Maximizing API Speed with Effective Caching
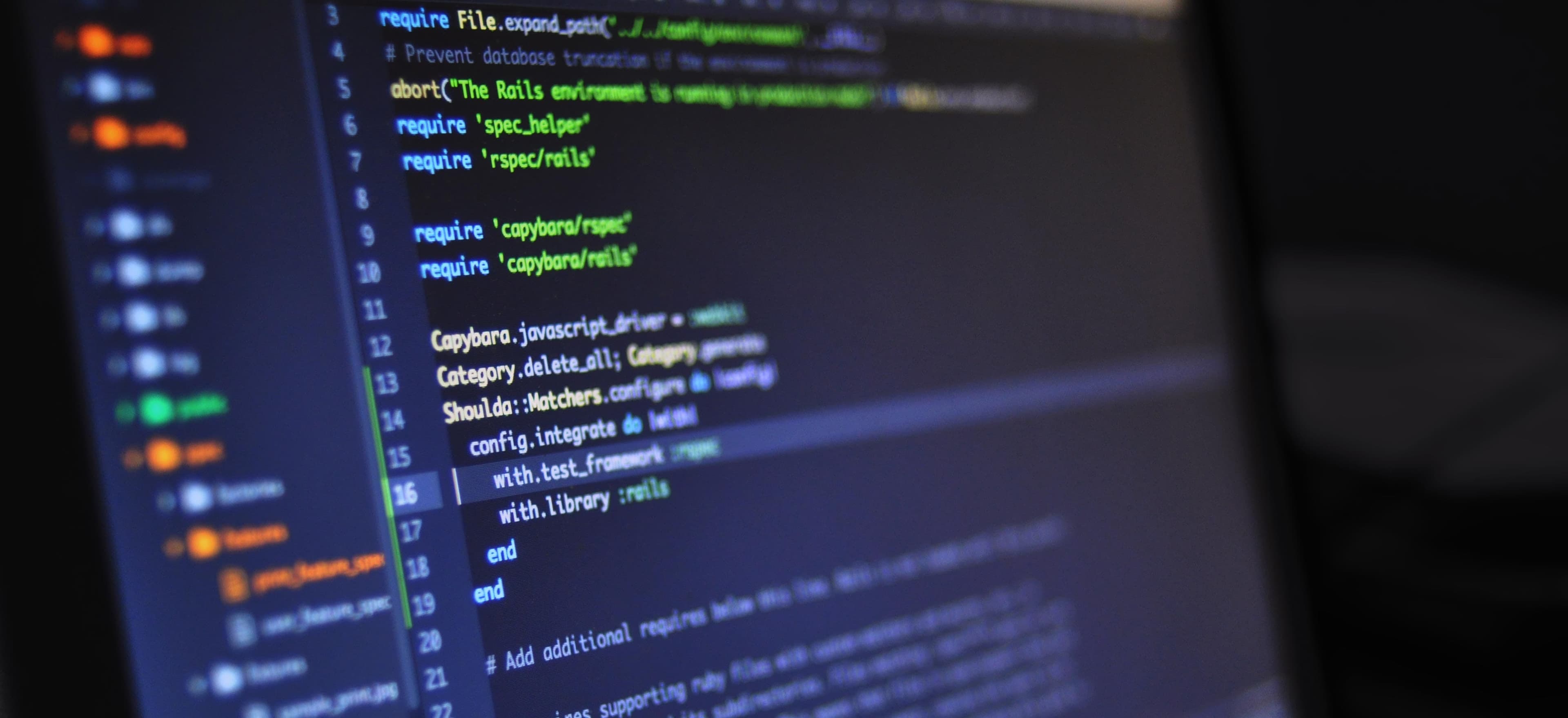
- Published on
Maximizing API Speed with Effective Caching
In today's fast-paced digital world, optimizing the performance of your Java applications is crucial. One of the key strategies for achieving this is through effective caching. By intelligently caching data, you can significantly improve the speed and responsiveness of your API, leading to a better user experience and overall system efficiency.
In this post, we will explore the concept of caching, its importance in API development, and how to implement effective caching strategies in Java to maximize API speed and performance.
Understanding Caching
What is Caching?
