Understanding Lambda Expressions in Java
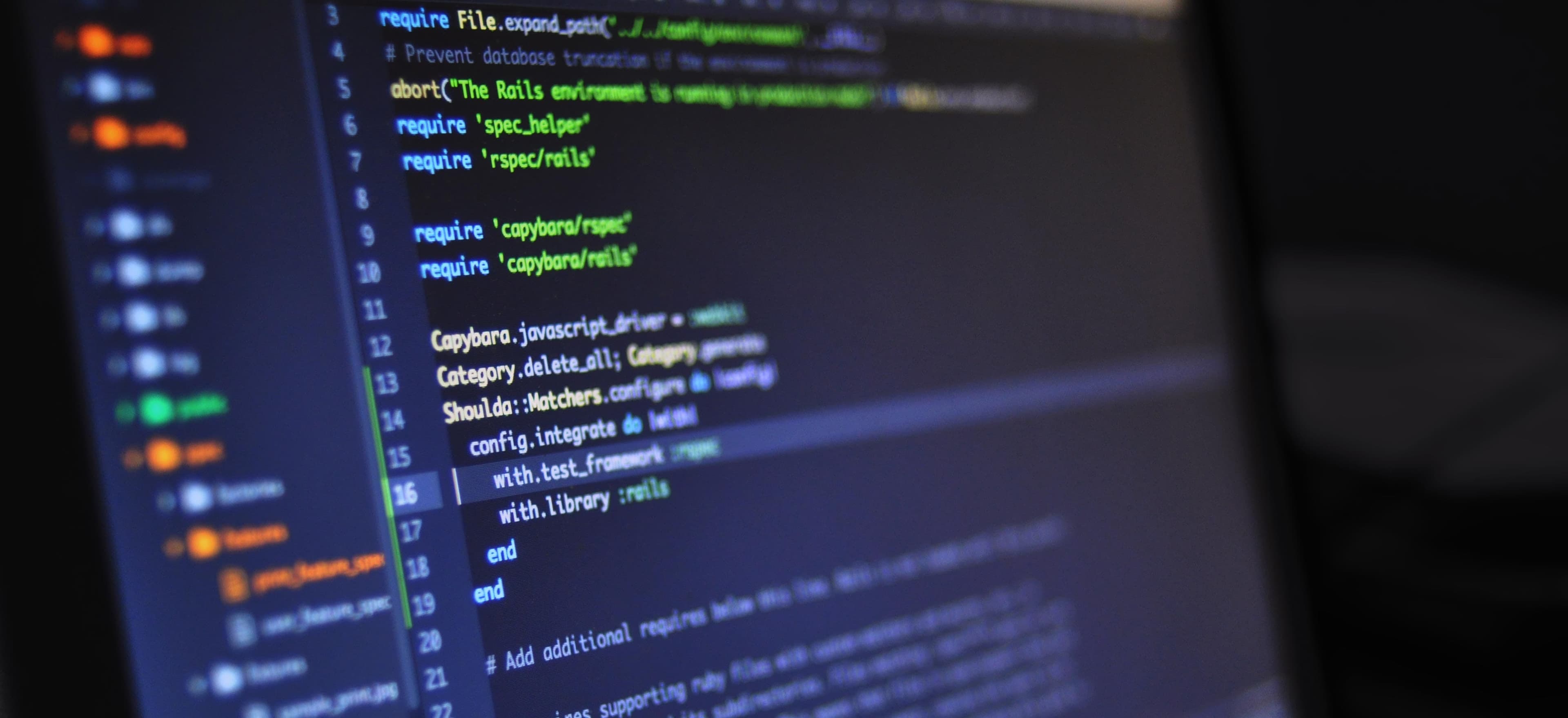
- Published on
Understanding Lambda Expressions in Java
In Java programming, lambda expressions are a powerful and concise way to represent a piece of functionality. They are a key feature of Java 8 and have greatly simplified the process of writing clean and efficient code. In this article, we will explore lambda expressions in Java, understand their syntax, and see how they can be used to write more expressive and compact code.
