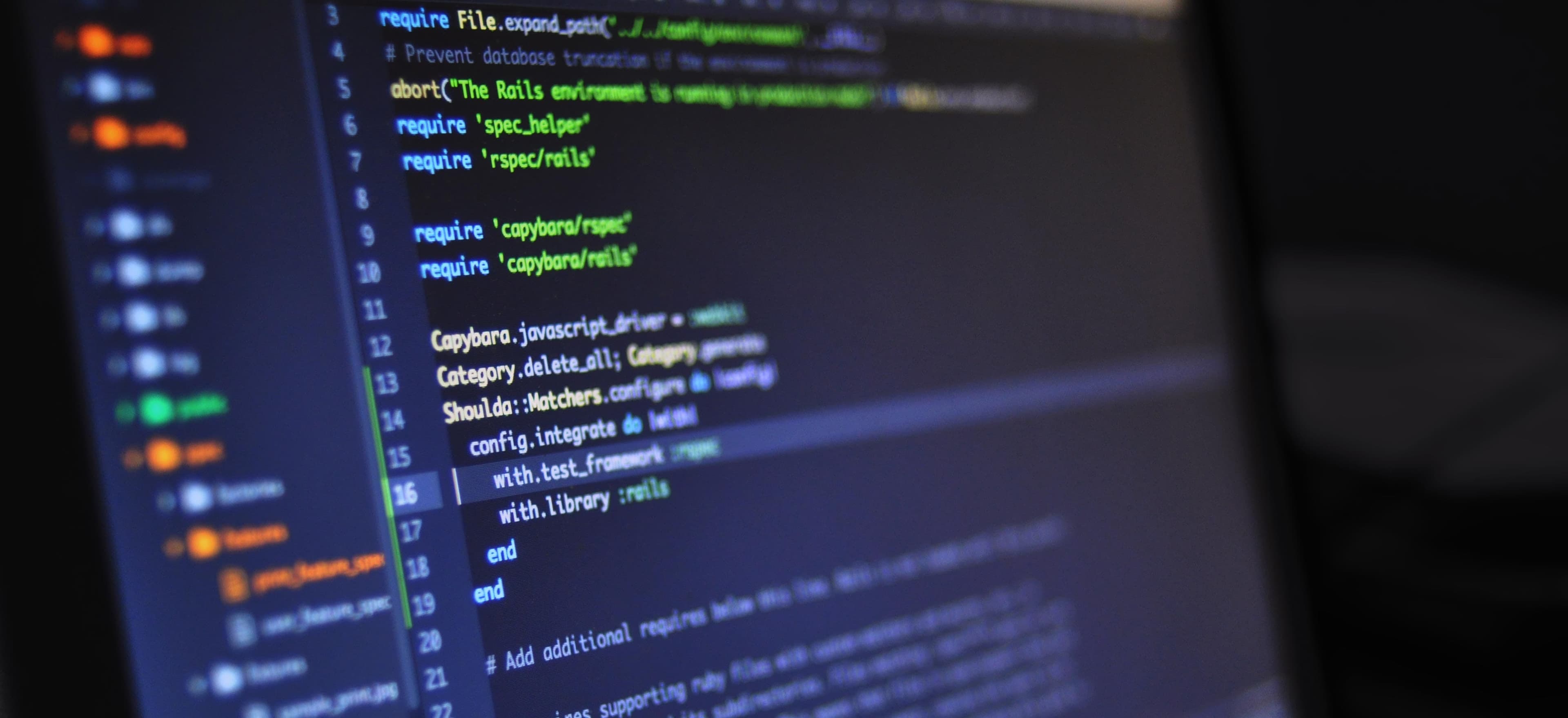
- Published on
Getting Started
Cross-Site Request Forgery (CSRF) is a type of security vulnerability that allows an attacker to make unauthorized transactions on behalf of a user. As a Java developer, it's crucial to secure your web applications from CSRF attacks to protect sensitive user data and prevent unauthorized actions.
In this blog post, we will discuss what CSRF attacks are, how they work, and most importantly, how to defend your Java web application against them using best practices and tools.
Understanding CSRF Attacks
CSRF attacks occur when an attacker tricks a user's browser into making an unintended request to a different website. These attacks typically target actions that have side effects, such as changing the user's data, making purchases, or transferring funds.
Here's a simple example: imagine a banking website that allows users to transfer money by sending a POST request to a specific endpoint. If an attacker can trick a logged-in user into clicking a malicious link while authenticated on the banking website, the browser will unknowingly send the POST request to transfer money to the attacker's account.
Preventing CSRF Attacks in Java Web Apps
Now that we understand the risks associated with CSRF attacks, let's explore how to prevent them in Java web applications. We will discuss two primary mechanisms for CSRF protection: Synchronizer Token Pattern and SameSite Cookies.
Synchronizer Token Pattern
The Synchronizer Token Pattern, also known as Double Submit Cookies, is a widely-used technique for mitigating CSRF attacks. It involves generating a unique token for each user session and including it in both the request body and a custom HTTP header.
Let's take a look at how this pattern can be implemented in Java using Spring Security. In this example, we'll create a simple form with a hidden CSRF token and configure Spring Security to validate the token on each POST request.
// Example of generating and validating CSRF token in Java using Spring Security
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.csrf()
.csrfTokenRepository(CookieCsrfTokenRepository.withHttpOnlyFalse());
}
@Bean
public CsrfTokenRepository csrfTokenRepository() {
CookieCsrfTokenRepository repository = CookieCsrfTokenRepository.withHttpOnlyFalse();
repository.setCookiePath("/");
return repository;
}
}
In the above code, we configure Spring Security to use a CookieCsrfTokenRepository
and set the HTTP cookie flag to false
to make the token accessible to the client-side code. This ensures that the CSRF token is included in each request and validated by the server.
SameSite Cookies
Another effective measure for mitigating CSRF attacks is the use of SameSite cookies. By setting the SameSite
attribute to Strict
or Lax
, you can control when cookies are sent in cross-origin requests, thus preventing CSRF attacks.
Let's consider an example of setting SameSite cookies in a Java web application using Servlet 4.0. In the following code snippet, we configure a session cookie with the SameSite
attribute set to Strict
:
// Example of setting SameSite cookie attribute in Java using Servlet 4.0
@WebServlet("/example")
public class ExampleServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
Cookie cookie = new Cookie("sessionToken", "abc123");
cookie.setPath("/");
cookie.setHttpOnly(true);
cookie.setSecure(true);
cookie.setSameSite(SameSitePolicy.STRICT);
response.addCookie(cookie);
}
}
In this code, we create a session cookie with the SameSite
attribute set to Strict
, ensuring that the cookie is only sent in a first-party context and not in cross-origin requests, thus preventing CSRF attacks.
Closing the Chapter
In conclusion, securing your Java web application from CSRF attacks is essential for safeguarding sensitive user data and maintaining the integrity of your application. By implementing measures such as the Synchronizer Token Pattern and SameSite cookies, you can significantly reduce the risk of CSRF vulnerabilities.
It's important to stay informed about the latest best practices and security features in Java web development to ensure that your applications remain resilient to evolving security threats. Remember, proactive defense is the key to a secure and robust Java web application.