Caching is the process of storing frequently accessed data in a temporary storage area to reduce the need to retrieve it from the original source. In the context of API development, caching can be used to store the results of expensive operations, such as database queries or network requests, and serve them directly from the cache when the same operation is requested again.
Importance of Caching in API Development
Caching plays a crucial role in API development for several reasons:
-
Performance Improvement: By caching the results of commonly executed operations, API response times can be significantly reduced, leading to faster and more efficient interactions.
-
Scalability: Caching helps distribute the load on the server by serving frequently requested data from the cache, thereby reducing the overall server load and improving scalability.
-
Cost Reduction: Caching can lead to cost savings by reducing the consumption of resources, such as database connections and network bandwidth.
Implementing Effective Caching in Java
Now that we understand the importance of caching in API development, let's dive into how we can implement effective caching strategies in Java to maximize API speed and performance.
1. Using Caffeine for In-Memory Caching
Caffeine is a high-performance, near-optimal caching library for Java. It provides an in-memory cache implementation with a strong focus on performance and scalability.
Example: Setting up a Caffeine Cache
import com.github.benmanes.caffeine.cache.Caffeine;
Cache<String, Object> cache = Caffeine.newBuilder()
.maximumSize(10000)
.expireAfterWrite(10, TimeUnit.MINUTES)
.build();
In this example, we create a new Caffeine cache with a maximum size of 10,000 entries and an expiration time of 10 minutes for entries written to the cache.
Why is this effective?: Caffeine's advanced algorithms and data structures make it a highly efficient choice for in-memory caching in Java applications.
2. Integrating Redis for Distributed Caching
Redis is an open-source, in-memory data store that can be used as a distributed cache. It is exceptionally fast and supports various data structures, making it an ideal choice for caching in distributed systems.
Example: Using Jedis with Redis for Caching
JedisPool jedisPool = new JedisPool("redis://localhost:6379/0");
try (Jedis jedis = jedisPool.getResource()) {
// Perform caching operations using Jedis
jedis.set("key", "value");
String cachedValue = jedis.get("key");
}
Why is this effective?: By leveraging Redis as a distributed cache, we can offload the burden of caching from the application servers, leading to improved performance and scalability.
3. Utilizing Spring Caching for Method-Level Caching
Spring Framework provides built-in support for method-level caching through its @Cacheable
, @CachePut
, and @CacheEvict
annotations. This allows for easy integration of caching into Spring-based applications.
Example: Implementing Method-Level Caching with Spring
@Service
public class ProductService {
@Cacheable("products")
public Product getProductById(Long id) {
// Implementation to fetch product from the database
}
@CachePut("products")
public Product updateProduct(Product product) {
// Implementation to update product in the database
}
@CacheEvict(value = "products", allEntries = true)
public void refreshCache() {
// Implementation to clear the cache
}
}
Why is this effective?: Spring's method-level caching simplifies the process of integrating caching into the application logic, leading to more concise and maintainable code.
4. Leveraging HTTP Caching in API Responses
In addition to caching data at the server-side, leveraging HTTP caching mechanisms can also improve API performance. By setting appropriate cache control headers in API responses, we can enable client-side caching of the data.
Example: Setting Cache-Control Headers in API Responses
@GetMapping("/products/{id}")
public ResponseEntity<Product> getProductById(@PathVariable Long id) {
Product product = productService.getProductById(id);
return ResponseEntity.ok()
.cacheControl(CacheControl.maxAge(60, TimeUnit.SECONDS))
.body(product);
}
Why is this effective?: By leveraging HTTP caching, we can reduce the number of repeated API requests from clients, leading to faster response times and reduced server load.
Closing the Chapter
Effective caching is a powerful strategy for maximizing API speed and performance in Java applications. By intelligently caching data at various levels, including in-memory, distributed, method-level, and HTTP caching, we can significantly improve the speed, scalability, and efficiency of our APIs.
Optimizing API speed through effective caching is not just a technical improvement; it directly impacts user experience, customer retention, and overall system reliability. Therefore, investing time and effort into implementing robust caching strategies is a crucial aspect of modern API development.
By incorporating advanced caching techniques and utilising powerful caching libraries and tools such as Caffeine, Redis, and Spring Framework, Java developers can ensure that their APIs deliver optimal performance and responsiveness, meeting the demands of today's fast-paced digital landscape. Remember, when it comes to API speed, every millisecond counts.