What are Lambda Expressions?
Simply put, a lambda expression is an anonymous function – a method without a name. It provides a clear and concise way to represent one method interface using an expression. Lambda expressions are particularly useful in situations where you need to pass functionality as an argument to a method, like when working with collections or threads.
Syntax of Lambda Expressions
The syntax for a lambda expression consists of the following components:
(parameters) -> expression
or
(parameters) -> { statements; }
-
parameters
: This is the input parameters required by the lambda expression. If there are no parameters, you can use an empty set of parentheses()
. If there is only one parameter, you can optionally omit the parentheses. For example,x -> x * x
represents a lambda expression with a single parameter. -
->
: This is the lambda operator, which separates the parameters from the body of the lambda expression. -
expression
or{ statements; }
: This is the body of the lambda expression. If the expression contains a single statement, you can use just the expression. If there are multiple statements, you need to enclose them in curly braces.
Example of Lambda Expression
Let's consider a simple example of sorting a list of strings using lambda expressions:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
// Prior to Java 8
Collections.sort(names, new Comparator<String>() {
@Override
public int compare(String s1, String s2) {
return s1.compareTo(s2);
}
});
// With lambda expression
Collections.sort(names, (s1, s2) -> s1.compareTo(s2));
In this example, the lambda expression (s1, s2) -> s1.compareTo(s2)
represents the compare
method of the Comparator
interface. The code is much more concise and readable compared to the anonymous inner class implementation.
Functional Interfaces
Lambda expressions are used primarily to implement functional interfaces – interfaces that specify exactly one abstract method. These interfaces provide a target type for lambda expressions and method references.
@FunctionalInterface
interface MyFunction {
int apply(int x, int y);
}
By annotating the interface with @FunctionalInterface
annotation, you can ensure that the interface is a functional interface, and the compiler will flag an error if the interface has more than one abstract method.
Using Lambda Expressions
Lambda expressions are widely used in the Java ecosystem, and they provide a clean and concise way to express behavior.
1. Using with Collections
Lambda expressions are commonly used when working with collections in Java. For example, you can use them with the forEach
method to iterate over a collection:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
names.forEach(name -> System.out.println(name));
2. Using with Threads
You can also use lambda expressions when working with threads. Instead of creating a new subclass of Thread
or implementing Runnable
, you can use a lambda expression to define the behavior of the thread:
Thread myThread = new Thread(() -> {
// Code to be executed in the thread
});
3. Using as Method References
Lambda expressions can be used to create method references, which are shorthand notation for a lambda expression calling a method:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David");
names.sort(String::compareToIgnoreCase);
In this example, String::compareToIgnoreCase
is a method reference to the compareToIgnoreCase
method of the String
class.
Benefits of Lambda Expressions
Lambda expressions offer several benefits, including:
-
Conciseness: Lambda expressions allow you to write concise code, reducing the verbosity of anonymous classes.
-
Readability: They make the code more readable by eliminating the noise of anonymous inner classes.
-
Flexibility: Lambda expressions provide a flexible way to pass behavior as an argument to a method, enhancing the expressiveness of the language.
-
Parallelism: They facilitate the writing of parallel and concurrent code by enabling a functional programming style.
The Last Word
In conclusion, lambda expressions in Java provide a succinct and expressive way to represent functionality as data. By understanding their syntax and benefits, you can leverage lambda expressions to write clean, readable, and efficient code. They are an essential feature of modern Java programming and are widely used in various libraries and frameworks.
Understanding lambda expressions is crucial for any Java developer aspiring to write modern, efficient, and clean code.
To deepen your understanding of lambda expressions in Java, I recommend checking out this comprehensive guide on Oracle's official documentation, which provides in-depth insight into this powerful feature.
Happy coding!