Unlocking the Power of Eclipse Collections in 2019
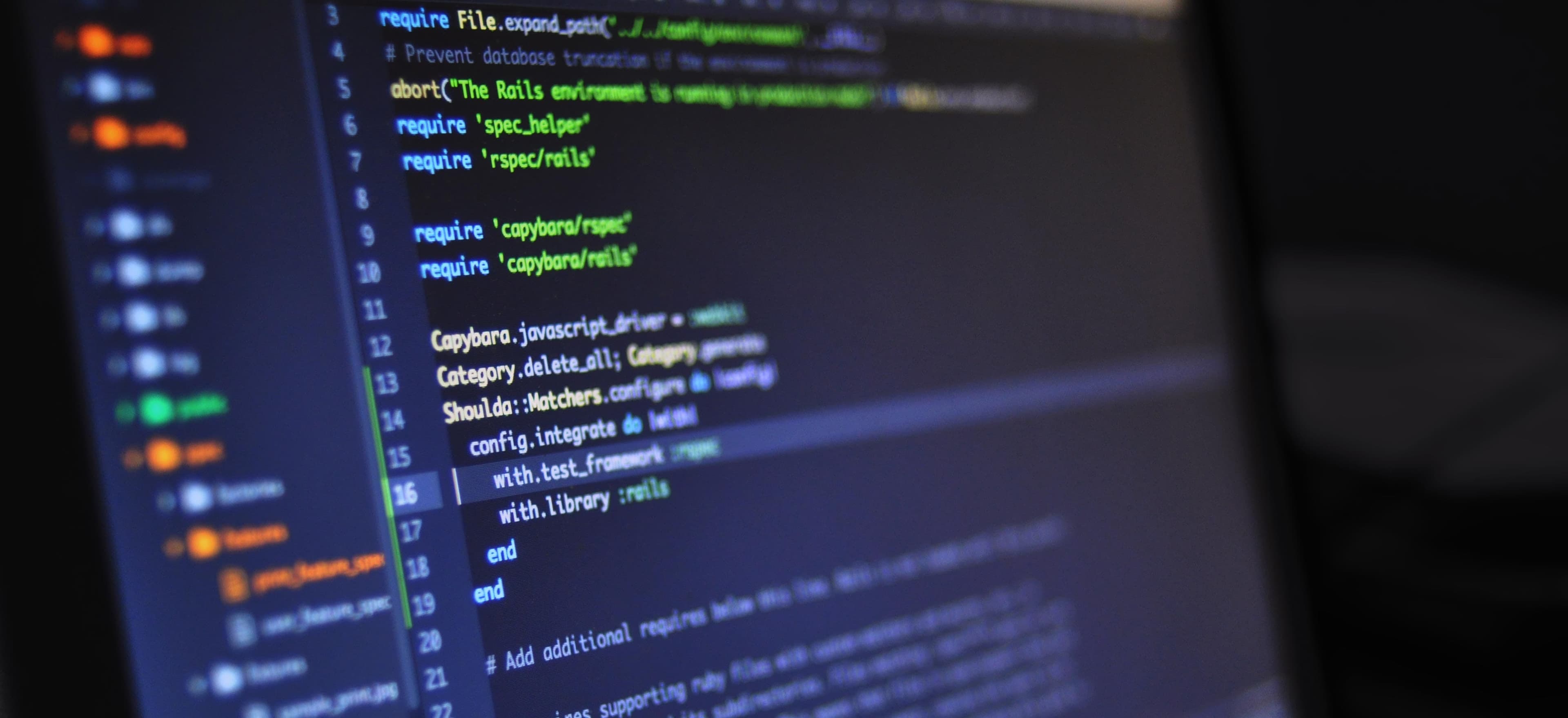
- Published on
Unlocking the Power of Eclipse Collections in 2019
In the world of Java programming, optimizing data structures and collections is a crucial aspect of writing high-performance code. Eclipse Collections is a Java library that provides a rich and expressive set of APIs for managing collections, surpassing the traditional Java Collections Framework in terms of both performance and readability. This blog post aims to shed light on the capabilities of Eclipse Collections and how it enables Java developers to write more efficient and elegant code.
