Uncovering Actionable Insights from Data Science
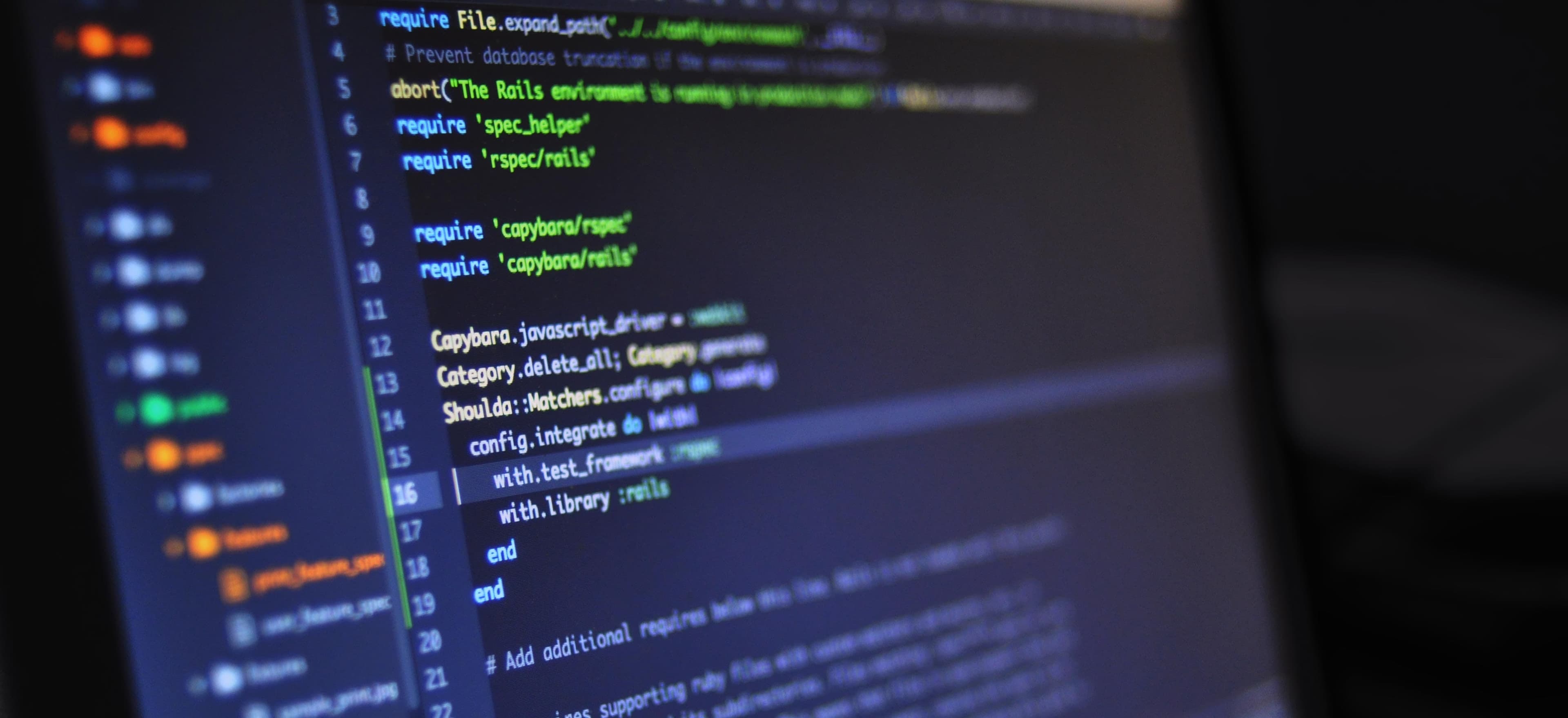
- Published on
Uncovering Actionable Insights from Data Science with Java
In the world of data science, Java might not be the first language that comes to mind. However, with its strong ecosystem, performance, and scalability, Java can be a powerful tool for data analysis and machine learning. In this blog post, we'll explore how Java can be used to uncover actionable insights from data.
Java for Data Analysis
Java might not have the same reputation for data analysis as Python or R, but it offers a wide range of libraries and tools for handling data. The most popular library for data analysis in Java is Apache Commons Math, which provides a wide range of mathematical algorithms and functions for data manipulation.
Example: Using Apache Commons Math for Data Analysis
import org.apache.commons.math3.stat.correlation.PearsonsCorrelation;
public class CorrelationExample {
public static void main(String[] args) {
double[] x = {1.0, 2.0, 3.0, 4.0, 5.0};
double[] y = {2.0, 3.0, 4.0, 5.0, 6.0};
PearsonsCorrelation correlation = new PearsonsCorrelation();
double result = correlation.correlation(x, y);
System.out.println("Pearson's correlation: " + result);
}
}
In this example, we use Apache Commons Math to calculate the Pearson's correlation coefficient between two arrays of numbers. This is just one example of how Java can be used for data analysis.
Java for Machine Learning
Java also has a growing ecosystem for machine learning. One of the most well-known libraries for machine learning in Java is Weka, which provides a wide range of algorithms for data mining and predictive modeling.
Example: Using Weka for Machine Learning
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
import weka.classifiers.functions.LinearRegression;
public class LinearRegressionExample {
public static void main(String[] args) throws Exception {
DataSource source = new DataSource("data.arff");
Instances dataset = source.getDataSet();
dataset.setClassIndex(dataset.numAttributes() - 1);
LinearRegression model = new LinearRegression();
model.buildClassifier(dataset);
System.out.println(model);
}
}
In this example, we use Weka to build a linear regression model from a dataset. This showcases how Java can be used for machine learning tasks.
Scalability and Performance
One of the key advantages of using Java for data analysis and machine learning is its scalability and performance. Java's ability to handle multithreading and its efficient memory management make it a strong contender for handling large datasets and complex machine learning models.
Integration with Big Data Technologies
Java seamlessly integrates with big data technologies such as Apache Hadoop and Apache Spark. This allows data scientists to leverage Java's strengths while working with distributed computing frameworks for handling massive amounts of data.
To Wrap Things Up
While Java might not be the most popular choice for data science and machine learning, it offers a robust ecosystem and powerful features for handling data and building machine learning models. With its scalability, performance, and integration with big data technologies, Java can be a valuable tool for uncovering actionable insights from data.
Start exploring the world of data science with Java and unleash the potential of data analysis and machine learning in your projects.
References:
- Apache Commons Math. Documentation
- Weka. Official Website
- Oracle. "Java Multithreading." Documentation
- Apache Hadoop. Official Website
- Apache Spark. Official Website