Troubleshooting Common Serialization Errors
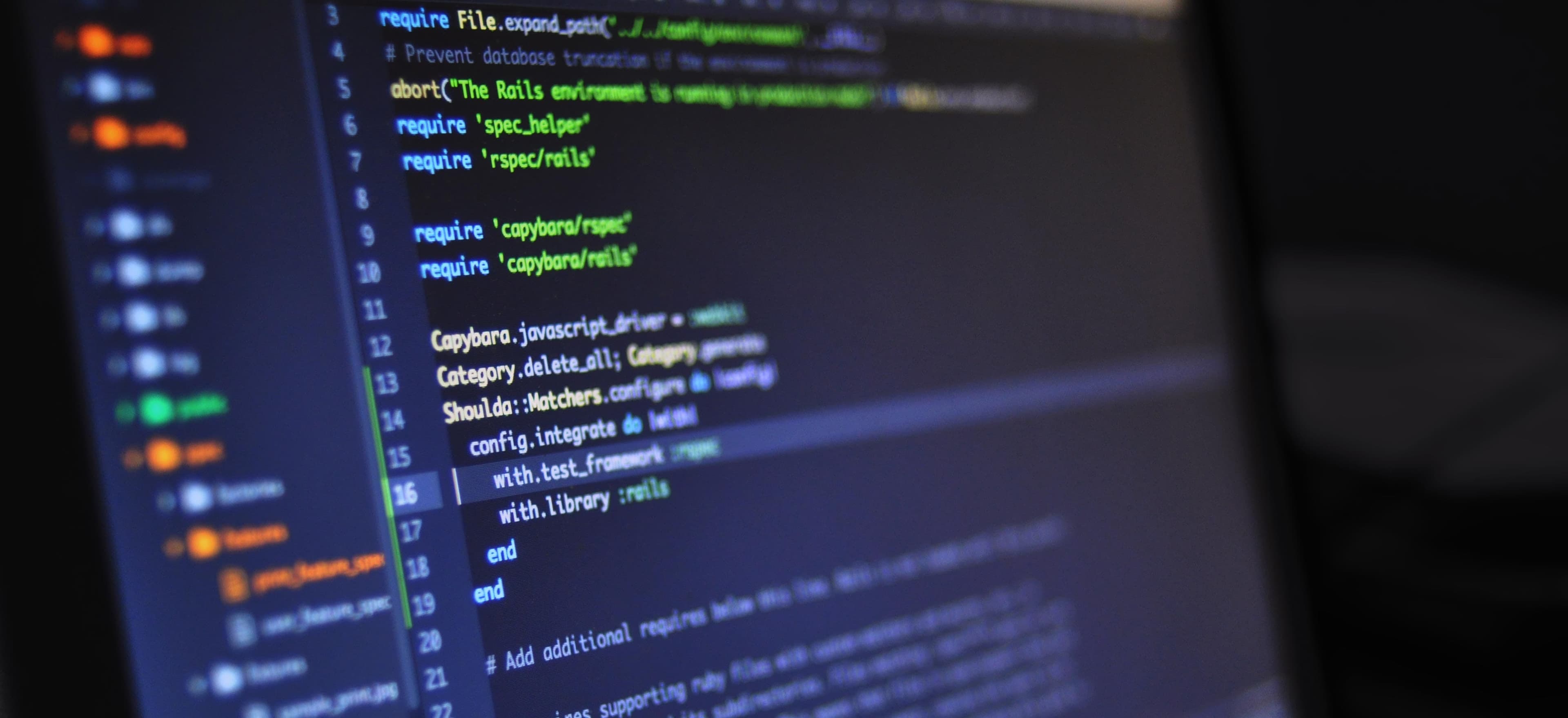
- Published on
Understanding Java Serialization Errors
Serialization is a fundamental aspect of Java programming, allowing objects to be converted into a stream of bytes for storage or transmission. While the process seems straightforward, developers often encounter a range of errors when working with serialization in Java. Understanding and solving these issues is crucial for maintaining the integrity of your applications.
In this article, we'll explore some common Java serialization errors and how to troubleshoot them effectively.
1. serialVersionUID Mismatch
When serializing objects, each serializable class has a unique identifier called serialVersionUID
. When the class is deserialized, Java uses this identifier to verify that the sender and receiver of a serialized object have loaded classes for that object that are compatible with respect to serialization.
If the serialVersionUID
of the serialized object does not match the serialVersionUID
of the receiving class, a InvalidClassException
is thrown. To resolve this, you can either manually set the serialVersionUID
in the class or implement the java.io.Serializable
interface and do not declare a serialVersionUID
.
// Example of manually setting the serialVersionUID
private static final long serialVersionUID = 1L;
2. ClassNotFoundException
This error occurs when the class being deserialized is not found in the classpath of the receiving application. It can happen if the class has been renamed, moved to a different package, or is not available in the classpath.
Ensure that the receiving application has the correct class in its classpath. If the class has been moved or renamed, consider using a custom readObject
and writeObject
method to handle these cases.
3. NotSerializableException
This exception is thrown when an object is not serializable. Ensure that all fields within the object are declared as transient
if they cannot be serialized, or implement the java.io.Serializable
interface in the class.
4. Externalizable interface not implemented
If a class implements the Externalizable
interface, serialization depends entirely on the class, and standard serialization is bypassed. When using Externalizable
, ensure that the readExternal
and writeExternal
methods are implemented correctly.
public class MyClass implements Externalizable {
@Override
public void writeExternal(ObjectOutput out) throws IOException {
// Custom serialization logic
}
@Override
public void readExternal(ObjectInput in) throws IOException, ClassNotFoundException {
// Custom deserialization logic
}
}
Lessons Learned
Serialization errors are common in Java, but with a clear understanding of the underlying causes and mechanisms, these issues can be effectively mitigated. By addressing serialVersionUID
mismatches, handling ClassNotFoundException
, and resolving NotSerializableException
and Externalizable
interface issues, developers can ensure smooth and error-free serialization in their applications.
For further insights into Java serialization, you can refer to the Java Object Serialization documentation. Additionally, exploring real-world scenarios and practicing serialization techniques will enhance your troubleshooting skills in this domain.