Detecting and Solving Java Deadlocks
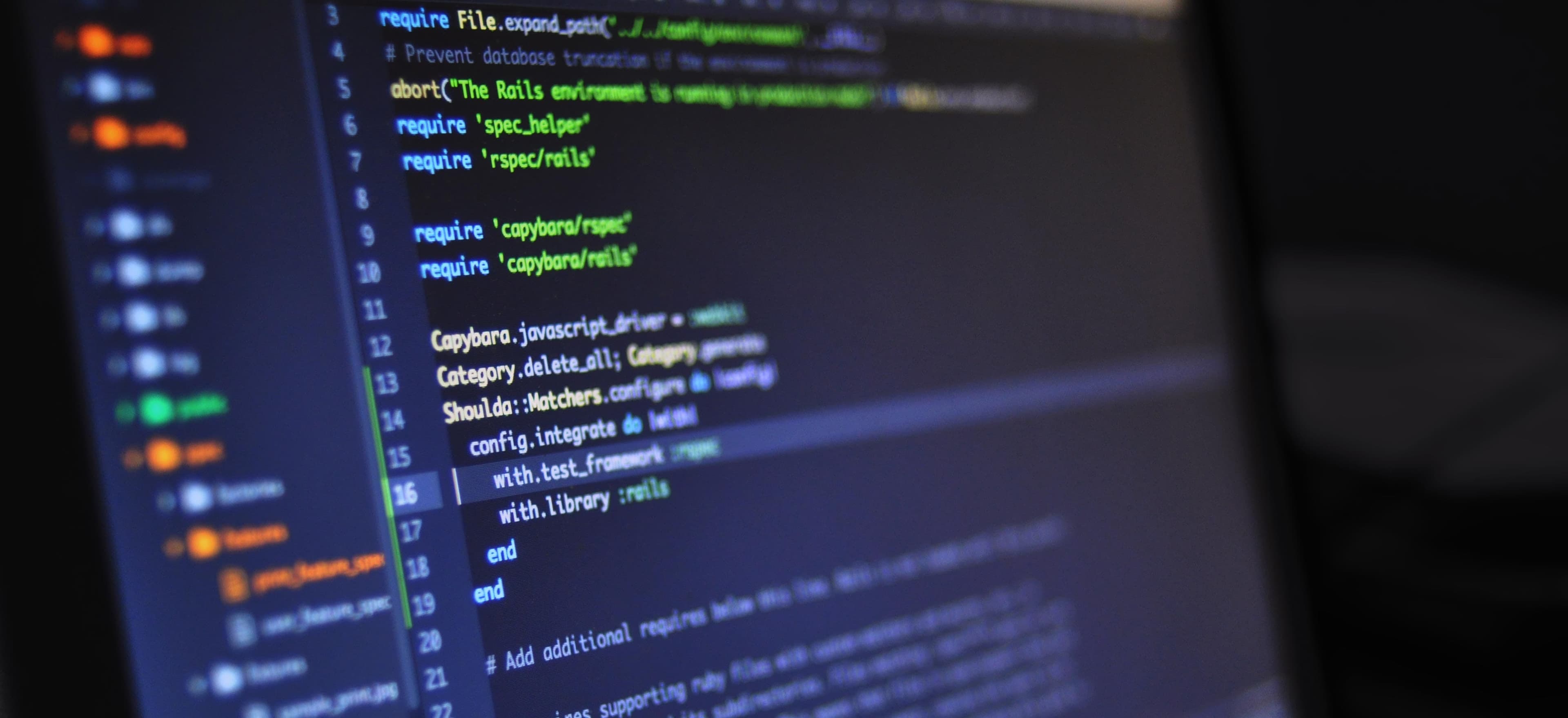
- Published on
In a multithreaded Java application, deadlocks can be a serious issue that can lead to performance degradation and unresponsive behavior. When two or more threads are blocked forever, each waiting for the other to release a lock, a deadlock occurs. In this article, we will delve into how to detect and solve deadlocks in Java.
