Fixing Android List Scroll Lag
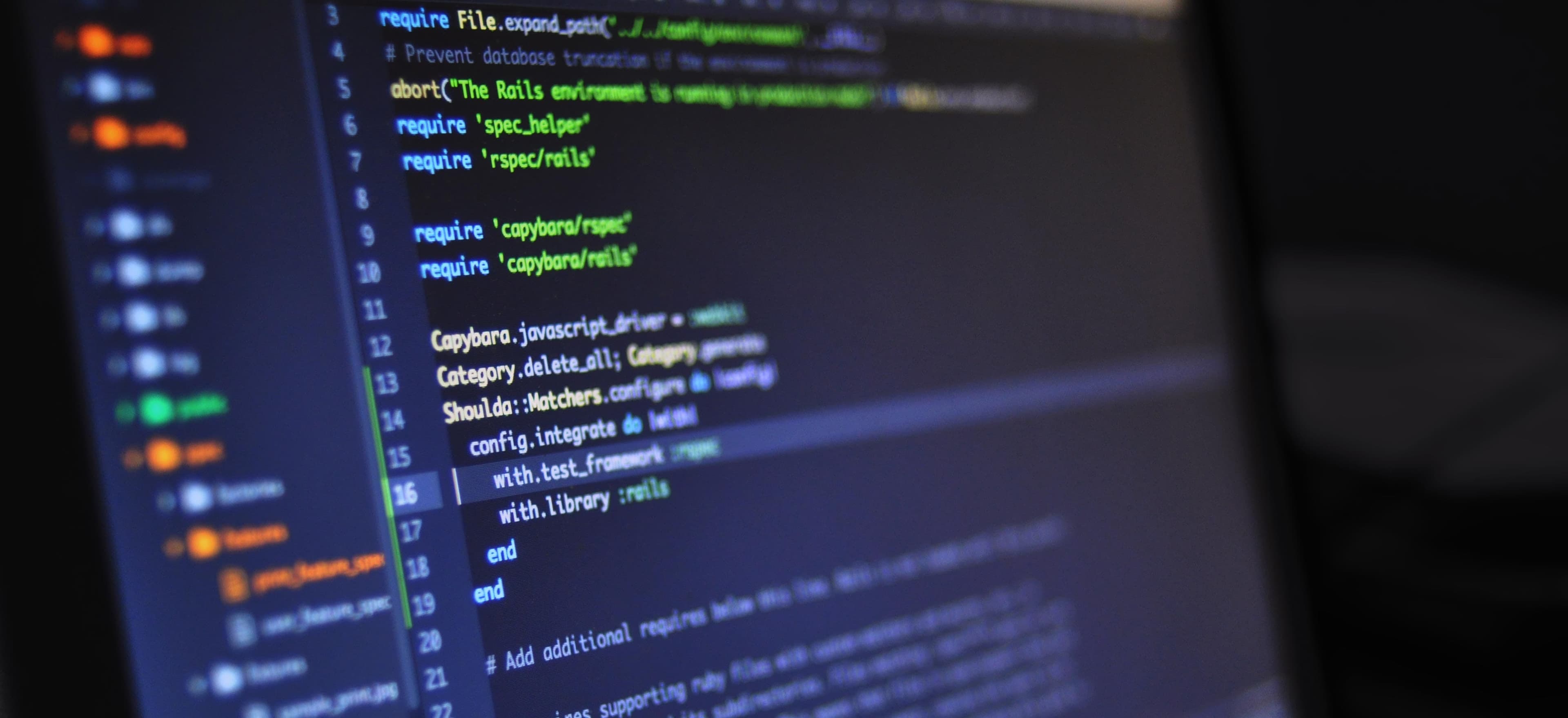
- Published on
Fixing Android List Scroll Lag
Are you experiencing scroll lag while using a list in your Android app? List scroll lag can be a frustrating issue for users and can negatively impact the overall user experience. In this post, we will explore some techniques to identify and fix list scroll lag in your Android app using Java.
Understanding the Problem
Before diving into the solutions, it's essential to understand the root cause of the list scroll lag. Scroll lag can occur due to various reasons such as inefficient use of data binding, complex view hierarchies, excessive layout calculations, or heavy UI updates during scrolling. Identifying the specific reason for scroll lag is crucial for applying the appropriate fix.
