Troubleshooting Common Invalid Test Attributes
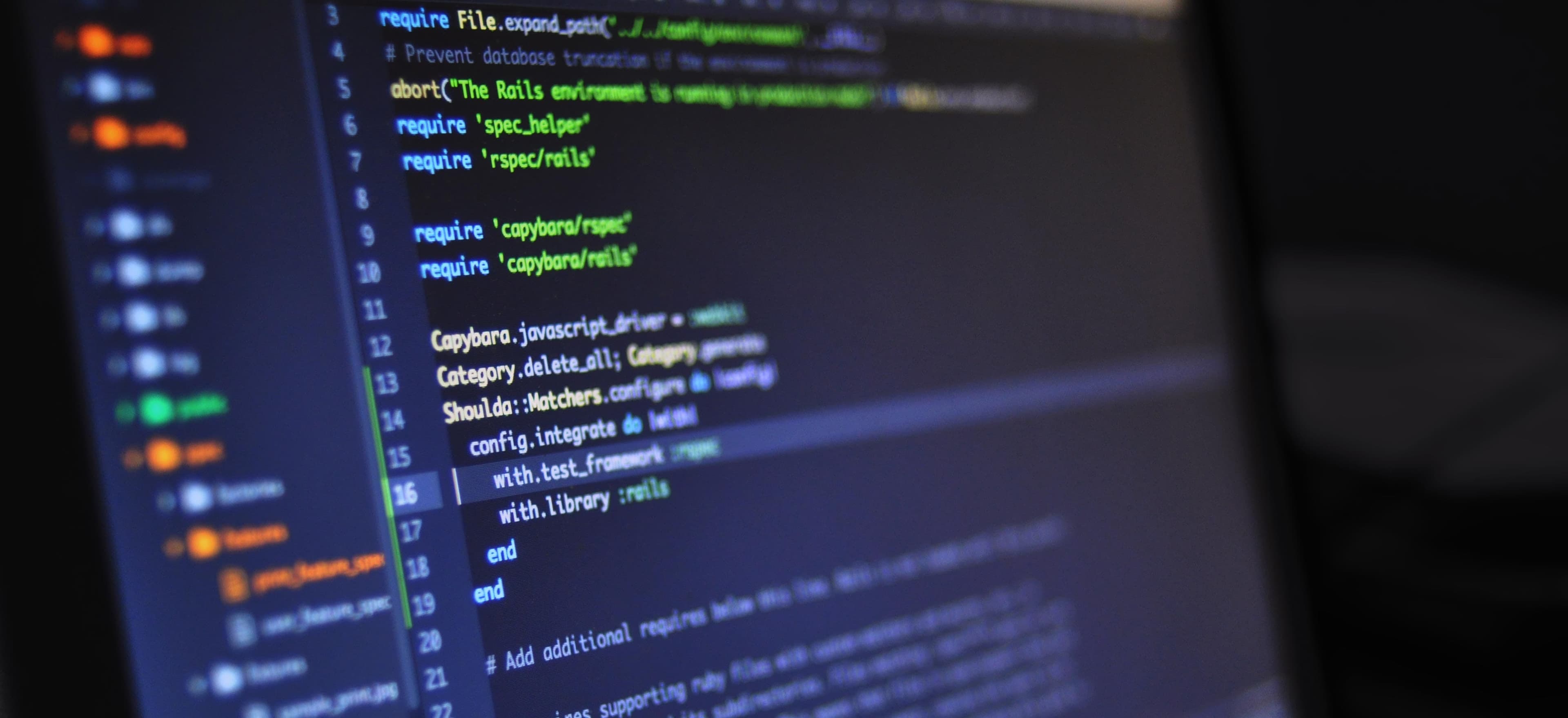
- Published on
Troubleshooting Common Invalid Test Attributes
In Java, annotations are a powerful way to add metadata to code. When writing tests, using annotations can provide valuable information to the test runner and ensure that the tests are executed correctly. However, it's common for developers to encounter issues with invalid test attributes when using annotations. This can lead to tests not running as expected or even failing altogether. In this article, we'll discuss some common invalid test attributes in Java and how to troubleshoot and resolve them.
Understanding Test Annotations in Java
Before diving into specific invalid test attributes, let's first understand the role of annotations in Java tests. Annotations are a form of metadata that can be added to Java code, including test methods and classes. They provide information about the code to the compiler, test runners, or other tools.
In the context of testing, two commonly used annotations are @Test
and @DisplayName
. The @Test
annotation is used to mark a method as a test method, while @DisplayName
is used to provide a custom display name for the test in the test report.
Here's an example of how these annotations are used:
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class ExampleTest {
@Test
@DisplayName("Test Addition")
public void testAddition() {
int result = Calculator.add(2, 3);
assertEquals(5, result, "Addition should return the correct sum");
}
}
In this example, the testAddition
method is annotated with @Test
, indicating that it is a test method. Additionally, the @DisplayName
annotation provides a custom name for the test, which will be displayed in the test report.
Common Invalid Test Attributes and How to Troubleshoot Them
1. Missing or Incorrect Imports
One common issue when working with test annotations is missing or incorrect imports. If the test annotations are not imported correctly, the compiler will not recognize them, leading to errors. To troubleshoot this issue, ensure that the correct import statements are included at the beginning of the test file.
For example, in JUnit 5, the @Test
and @DisplayName
annotations are part of the org.junit.jupiter.api
package. Make sure to include the following import statements:
import org.junit.jupiter.api.DisplayName;
import org.junit.jupiter.api.Test;
2. Incorrect Annotation Usage
Another common issue is using annotations incorrectly. For example, applying a test annotation to a method that is not intended to be a test method can lead to unexpected behavior. It's important to review the documentation for the specific annotation to understand its correct usage.
In the case of the @Test
annotation, ensure that it is only applied to methods that are meant to serve as test methods. Similarly, when using @DisplayName
, only annotate test methods that require a custom display name.
3. Typographical Errors
Typographical errors are another source of problems when working with annotations. Make sure that the annotations are spelled correctly, including the correct case. For example, @Test
must be spelled exactly as shown, with a capital T.
Final Considerations
In conclusion, understanding and troubleshooting invalid test attributes in Java is crucial for ensuring the proper execution of tests. By addressing issues such as missing imports, incorrect annotation usage, and typographical errors, developers can maintain the integrity and reliability of their test suite.
Remember, annotations are a powerful tool for conveying metadata about code, and using them correctly is essential for effective test-driven development in Java.
For further reading, you can explore the official documentation of JUnit 5, which provides comprehensive information on writing tests with annotations. Additionally, you may also find useful information in the JUnit 5 User Guide.