Maximizing Efficiency: Mastering javax.cache in Java
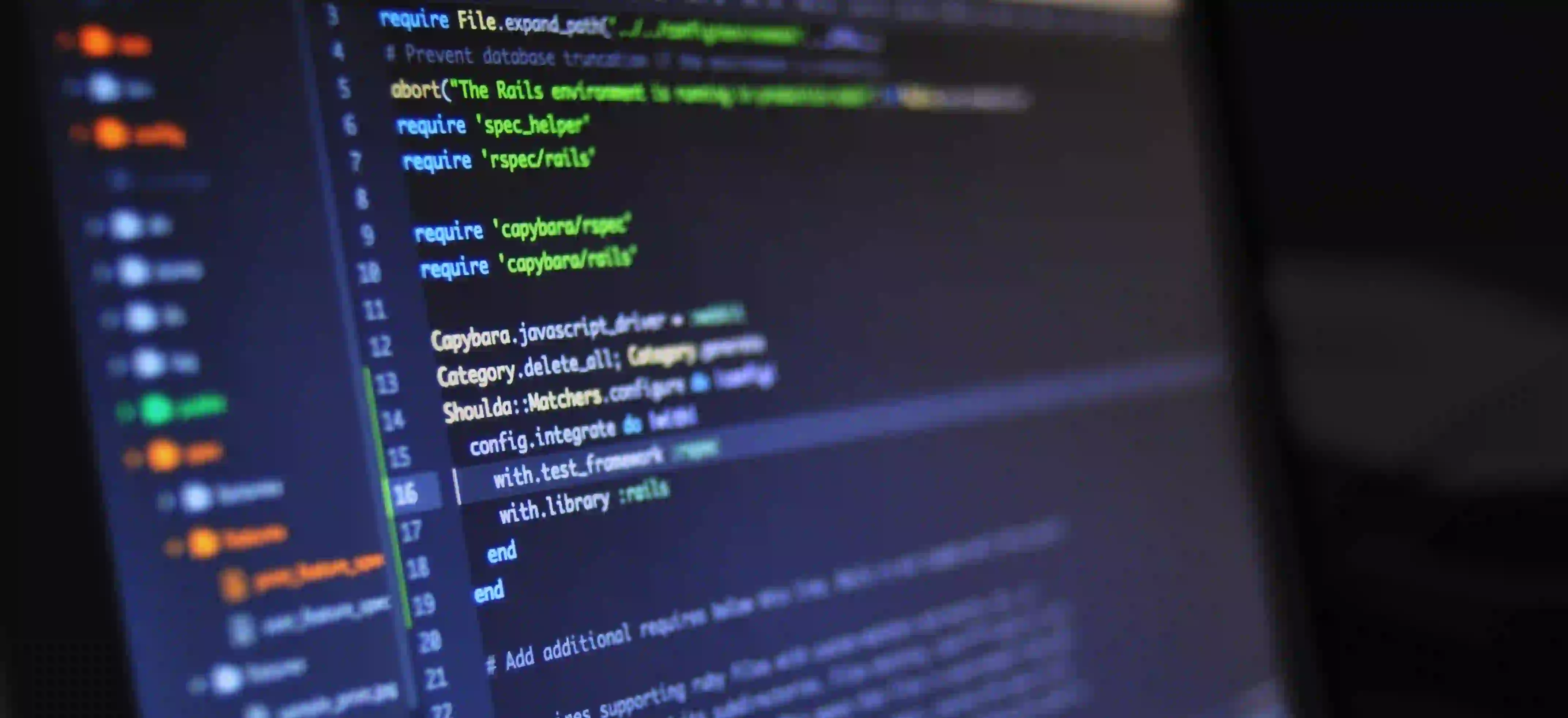
Maximizing Efficiency: Mastering javax.cache in Java
In the world of Java programming, efficiency is key. With the increasing volume of data and the need for fast and responsive applications, developers constantly seek ways to optimize their code and improve performance. One powerful tool that Java developers can leverage to achieve these goals is the javax.cache
API. In this blog post, we will explore the potential of javax.cache
and how it can be used to maximize efficiency in Java applications.
What is javax.cache?
The javax.cache
API, also known as JCache, is a standard caching API for Java that provides a common way for Java applications to interact with caching solutions. It is part of the Java Caching Standard (JSR-107) and is designed to be vendor agnostic, allowing developers to use it with various caching providers such as Ehcache, Hazelcast, and others.
Why use javax.cache?
Caching plays a crucial role in improving the performance of applications by reducing the response time and minimizing the load on backend resources. By using javax.cache
, developers can introduce caching into their applications in a standard and portable manner, without being tied to a specific caching implementation. This allows for greater flexibility and ease of switching between different caching providers if needed.
Getting started with javax.cache
To start using javax.cache
in your Java application, you first need to include the javax.cache
API and a caching provider as dependencies in your project. One popular caching provider is Ehcache, which can be easily integrated with the javax.cache
API.