Understanding Deadlocks
A deadlock situation arises when multiple threads need the same locks but obtain them in a different order. This can lead to a scenario where each thread indefinitely waits for the other to release the lock, resulting in a deadlock. Deadlocks can be quite elusive and not always easy to reproduce.
Here's an example of how a deadlock can occur in Java:
public class DeadlockExample {
private static final Object lock1 = new Object();
private static final Object lock2 = new Object();
public static void main(String[] args) {
Thread thread1 = new Thread(() -> {
synchronized (lock1) {
System.out.println("Thread 1: Holding lock 1...");
try { Thread.sleep(100); }
catch (InterruptedException e) {}
System.out.println("Thread 1: Waiting for lock 2...");
synchronized (lock2) {
System.out.println("Thread 1: Holding lock 1 and lock 2...");
}
}
});
Thread thread2 = new Thread(() -> {
synchronized (lock2) {
System.out.println("Thread 2: Holding lock 2...");
try { Thread.sleep(100); }
catch (InterruptedException e) {}
System.out.println("Thread 2: Waiting for lock 1...");
synchronized (lock1) {
System.out.println("Thread 2: Holding lock 1 and lock 2...");
}
}
});
thread1.start();
thread2.start();
}
}
In this example, thread1
acquires lock1
and waits for lock2
, while thread2
acquires lock2
and waits for lock1
.
Detecting Deadlocks
Detecting deadlocks in a Java application can be challenging. However, there are several approaches and tools that can help identify and diagnose deadlock situations.
Thread Dump Analysis
A thread dump provides a snapshot of what each thread is currently doing. It shows which threads are running, waiting, or blocked. By analyzing a thread dump, you can identify threads that are deadlocked.
You can generate a thread dump using various methods, such as using JConsole, VisualVM, or jstack
command-line tool.
Using tools like JConsole
JConsole is a monitoring tool that provides information about the performance and resource consumption of applications running on the Java platform. It can also be used to detect deadlocks by analyzing the thread status and stack trace of the application.
Resolving Deadlocks
Once you have identified a deadlock, you can take steps to resolve it. Here are some strategies to deal with deadlocks in Java:
Avoid Nested Locks
One way to prevent deadlocks is by avoiding nested locks. If a thread holds one lock and tries to acquire another, it may result in a deadlock. To avoid this, always acquire locks in the same order across all threads.
Timeout Mechanism
Introducing a timeout mechanism for acquiring locks can help prevent deadlocks. By specifying a maximum time to wait for a lock, threads can detect potential deadlocks and take appropriate action, such as releasing acquired locks and retrying.
Using tryLock()
The tryLock()
method in Java can be used to acquire a lock without waiting indefinitely. If the lock is not available, the thread can perform other tasks instead of blocking indefinitely. This approach allows the thread to avoid deadlock situations by acquiring locks only when they are available.
Employing Executor Framework
Using the Executor framework and concurrent data structures from the java.util.concurrent
package can help avoid deadlocks by providing higher-level abstractions for synchronization and coordination between threads.
Releasing Locks in a Specific Order
Releasing locks in the reverse order they were acquired can prevent deadlocks. This ensures that threads waiting for specific locks do not end up in a cyclic waiting scenario.
Wrapping Up
In conclusion, deadlocks in Java can be detrimental to the performance and stability of multithreaded applications. Detecting and resolving deadlocks requires a good understanding of synchronization, thread management, and the use of appropriate tools for diagnosis.
By following best practices such as avoiding nested locks, implementing timeout mechanisms, and using higher-level concurrency utilities, developers can mitigate the risk of deadlocks in their Java applications.
Remember, vigilance and proactive measures are key to keeping your Java applications free from the perils of deadlocks. Keep learning, experimenting, and refining your multithreading skills to ensure the robustness of your Java applications.
So, do you have any experiences or best practices to share in dealing with Java deadlocks? Let's talk about it in the comments!