Guaranteeing Order in Kafka: Overcoming Partitioning Challenges
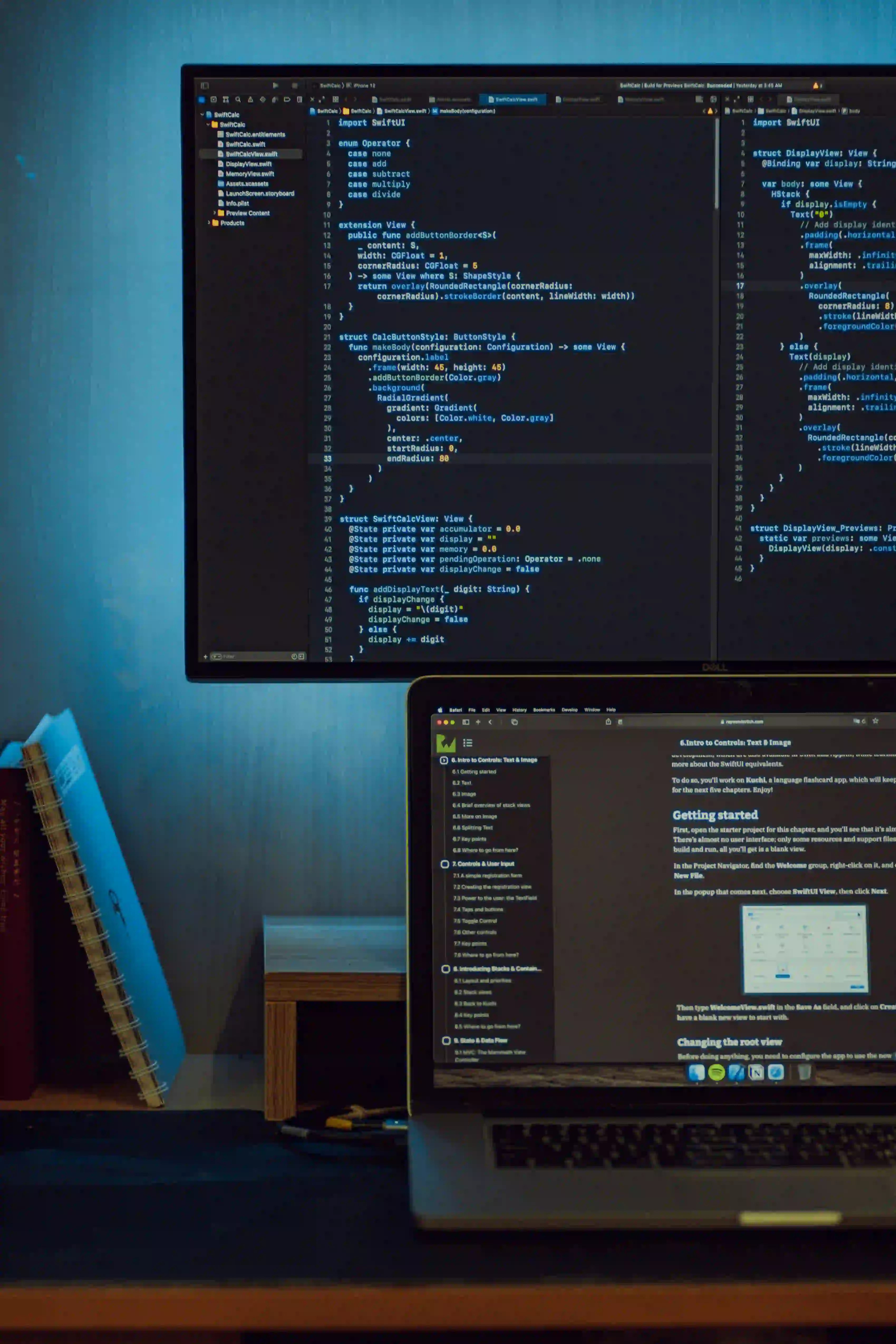
Guaranteeing Order in Kafka: Overcoming Partitioning Challenges
Apache Kafka is a distributed streaming platform designed to provide a fault-tolerant, highly scalable, and reliable way to publish and subscribe to streams of records. It accomplishes this by partitioning data and distributing it across a cluster of brokers. While this architecture provides great benefits in terms of scalability and fault tolerance, it can also introduce challenges with maintaining order when messages are produced and consumed.
The Challenge of Order
When messages are produced to Kafka, they are appended to a partition in the order they are received. However, when consuming messages from multiple partitions, the order in which they are processed is not guaranteed. This can be a problem for applications where maintaining the message order is crucial, such as processing financial transactions or maintaining a log of events.
Understanding Message Ordering in Kafka
To understand how to guarantee order in Kafka, it's essential to understand how messages are distributed across partitions.
Partitioning
Partitions in Kafka serve as the unit of parallelism. Each partition is an ordered, immutable sequence of records that is continually appended to. When a message is produced without specifying a key or partition, the message is assigned to a random partition. If a key is provided, Kafka uses a hashing algorithm to determine the partition for the message based on the key.
Consumer Groups
Consumers in Kafka are part of a consumer group, which allows a group of consumers to divide the topic partitions amongst themselves for parallel processing. Each message within a partition is delivered to only one consumer in the group.
Now let's delve into techniques for overcoming the challenges of message ordering in Kafka.
Techniques for Guaranteeing Order
Single Partition Per Key
One way to guarantee message order is by ensuring that all messages for a specific key are sent to the same partition. This way, when consuming messages for that key, they will be in the correct order within that partition.
Example Code:
ProducerRecord<String, String> record = new ProducerRecord<>("topic", key, value);
producer.send(record);
Why This Works:
By specifying a key when producing a message, Kafka ensures that all messages with the same key will always go to the same partition. This allows consumers to process messages for a specific key in order within a single partition.
One Consumer Per Partition
Another technique to ensure order is to have only one consumer per partition. This way, each message within a partition is consumed and processed in the order it was appended.
Example Code:
properties.put("group.id", "consumer-group");
properties.put("max.poll.records", 1);
Why This Works:
By setting the max.poll.records
property to 1, we ensure that only one record is consumed in each poll, allowing the consumer to process messages in the order they are stored within the partition.
Idempotent Producers
Using idempotent producers ensures that messages are delivered exactly once in the face of network failures and retries. This helps in guaranteeing order when producing messages.
Example Code:
properties.put("enable.idempotence", true);
Why This Works:
By enabling idempotence, the producer assigns a sequence number to each message, allowing the broker to detect and reject duplicate messages. This helps in maintaining the order of messages within a partition.
My Closing Thoughts on the Matter
Maintaining message order is a crucial aspect of many applications. Although Kafka's distributed nature can introduce challenges in this regard, by understanding its partitioning strategy and implementing specific techniques like key-based partitioning, single consumer per partition, and idempotent producers, it is possible to guarantee order in Kafka.
By applying these techniques, developers can ensure that their Kafka-based applications meet the strict ordering requirements, enabling them to build robust and reliable systems.
For further exploration of this topic, the official Apache Kafka documentation provides in-depth information about the concepts discussed here. Additionally, the Kafka community and Confluent blog offer valuable insights and best practices for working with Kafka in real-world scenarios.