Effective Use of Builder Pattern for Creating Objects
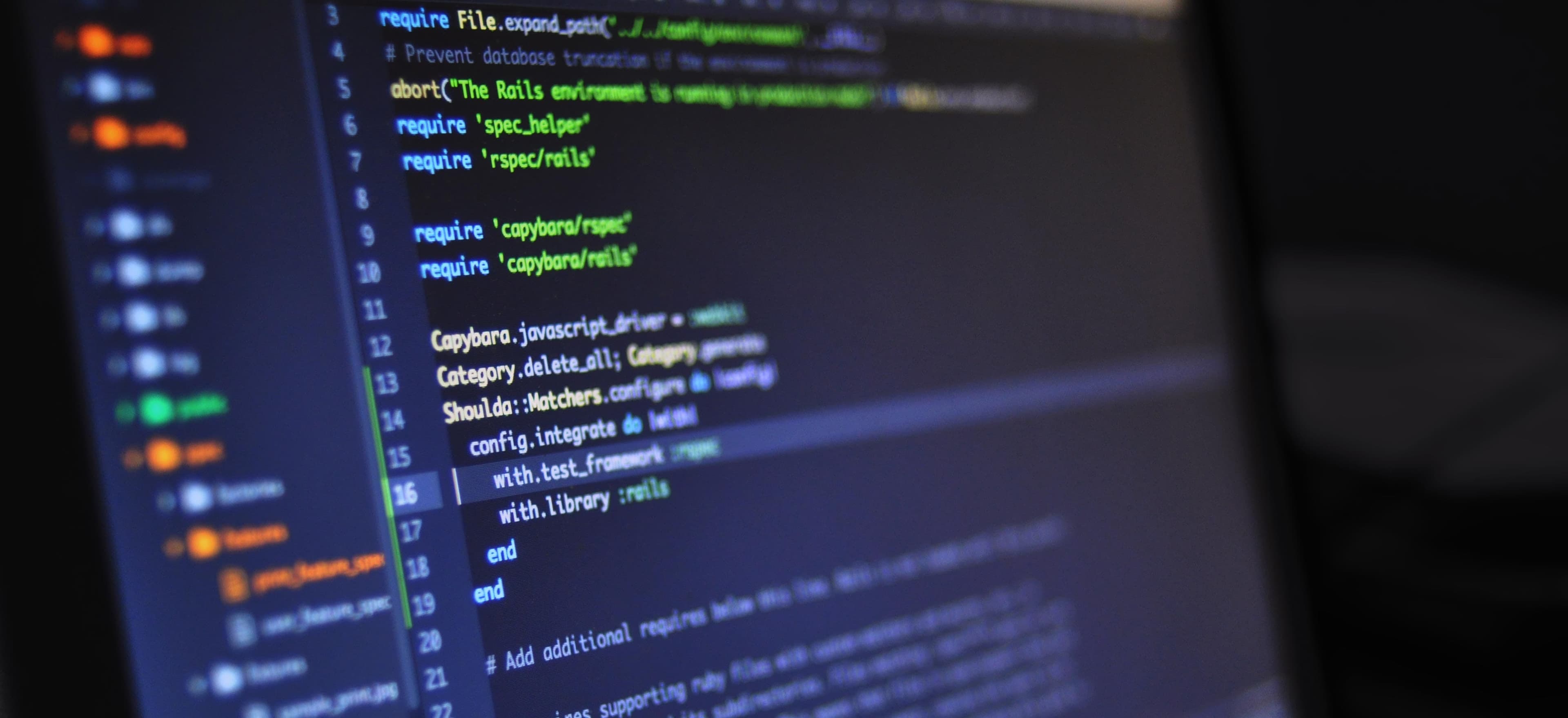
- Published on
The Power of Builder Pattern in Java
In the world of software development, creating objects effectively and maintaining code readability are essential aspects. The Builder Pattern, a creational design pattern, plays a pivotal role in addressing these concerns by providing a solution to construct complex objects while keeping the construction process simple and intuitive.
Understanding the Builder Pattern
The Builder Pattern separates the construction of a complex object from its representation, allowing the same construction process to create different representations. In Java, the Builder Pattern involves creating a separate builder class that contains methods for setting the properties of the object to be created. This builder class is then used to construct the final object with the desired properties.
Advantages of Using the Builder Pattern
1. Readability
The Builder Pattern enhances code readability by providing clear and expressive methods for setting object properties. This results in more intuitive and understandable object creation code, making the overall codebase more maintainable.
2. Flexibility
By decoupling the construction process from the actual object, the Builder Pattern provides flexibility in creating different configurations of the same object. This flexibility is valuable in scenarios where multiple variations of an object need to be constructed.
3. Immutability
The Builder Pattern allows for the creation of immutable objects by enforcing the setting of all required properties at the time of object construction. This immutability ensures that the state of the object remains consistent throughout its lifecycle.
Implementing the Builder Pattern
Let's illustrate the implementation of the Builder Pattern in Java with a practical example. Consider a simple User
class with properties such as firstName
, lastName
, email
, and age
. We will create a UserBuilder
class to facilitate the construction of User
objects.
User Class
public class User {
private final String firstName;
private final String lastName;
private final String email;
private final int age;
private User(String firstName, String lastName, String email, int age) {
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
this.age = age;
}
// Getters for properties
}
UserBuilder Class
public class UserBuilder {
private String firstName;
private String lastName;
private String email;
private int age;
public UserBuilder setFirstName(String firstName) {
this.firstName = firstName;
return this;
}
public UserBuilder setLastName(String lastName) {
this.lastName = lastName;
return this;
}
public UserBuilder setEmail(String email) {
this.email = email;
return this;
}
public UserBuilder setAge(int age) {
this.age = age;
return this;
}
public User build() {
return new User(firstName, lastName, email, age);
}
}
In the UserBuilder
class, each setter method returns the instance of the UserBuilder
itself, allowing method chaining when setting the properties of the User
object. The build()
method is responsible for creating the User
object with the specified properties.
Utilizing the Builder Pattern
Now, let's utilize the UserBuilder
to create a User
object in a clear and concise manner.
public class Main {
public static void main(String[] args) {
User user = new UserBuilder()
.setFirstName("John")
.setLastName("Doe")
.setEmail("john.doe@example.com")
.setAge(30)
.build();
}
}
In this example, the method chaining and expressive nature of the UserBuilder
facilitate the creation of a User
object with specified properties without cluttering the object construction code.
When to Use the Builder Pattern
The Builder Pattern is particularly beneficial in the following scenarios:
-
Complex Object Creation: When the process of object creation involves multiple steps or requires setting numerous properties, the Builder Pattern simplifies and organizes the construction process.
-
Optional Properties: If an object has optional properties, the Builder Pattern allows for a clear and concise way of setting only the required properties, leaving the optional ones to default values or explicitly setting them when needed.
-
Immutable Objects: For creating immutable objects with a significant number of properties, the Builder Pattern ensures a consistent and straightforward approach to constructing such objects.
Bringing It All Together
In the realm of Java programming, the Builder Pattern serves as a valuable tool for creating complex objects while maintaining code readability and flexibility. By delegating the object construction process to a dedicated builder class, the Builder Pattern enhances the clarity and expressiveness of object creation code. Its ability to support method chaining and facilitate the creation of immutable objects makes it a compelling choice for scenarios involving intricate object creation requirements.
To delve deeper into design patterns and their usage in Java, consider exploring "Effective Java" by Joshua Bloch, which provides comprehensive insights into best practices for Java development.
Start leveraging the Builder Pattern in your Java projects to streamline object construction and embrace code clarity and maintainability.