Debugging Common Issues in Play Framework 2
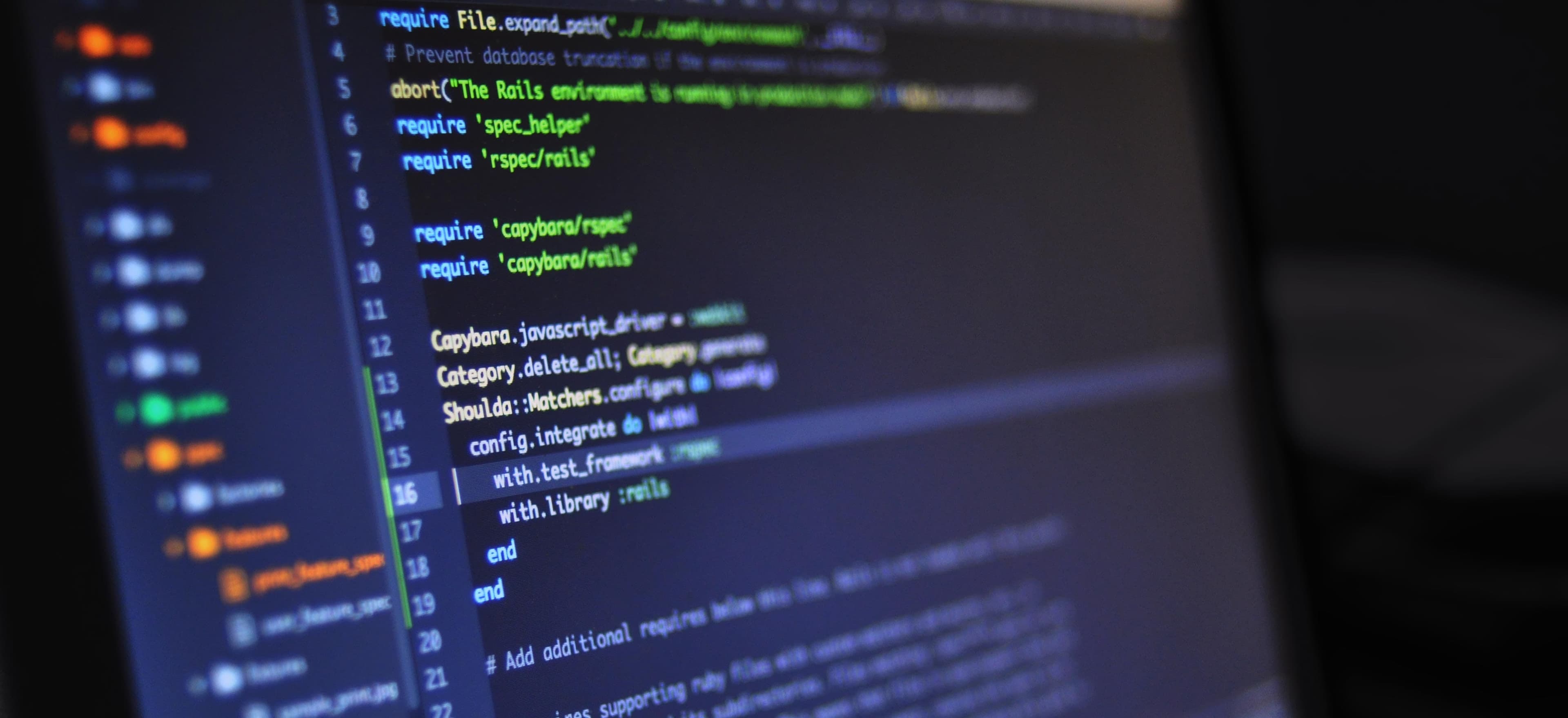
- Published on
Debugging Common Issues in Play Framework 2
Play Framework is a modern web application framework based on the Java programming language. It enables developers to build scalable and fast web applications. However, like any software, developers may encounter common issues or bugs while developing applications using Play Framework. In this post, we'll explore some common issues and how to debug them effectively.
1. Understanding ClassNotFound Exception
One of the most common issues developers face when working with Play Framework is the java.lang.ClassNotFoundException
. This exception occurs when the JVM can't find a class at runtime that it expected to be present. It can happen for various reasons, such as missing dependencies or incorrect classpath configurations.