What is Eclipse Collections?
Eclipse Collections is an open-source, high-performance collections framework for Java. It provides new types of collections and utilities that are not available in the standard Java Collections Framework, such as multimap, bag, and primitive collections. With a strong focus on functional programming and immutability, Eclipse Collections encourages developers to write cleaner, more concise code.
Features and Benefits
Enhanced Performance
One of the most compelling reasons to use Eclipse Collections is its superior performance compared to the standard Java Collections Framework. The library is optimized for speed and memory efficiency, making it an excellent choice for applications that demand high performance.
Fluent and Expressive APIs
Eclipse Collections offers fluent and expressive APIs that facilitate a more natural way of working with collections. The APIs enable developers to write code that is not only more readable but also more succinct, leading to increased productivity and maintainability.
Functional Programming Support
The library embraces functional programming paradigms, providing support for operations such as map, filter, and collect. This functional style of programming leads to less mutable state and more predictable code, ultimately resulting in fewer bugs and easier debugging.
Immutable Collections
Immutable collections are a key feature of Eclipse Collections. By default, the library encourages immutability, which helps in writing thread-safe code and avoiding unexpected side effects.
Additional Data Structures
Apart from the standard collection types available in Java, Eclipse Collections provides additional data structures such as Multimaps, Bags, and primitive collections. These additional data structures offer more versatility and flexibility in handling different use cases.
Getting Started with Eclipse Collections
Let’s dive into some practical examples to showcase the power and elegance of Eclipse Collections.
Creating Immutable Collections
ImmutableList<String> immutableList = Lists.immutable.of("apple", "banana", "cherry");
The above code snippet demonstrates how to create an immutable list using Eclipse Collections' factory methods. Immutable collections play a vital role in writing robust, thread-safe code and are an essential part of Eclipse Collections' core philosophy.
Transforming and Filtering Collections
MutableList<String> fruits = Lists.mutable.of("apple", "banana", "cherry", "date", "elderberry");
MutableList<String> uppercasedFruits = fruits.collect(String::toUpperCase);
MutableList<String> filteredFruits = fruits.select(s -> s.startsWith("a"));
In this example, we showcase the collect
and select
methods, which enable developers to transform and filter collections using a more functional approach. This leads to more readable and concise code, promoting better code maintainability.
Grouping and Counting with Multimaps
MutableListMultimap<Integer, String> lengthToWords =
Lists.mutable.of("apple", "banana", "cherry", "date", "elderberry")
.groupBy(String::length);
int countOfFiveLetterWords = lengthToWords.get(5).size();
Here, we utilize Eclipse Collections' groupBy
method to create a multimap that groups words based on their length. This demonstrates how Eclipse Collections simplifies tasks that would otherwise require multiple steps with standard Java collections.
Integration with Stream API
Eclipse Collections seamlessly integrates with the Java Stream API, allowing developers to leverage the benefits of both libraries in a unified manner. This integration provides a wider set of operations and enhances the overall functionality of working with collections in Java.
Migrating from Java Collections Framework
Migrating existing code to Eclipse Collections may seem daunting at first, but the benefits it offers make it a worthwhile pursuit. Consider using the Eclipse Collections Migration Tool, which automates a significant portion of the migration process, making it easier to transition existing codebases.
My Closing Thoughts on the Matter
The prowess of Eclipse Collections in providing a more efficient, readable, and performant approach to working with collections is undeniable. By embracing functional programming, immutability, and additional data structures, Eclipse Collections elevates the Java programming experience to a new level.
In conclusion, Java developers in 2019 should seriously consider adopting Eclipse Collections as their go-to library for handling collections, as it offers a substantial improvement over the standard Java Collections Framework.
Embrace the power of Eclipse Collections and unlock a new realm of possibilities in Java development!
To explore more about Eclipse Collections, refer to the official documentation and start integrating its capabilities into your Java projects.