Framework while maintaining stellar performance.
Keep in mind that profiling and benchmarking your application are essential steps in identifying performance bottlenecks and validating the effectiveness of performance optimization efforts.
In conclusion, a well-architected and efficiently implemented Spring application not only delivers high performance but also sets the stage for scalable and maintainable systems.
Now that you have a better understanding of potential performance degradation in Spring Framework usage, it's time to apply these best practices to your own projects and ensure that your applications perform at their best.
Remember, optimizing performance is an ongoing process, and staying informed about the latest developments and best practices is crucial in the ever-evolving landscape of software development.