Using RecyclerView for Efficient List Rendering
One of the common causes of scroll lag in Android apps is the use of ListView
for displaying lists. ListView
tends to be less efficient compared to RecyclerView
, especially when dealing with large datasets. RecyclerView
provides better performance by recycling the views that are no longer visible, thus minimizing the memory overhead and layout inflation during scrolling.
Here's a basic RecyclerView
implementation in Java:
public class MyRecyclerViewAdapter extends RecyclerView.Adapter<MyRecyclerViewAdapter.ViewHolder> {
private ArrayList<String> mData;
// Constructor and ViewHolder implementation
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_layout, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
String item = mData.get(position);
holder.bind(item);
}
@Override
public int getItemCount() {
return mData.size();
}
// ViewHolder class
}
By switching from ListView
to RecyclerView
, you can significantly improve the scrolling performance of your lists.
Implementing Efficient Adapter Data Binding
Inefficient data binding within the adapter can also contribute to scroll lag. It's crucial to ensure that the data binding process within the adapter is optimized to minimize any unnecessary overhead during list scrolling.
Here's an example of efficient data binding within a RecyclerView
adapter:
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
String item = mData.get(position);
holder.textView.setText(item);
// Perform additional binding operations specific to your use case
}
Optimizing the data binding process can lead to smoother list scrolling by reducing the overhead associated with updating list item views.
Using ViewHolder Pattern for View Reusability
Utilizing the ViewHolder pattern in your RecyclerView
adapter can significantly improve the efficiency of view recycling during scrolling. The ViewHolder pattern caches the references to the subviews of the item view, eliminating the need to call findViewById
for every list item during scrolling.
Here's how to implement the ViewHolder pattern in a RecyclerView
adapter:
public class MyRecyclerViewAdapter extends RecyclerView.Adapter<MyRecyclerViewAdapter.ViewHolder> {
// Other adapter methods
public class ViewHolder extends RecyclerView.ViewHolder {
TextView textView;
public ViewHolder(View itemView) {
super(itemView);
textView = itemView.findViewById(R.id.text_view);
}
// Additional ViewHolder methods for binding data
}
}
By implementing the ViewHolder pattern, you can reduce the overhead of view initialization and improve the efficiency of view recycling, leading to smoother list scrolling.
Offloading Complex Operations from the Main Thread
Performing complex operations, such as heavy calculations or network calls, on the main thread can directly impact the responsiveness of list scrolling. It's essential to offload such operations from the main thread to background threads or asynchronous tasks to ensure that the main thread remains available for handling UI interactions.
Here's an example of offloading heavy operations using an AsyncTask:
private class DataLoadTask extends AsyncTask<Void, Void, ArrayList<String>> {
// Override doInBackground to perform heavy operations in the background
@Override
protected void onPostExecute(ArrayList<String> result) {
// Update the adapter with the result
}
}
By offloading heavy operations from the main thread, you can prevent UI thread congestion and ensure smoother list scrolling in your Android app.
Minimizing Layout Hierarchies and Complex Views
Complex view hierarchies and excessive nesting of layout elements can contribute to rendering bottlenecks, resulting in list scroll lag. It's crucial to minimize the layout hierarchies and simplify the view structures wherever possible to optimize the rendering performance.
Consider using ConstraintLayout
to flatten the view hierarchy and reduce layout complexity, resulting in improved rendering performance for lists.
Utilizing RecyclerView Item Decorations
RecyclerView item decorations offer a convenient way to add visual and structural elements to individual items in the list while maintaining efficient rendering during scrolling. By utilizing item decorations, you can enhance the visual appearance of list items without sacrificing the scroll performance.
Here's an example of implementing an item decoration for RecyclerView:
public class MyItemDecoration extends RecyclerView.ItemDecoration {
// Override methods to customize item decorations
}
Utilizing item decorations can add visual enhancements to your list items without compromising the scrolling performance of the RecyclerView.
Closing Remarks
Inefficient list scrolling can significantly impact the user experience of your Android app. By implementing the aforementioned techniques, such as utilizing RecyclerView
, optimizing data binding, offloading complex operations, and minimizing layout hierarchies, you can effectively mitigate scroll lag and ensure a smooth and responsive user interface for your app.
Remember, identifying the specific performance bottlenecks and applying targeted optimizations is essential for resolving list scroll lag effectively. Implementing these best practices will not only improve the scrolling performance of your lists but also contribute to the overall fluidity and responsiveness of your Android app.