Let's consider the following scenario, a Play Framework application using a library which cannot be found at runtime:
import com.example.SomeClass;
public class HomeController extends Controller {
public Result index() {
SomeClass.doSomething(); // This may result in a ClassNotFoundException
return ok("Hello World");
}
}
In this case, if SomeClass
is not found at runtime, a ClassNotFoundException
will be thrown. To resolve this issue, ensure that all the required dependencies are correctly included in the project's build configuration. Double-check the build.sbt
file and make sure the necessary libraries are added as dependencies.
2. Dealing with Template Compilation Errors
Play Framework uses the Twirl template engine to process and compile templates. It's not uncommon for developers to encounter compilation errors when working with templates. These errors can be due to syntax issues, incorrect imports, or invalid expressions within the templates.
Consider the following Play template code snippet:
@(title: String)
<html>
<head>
<title>@title</title>
</head>
<body>
<h1>Hello, world!</h1>
</body>
</html>
If there is a syntax error or an undefined variable within the template, it can lead to compilation errors. To debug template compilation issues, carefully review the template code and look for syntax errors, misspelled variables, or incorrect imports. Additionally, Play Framework provides helpful error messages that can assist in pinpointing the issue.
3. Resolving Routing Errors
Routing defines how HTTP requests are mapped to controller actions in Play Framework applications. It's a common source of issues, especially when dealing with complex routing configurations. Developers may encounter errors such as 404 Not Found or 500 Internal Server Error due to incorrect routing setups.
Here's an example of a Play Framework route configuration:
GET /products controllers.ProductController.list()
POST /products/new controllers.ProductController.create()
If a request to /products/new
results in a 404 error, it indicates a routing issue. To debug routing errors, carefully review the routes file (conf/routes
) and ensure that the routes are correctly defined. Look for typos, incorrect HTTP methods, or controller method mappings. Additionally, leveraging Play Framework's routing documentation can provide insights into resolving routing-related problems.
4. Handling Database Connection Issues
Play Framework provides seamless integration with databases using its built-in Ebean ORM or through third-party libraries like Slick. When working with databases, developers may encounter connection-related issues such as connection timeouts, database not found errors, or incorrect configuration settings.
Consider the following Ebean model in a Play Framework application:
import io.ebean.Model;
import javax.persistence.Entity;
@Entity
public class User extends Model {
// Class definition
}
If there are database connection issues, such as failure to establish a connection to the configured database, it can lead to runtime exceptions. To debug database connection issues, review the database configuration in application.conf
and ensure that the database credentials, connection URLs, and driver configurations are accurate. Testing the database connection outside of the Play Framework application can also help isolate database-specific problems.
5. Troubleshooting Performance Bottlenecks
Performance issues can significantly impact the scalability and responsiveness of Play Framework applications. Common performance bottlenecks include inefficient database queries, excessive object creation, or blocking I/O operations. Identifying and addressing performance bottlenecks is crucial for ensuring the smooth operation of Play Framework applications.
Consider the following snippet that demonstrates a potentially inefficient database query:
public Result listProducts() {
List<Product> products = Product.find.query().where().eq("category", "electronics").findList();
return ok(views.html.products.list.render(products));
}
In this case, if the database query takes a substantial amount of time to execute, it can impact the overall application performance. To troubleshoot performance bottlenecks, utilize Play Framework's built-in profiling and monitoring tools. Additionally, consider optimizing database queries, caching frequently accessed data, and employing asynchronous programming techniques to mitigate performance issues.
The Closing Argument
In conclusion, while developing web applications using Play Framework, encountering issues and bugs is inevitable. However, by understanding common problems and knowing how to effectively debug them, developers can streamline the development process and ensure the robustness of their applications. By carefully reviewing code, configuration, and leveraging Play Framework's documentation and community resources, developers can tackle and overcome common issues with confidence.
Debugging in Play Framework is not merely about fixing errors; it's about gaining a deeper understanding of the framework and honing problem-solving skills to build resilient and efficient web applications.
Remember, embracing debugging as an integral part of the development process can lead to more reliable and performant Play Framework applications.
Happy coding!