<dependency>
<groupId>javax.cache</groupId>
<artifactId>cache-api</artifactId>
<version>1.1.1</version>
</dependency>
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
<version>3.9.4</version>
</dependency>
After adding the dependencies, you can start using javax.cache
to incorporate caching into your Java application.
Using javax.cache for efficient data retrieval
One of the primary use cases of caching is to improve the efficiency of data retrieval operations. Consider a scenario where an application needs to fetch data from a remote database. Without caching, this operation can be resource-intensive and may result in slower response times. By utilizing javax.cache
, the retrieved data can be stored in the cache, allowing subsequent requests for the same data to be served from the cache, thus reducing the load on the database and improving overall performance.
Let's take a look at an example of how you can use javax.cache
to cache the results of a database query using Ehcache as the caching provider.
CacheManager cacheManager = Caching.getCachingProvider().getCacheManager();
Cache<String, List<Data>> cache = cacheManager
.createCache("dataCache", new MutableConfiguration<>()
.setExpiryPolicyFactory(AccessedExpiryPolicy.factoryOf(Duration.ONE_HOUR))
.setStatisticsEnabled(true));
String query = "SELECT * FROM data";
List<Data> result = cache.get(query);
if (result == null) {
result = fetchDataFromDatabase(query);
cache.put(query, result);
}
In this example, a cache named "dataCache" is created, and it is used to store the results of the database query. The get
method is used to retrieve the data from the cache, and if it is not found, the data is fetched from the database and stored in the cache using the put
method.
By employing javax.cache
in this manner, developers can significantly reduce the overhead of data retrieval operations and enhance the responsiveness of their applications.
Handling cache eviction and expiration
Efficient cache management involves handling cache eviction and expiration to ensure that the cache remains effective and does not consume excessive memory. javax.cache
provides mechanisms to address these aspects of caching through its configuration options.
For instance, you can set a maximum size for the cache and define eviction policies to remove less frequently accessed data when the cache reaches its capacity. Additionally, you can specify expiration policies to automatically remove cached entries after a certain period.
Cache<String, Data> cache = cacheManager.createCache("dataCache",
new MutableConfiguration<>()
.setExpiryPolicyFactory(ModifiedExpiryPolicy.factoryOf(Duration.ONE_HOUR))
.setStatisticsEnabled(true)
.setManagementEnabled(true)
.setSizeOfMaxObjectGraph(1000)
.setEvictionPolicy(Eviction.createLRU(1000)));
In this code snippet, we create a cache with eviction policies and expiration settings. The ModifiedExpiryPolicy
is used to expire entries after an hour, and the cache is configured with a maximum size and LRU (Least Recently Used) eviction policy.
By proactively managing cache eviction and expiration, developers can ensure that the cache remains efficient and does not become a bottleneck for the application.
Integrating javax.cache with Spring Framework
For developers using the Spring Framework, integrating javax.cache
into their applications is straightforward. Spring provides support for JSR-107 annotations, allowing developers to easily incorporate caching with minimal configuration.
@Cacheable(value = "dataCache", key = "#query")
public List<Data> fetchDataFromDatabase(String query) {
// Database query logic
return data;
}
In this example, the @Cacheable
annotation is used to indicate that the fetchDataFromDatabase
method's return value should be cached in the "dataCache" cache with the query string as the key. Spring takes care of caching the method's return value based on the specified cache configuration.
By seamlessly integrating javax.cache
with the Spring Framework, developers can leverage caching to enhance the performance of their applications with minimal effort.
Closing Remarks
Efficiency is a critical aspect of Java application development, and leveraging caching is a powerful technique to optimize performance. The javax.cache
API provides a standardized approach to incorporating caching into Java applications, offering portability and flexibility across different caching providers.
By utilizing javax.cache
for efficient data retrieval, managing cache eviction and expiration, and seamlessly integrating with frameworks such as Spring, developers can maximize the efficiency and responsiveness of their Java applications.
In conclusion, mastering javax.cache
empowers Java developers to take control of application performance and deliver exceptional user experiences through optimized data access and responsiveness.
Embrace the power of javax.cache
to unlock the full potential of caching in your Java applications and stay ahead in the quest for maximum efficiency and performance.
In this article, we delved into the world of javax.cache
in Java, exploring its potential to maximize efficiency in Java applications. We learned about the benefits of using javax.cache
, how to get started with it, and various strategies to implement efficient caching. Whether you are a seasoned Java developer or just starting your journey, incorporating javax.cache
into your arsenal can significantly enhance the performance of your applications.
By optimizing data retrieval, managing cache eviction and expiration, and seamlessly integrating with popular frameworks like Spring, javax.cache
proves to be a valuable asset in the pursuit of efficiency. So, why wait? Dive into javax.cache
and elevate your Java applications to new heights of performance and responsiveness.