Optimizing Testability with a Smart Package Structure
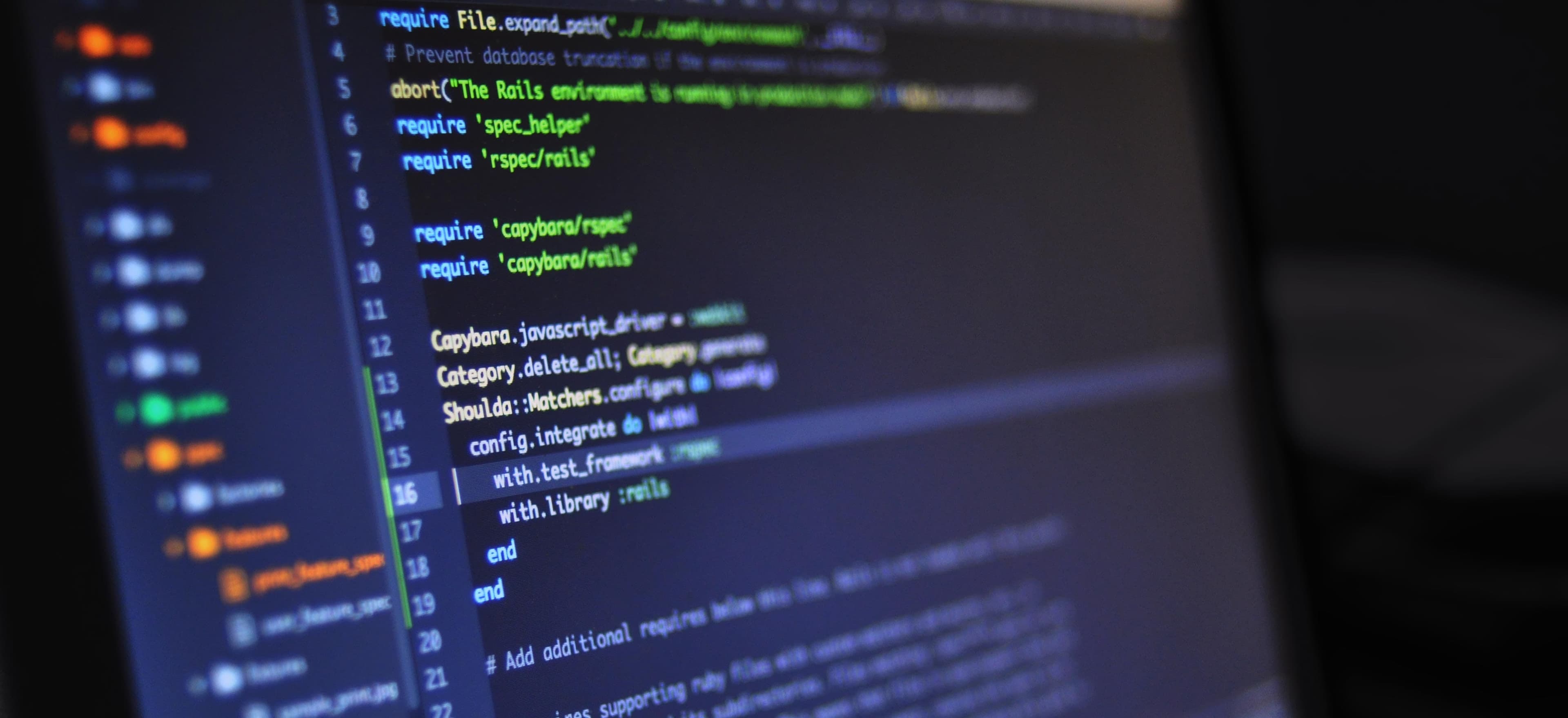
- Published on
Optimizing Testability with a Smart Package Structure
When it comes to developing software applications, writing code is just one part of the equation. It's crucial to ensure that the code is testable to validate its functionality through unit testing, integration testing, and other forms of testing. Testability is a key aspect of software quality, and it can be enhanced by structuring the codebase in a way that makes testing easier and more effective. In this blog post, we'll explore how to optimize testability in Java applications by using a smart package structure.
The Importance of Testability
Testability is the degree to which a software application supports testing efforts. A highly testable codebase allows for the efficient creation and execution of tests, which in turn leads to more reliable and maintainable software. Testable code is typically modular, loosely coupled, and easy to set up for testing.
In the context of Java applications, achieving high testability involves not only writing testable code but also organizing the codebase in a way that facilitates testing. A well-designed package structure plays a significant role in this aspect.
The Role of Package Structure in Testability
The package structure of a Java application determines how code is organized into different modules or components. A smart and logical package structure can greatly enhance the testability of the application. It can help in isolating components for testing, promoting reusability, and reducing coupling between different parts of the codebase.
