Angular Directive Not Rendering Properly
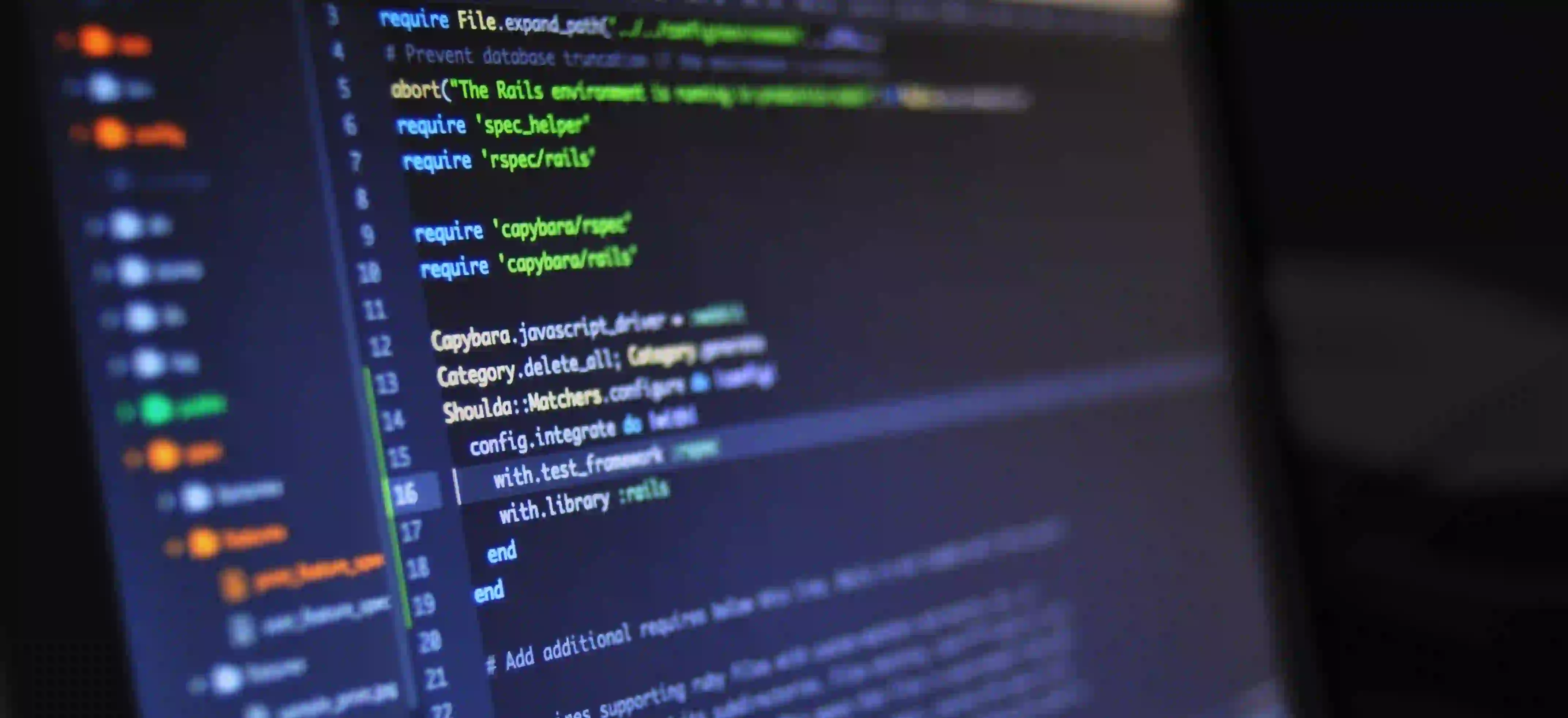
Troubleshooting Angular Directives Not Rendering Properly
If you're encountering issues with Angular directives not rendering as expected, it can be a frustrating roadblock in your development process. However, fear not, as this post will guide you through the process of identifying and resolving common issues related to this problem. We'll explore potential causes and viable solutions to help you overcome these rendering obstacles.
Understanding Angular Directives
Angular directives are a fundamental aspect of Angular development, allowing you to create reusable components and extend the functionality of HTML. However, they can sometimes present challenges when they fail to render correctly. When faced with such issues, a systematic approach to troubleshooting is necessary.
Potential Causes of Directive Rendering Issues
Several factors can contribute to directive rendering issues, such as:
- Misconfigured directive definitions
- Incorrect usage of directives within HTML templates
- Scope-related problems
- Data-binding inconsistencies
Identifying the Problem
Troubleshooting rendering issues begins with identifying the root cause of the problem. Start by inspecting the console for any error messages that may provide insight into why the directive is not rendering as expected.
Additionally, examining the directive's code and the template where it is being used can help pinpoint discrepancies or misconfigurations.
Reviewing Directive Definitions
Check the directive definition to ensure that it is properly configured. The most common mistakes include incorrectly specifying the directive's restrictions, scope, or template URL.
Example of misconfigured directive definition:
app.directive('customDirective', function() {
return {
restrict: 'E',
templateUrl: 'templates/custom-directive.html',
scope: {
// incorrect scope configuration
data: '='
},
link: function(scope, element, attrs) {
// directive logic
}
};
});
When reviewing the directive definition, pay close attention to the restrict
property, scope bindings, and template URLs. These areas are common culprits for rendering issues.
Checking Directive Usage in HTML
Ensure that you are using the directive correctly within your HTML templates. Incorrect usage can lead to rendering problems.
Example of incorrect directive usage:
<!-- Incorrect directive usage -->
<customDirective></customDirective>
In the above example, the directive is not being utilized with the appropriate syntax. Correct usage would be:
<!-- Correct directive usage -->
<custom-directive></custom-directive>
Ensure that the directive is referenced using kebab-case in HTML, matching the naming convention defined in the directive itself.
Resolving Scope Issues
Scope-related problems are another common cause of directive rendering issues. If your directive relies on scope bindings, ensure that they are set up correctly and that the necessary data is being passed from the parent scope.
Example of scope-related issue:
app.controller('MainController', function($scope) {
$scope.data = 'Sample Data';
});
<!-- Incorrect scope binding -->
<custom-directive data="data"></custom-directive>
In the above example, the incorrect use of scope binding can prevent the directive from rendering properly.
Understanding Data-Binding Inconsistencies
Data-binding inconsistencies can also lead to rendering issues. Ensure that the data you are binding to the directive is in the expected format and that it is being passed correctly.
Example of data-binding inconsistency:
<!-- Incorrect data binding -->
<custom-directive data="'Sample Data'"></custom-directive>
In the above example, the data-binding syntax is incorrect, which can cause the directive to fail in rendering the data as expected.
In Conclusion, Here is What Matters
In conclusion, troubleshooting Angular directive rendering issues involves a methodical approach of reviewing directive definitions, checking HTML usage, resolving scope-related problems, and ensuring data-binding consistency. By systematically addressing these potential problem areas, you can effectively identify and resolve rendering issues, allowing your directives to function as intended.
Remember, paying attention to detail and thoroughly reviewing your code and usage of directives is key to overcoming rendering obstacles. With the insights gained from this guide, you should be better equipped to tackle and resolve Angular directive rendering issues in your projects.
For further reading on Angular directives, refer to the official Angular documentation for detailed insights into directive usage and best practices.