Navigating Technical Interview Anxiety
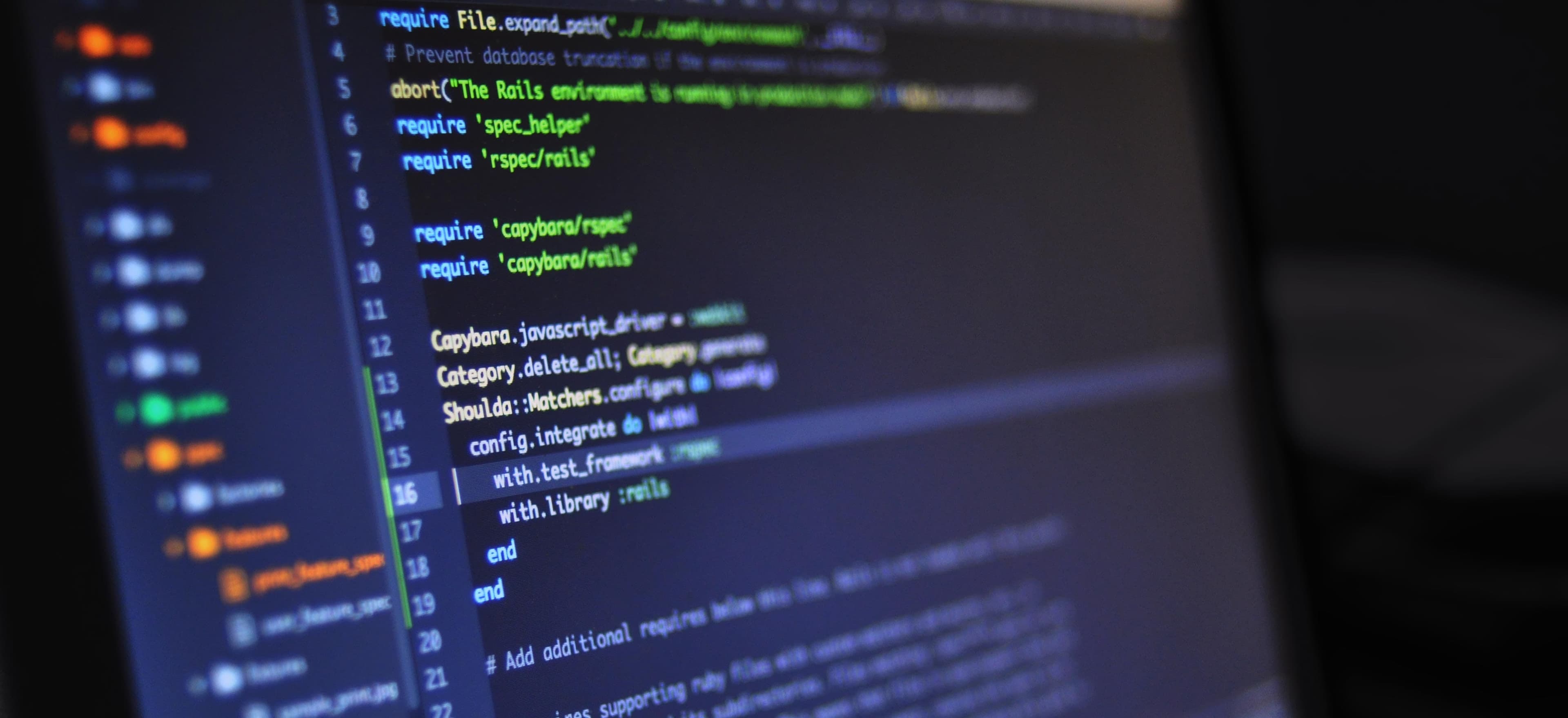
- Published on
Navigating Technical Interview Anxiety
Technical interviews are a crucial part of the hiring process for any software developer. However, for many, they can also be the source of significant anxiety and stress. This blog post aims to provide insights and strategies for navigating technical interview anxiety and performing at your best.
Understanding the Technical Interview
The technical interview is designed to assess a candidate’s technical knowledge, problem-solving abilities, and coding skills. It typically involves solving coding challenges, discussing technical concepts, and sometimes even working through real-world problems.
The Importance of Preparation
Preparation is key to overcoming anxiety in a technical interview. This involves revisiting fundamental data structures and algorithms, practicing coding problems, and familiarizing yourself with common interview questions. Online platforms like LeetCode, HackerRank, and others offer a plethora of coding challenges to help you sharpen your skills.
public class Factorial {
public static int factorial(int n) {
if (n == 0) {
return 1;
} else {
return n * factorial(n - 1);
}
}
}
Understanding the underlying reason for implementing certain algorithms is just as crucial as writing the code itself. This knowledge will not only boost your confidence but also help you approach problems with a deeper understanding.
Managing Interview Anxiety
Practice, Practice, Practice
The more you practice, the more confident and comfortable you will become with solving problems under pressure. Utilize mock interviews with friends or mentors to simulate the interview environment. In doing so, you'll learn how to communicate your thought process effectively, which is equally important as writing the code.
Deep Breathing and Visualization Techniques
Before the interview, engage in deep breathing or visualization exercises to calm your nerves. Visualize yourself approaching each problem with clarity and confidence. These simple techniques can help reduce anxiety and promote a sense of control.
Stay Positive and Embrace Failure
Acknowledge that mistakes and stumbles are part of the learning process. Embrace failure as an opportunity to grow and improve. Every challenge and setback is a chance to refine your skills and approach.
Technical Interview Best Practices
Understanding the Problem
When presented with a problem, take the time to fully grasp its requirements and constraints. Ask clarifying questions if needed before jumping into the solution. Rushing into writing code without a clear understanding often leads to errors and missteps.
public class TwoSum {
public int[] twoSum(int[] nums, int target) {
Map<Integer, Integer> numMap = new HashMap<>();
for (int i = 0; i < nums.length; i++) {
int complement = target - nums[i];
if (numMap.containsKey(complement)) {
return new int[] { numMap.get(complement), i };
}
numMap.put(nums[i], i);
}
throw new IllegalArgumentException("No two sum solution");
}
}
In the above code snippet, it's important to understand the problem of finding two numbers that add up to a specific target. The use of a hashmap to store complements improves the algorithm's efficiency, showcasing the importance of understanding the problem before diving into the solution.
Communication is Key
Articulating your thoughts and explaining your approach is crucial during a technical interview. Even if you encounter difficulties, vocalize your thought process. This not only demonstrates your problem-solving approach but also allows the interviewer to provide guidance if you stray off course.
Handling Feedback
Be open to receiving feedback during and after the interview. Constructive criticism provides valuable insights for improvement. Embrace feedback as a tool for honing your skills and becoming a stronger candidate for future opportunities.
The Closing Argument
Technical interview anxiety is a common experience, but with the right strategies, it can be managed effectively. Preparation, practice, and a positive mindset are essential components of navigating through the challenges of a technical interview. By honing your technical skills, mastering problem-solving strategies, and embracing a growth mindset, you can approach technical interviews with confidence and composure.
Remember, a technical interview is not only an assessment of your skills but also an opportunity to learn and grow. Embrace the process, stay committed to improvement, and success will follow.