When it comes to testing, it's beneficial to separate production code from test code. This segregation helps in maintaining a clear boundary between the actual application logic and the testing logic. A well-structured package layout can enforce this separation and make it easier to manage and maintain the tests alongside the production code.
In the following sections, we'll delve into specific strategies for organizing the package structure of a Java application to optimize testability.
Layered Package Structure
One common approach to structuring Java applications is to use a layered architecture, where different layers represent distinct responsibilities and concerns. A typical layered architecture includes layers such as presentation/UI, business logic, and data access.
By reflecting these layers in the package structure, you can create clear boundaries and isolation between different parts of the system. This separation makes it easier to test individual layers in isolation, leading to more focused and targeted testing efforts.
Consider the following package structure for a web application:
com.example
├── controller
├── service
├── repository
├── model
└── util
In this example, the controller
package contains classes responsible for handling web requests and coordinating the flow of data. The service
package holds the business logic and application-specific functionality. The repository
package encapsulates the data access layer, interfacing with the underlying database or data storage. The model
package includes domain objects and data transfer objects, while the util
package houses utility classes and helpers.
This organized package structure allows for clear separation of concerns, making it easier to write targeted tests for each layer without having to deal with the complexities of the other layers.
Module-Based Package Structure
In larger Java applications, especially those built using modular frameworks like Spring or OSGi, a module-based package structure can be highly beneficial for testability. In this approach, each functional module or feature of the application is encapsulated within its own package or set of packages.
For example, in a modular e-commerce application, the package structure might look like this:
com.example
├── customer
│ ├── controller
│ ├── service
│ ├── repository
│ └── model
├── product
│ ├── controller
│ ├── service
│ ├── repository
│ └── model
└── order
├── controller
├── service
├── repository
└── model
Here, each major feature or module (e.g., customer management, product catalog, order processing) has its own dedicated package structure, containing the necessary components for that specific feature. This arrangement allows for focused testing within each module, reducing the scope and complexity of the tests.
Moreover, module-based package structures promote code reusability and maintainability, as each module is self-contained and can be developed, tested, and updated independently.
Test-Specific Package Structure
In addition to organizing the production codebase, it can be advantageous to create a separate package structure specifically for test code. This helps in distinguishing tests from the production code, making it easier to manage, execute, and maintain the test suite.
A typical test-specific package structure might look like this:
com.example
├── controller
│ └── ControllerTest.java
├── service
│ └── ServiceTest.java
├── repository
│ └── RepositoryTest.java
└── util
└── UtilTest.java
In this example, each package from the production code has a corresponding package for test code, with test classes named in a recognizable pattern (e.g., *Test.java
). This alignment between the production and test packages allows for easy navigation and mapping between the source code and its corresponding tests.
Separating the test code into its own package structure also enables the application of different testing strategies, such as integration tests, unit tests, and component tests, without cluttering the production codebase.
Creating Testable Code with Dependency Injection
While organizing the package structure is important for testability, writing code that is inherently testable is equally crucial. In Java applications, using dependency injection is a powerful technique for creating testable code.
By employing dependency injection frameworks like Spring or Guice, you can externalize dependencies and make them configurable. This allows for easier substitution of real dependencies with mock objects or test doubles during testing.
Let's consider a simple example of dependency injection using Spring:
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// ... (other methods)
}
In this example, the UserService
class declares a dependency on the UserRepository
. By using constructor injection and annotating the constructor with @Autowired
, the dependency is injected from the Spring application context. During testing, you can easily provide a mock implementation of UserRepository
for isolated unit testing of the UserService
.
By designing classes with dependency injection in mind and keeping dependencies outside of the class, you promote loose coupling and isolation, making the code more amenable to testing and refactoring.
To Wrap Things Up
In this blog post, we've explored the significance of testability in Java applications and how a well-structured package layout can enhance testability. The package structure plays a crucial role in segregating different parts of the system, promoting isolation, and simplifying testing efforts.
By adopting a layered or module-based package structure and creating a dedicated package for test code, you can streamline your testing processes and make your applications more robust and maintainable. Additionally, leveraging dependency injection allows you to write code that is inherently testable and adaptable to different testing scenarios.
Optimizing testability with a smart package structure is not only about enabling easier testing but also about fostering good software design practices. It encourages modularity, decoupling, and reusability, leading to code that is easier to understand, maintain, and extend.
In the ever-evolving landscape of software development, prioritizing testability through thoughtful package structuring is a strategic investment that pays off in the form of more reliable, adaptable, and high-quality codebases.
Testability matters, and the package structure is a valuable ally in the pursuit of better software testing and development practices.
Happy coding and testing!
To dive deeper into testability and package structuring, check out Martin Fowler's article on "What is a package?".