Understanding the Impact of Changing Delay on Order in Network Communication
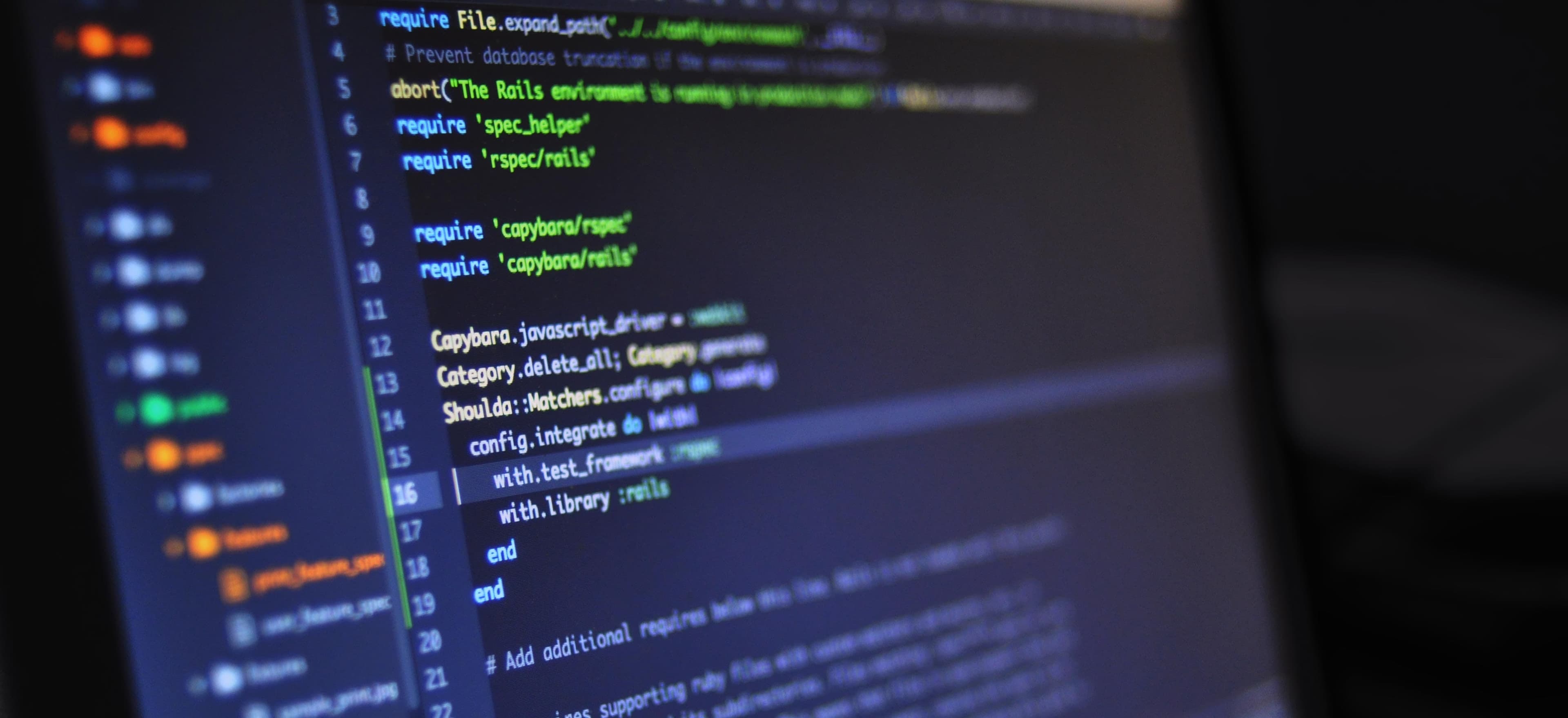
- Published on
Understanding the Impact of Changing Delay on Order in Network Communication
When we talk about network communication, ordering of messages is crucial. But what happens when there's a delay? Let's dive into the impact of changing delay on order in network communication and how Java helps in managing these scenarios.
The Importance of Order in Network Communication
In network communication, especially in distributed systems, maintaining the order of messages is vital. Consider a scenario where a client sends two messages to a server. The application logic at the server end may expect these messages to be processed in the same order as they were sent. Any disruption in the order of these messages could result in incorrect processing and potentially lead to unforeseen issues.
Understanding Delays in Network Communication
Networks are inherently unpredictable. While we'd like to think of data traveling at the speed of light, in reality, it's subject to various factors such as network congestion, routing protocols, and hardware limitations. These factors contribute to varying degrees of delay in message delivery.
Impact of Delay on Message Order
Let's consider a simple scenario to understand the impact of delay on message order.
Scenario
We have a client-server setup where the client sends two messages to the server.
- Message A: "Hello"
- Message B: "World"
In an ideal scenario with no delay, the server would receive and process Message A before Message B. However, when there's a delay in the network, the order of message processing can be affected.
Java Code Example: Simulating Delay
Let's use Java to simulate a delay in message transmission. We'll use ScheduledExecutorService
to introduce a delay before processing messages on the server side.
import java.util.concurrent.*;
public class DelayedMessageProcessor {
private static final ScheduledExecutorService executor = Executors.newScheduledThreadPool(1);
public void processMessageWithDelay(String message, long delayInSeconds) {
executor.schedule(() -> processMessage(message), delayInSeconds, TimeUnit.SECONDS);
}
private void processMessage(String message) {
System.out.println("Processing message: " + message);
}
}
In this example, processMessageWithDelay
simulates the delay using ScheduledExecutorService
. The message processing is scheduled after the specified delay, mimicking the network delay.
Impact
Introducing delay in message processing can lead to out-of-order message delivery. This can pose significant challenges in systems where message order is crucial for maintaining data integrity and consistency.
Managing Message Order in Java
Java provides several mechanisms to manage message order and handle scenarios where delay can impact the expected order of message processing. Let's explore some of these mechanisms.
1. Sequence Numbers
Using sequence numbers in messages allows the receiver to order incoming messages based on their sequence number. If a message with a higher sequence number arrives before a message with a lower sequence number, the receiver can reorder or delay the processing of the higher sequence number message until the lower sequence number message is received and processed.
public class Message {
private int sequenceNumber;
private String content;
// Getters and setters
// Other message properties and methods
}
In this example, the Message
class includes a sequenceNumber
field. The receiver can utilize this sequence number to reorder messages based on their arrival sequence.
2. Message Queues
Using message queues, such as Apache Kafka or RabbitMQ, introduces a buffer between message producers and consumers. Messages are stored in the queue and delivered to consumers in the order they were received. This helps mitigate the impact of network delays on message order.
// Code to produce and consume messages using a message queue
3. Event-Driven Architecture
Implementing an event-driven architecture allows components to react to events in a decoupled manner. When dealing with delayed messages, this approach enables components to process messages independently, reducing the dependency on strict message order.
// Implementing event-driven handling of messages in Java
By leveraging these mechanisms, developers can mitigate the impact of network delays on message order, ensuring the stability and correctness of distributed systems.
Closing the Chapter
In network communication, maintaining message order is crucial for the stability and consistency of distributed systems. Understanding the impact of changing delay on message order is essential for designing resilient and robust systems. Java provides developers with the tools and strategies to manage message order in the face of network delays, ensuring the reliability of distributed applications.
In conclusion, by incorporating relevant tools and strategies in Java, we can address the challenges posed by changing delays in network communication, ultimately enhancing the performance and reliability of distributed systems.