Effective Unit Testing Best Practices
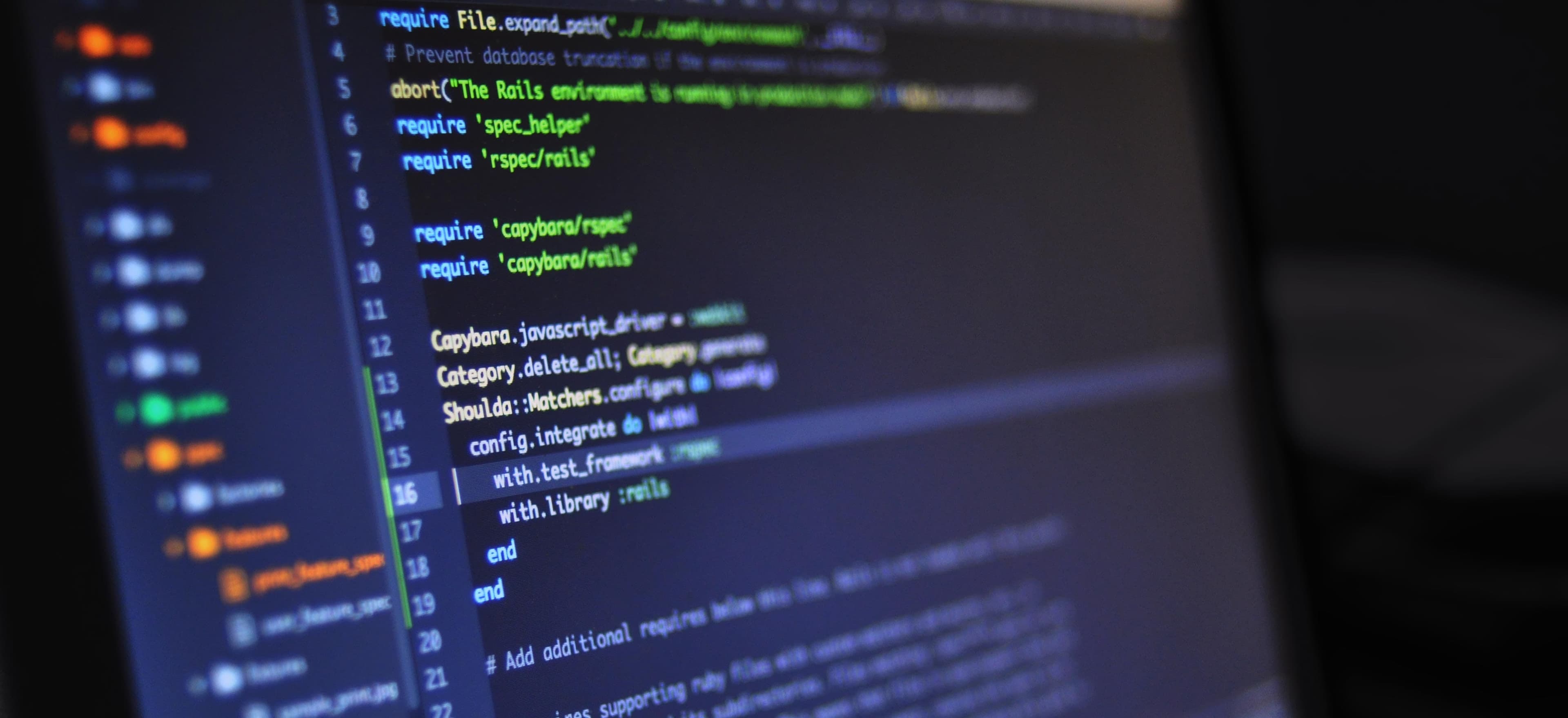
- Published on
Effective Unit Testing Best Practices
Unit testing is a crucial aspect of software development. It helps in identifying and fixing bugs early in the development process, ensures that code behaves as expected, and provides a safety net for code refactoring. In this blog post, we will discuss the best practices for writing effective unit tests in Java.
1. Test One Thing at a Time
Each unit test should focus on testing a single piece of functionality. This helps in keeping the tests simple, clear, and easy to maintain. It also makes it easier to identify the cause of a test failure.
@Test
public void calculateTotalPrice_whenNoDiscountApplied_shouldReturnTotalPrice() {
// Test logic for calculating total price without applying any discount
}
2. Use Descriptive and Clear Test Names
A descriptive test name helps in understanding what is being tested without looking at the test code. It also serves as documentation for the intended behavior of the code being tested.
@Test
public void calculateTotalPrice_whenNoDiscountApplied_shouldReturnTotalPrice() {
// Test logic for calculating total price without applying any discount
}
3. Follow the AAA Pattern (Arrange, Act, Assert)
Arrange the prerequisites for the test, perform the action being tested, and assert the expected behavior. This pattern organizes the test code and makes it easy to understand the flow of the test.
@Test
public void calculateTotalPrice_whenNoDiscountApplied_shouldReturnTotalPrice() {
// Arrange
ShoppingCart cart = new ShoppingCart();
cart.addItem(new Item("Product 1", 100));
// Act
double totalPrice = cart.calculateTotalPrice();
// Assert
assertEquals(100, totalPrice, 0.001);
}
4. Limit Test Scope
Avoid testing implementation details that are likely to change. Focus on testing the public API of the code to ensure that the behavior is maintained while allowing for changes to the internal implementation.
5. Use Parameterized Tests for Data-Driven Testing
Parameterized tests allow running the same test with different inputs. This is useful for testing different scenarios with varying input values.
@ParameterizedTest
@CsvSource({
"100, 0, 100",
"100, 10, 90"
})
public void applyDiscount_shouldReturnDiscountedPrice(int price, int discount, int expectedPrice) {
// Test logic for applying discount to the price
}
6. Mock External Dependencies
Use mocking frameworks like Mockito to create mock objects for external dependencies. This allows isolating the code under test and focusing on its behavior without involving the actual external dependencies.
@Test
public void processOrder_shouldUpdateInventory() {
// Arrange
InventoryService inventoryService = Mockito.mock(InventoryService.class);
Order order = new Order(/*...*/);
OrderProcessor orderProcessor = new OrderProcessor(inventoryService);
// Act
orderProcessor.processOrder(order);
// Assert
Mockito.verify(inventoryService, Mockito.times(1)).updateInventory(order);
}
7. TDD (Test-Driven Development)
Adopt the practice of writing tests before writing the actual code. This ensures that the code is testable and that the tests cover the intended behavior.
8. Use Code Coverage Tools
Utilize code coverage tools like JaCoCo to measure how much of the code is covered by the unit tests. Aim for high code coverage to ensure that most of the code paths are tested.
9. Run Tests Automatically
Integrate unit tests with build processes using tools like Maven or Gradle. Set up continuous integration to run tests automatically on every code change.
10. Refactor Tests Along with Production Code
As the production code evolves, refactor the tests to maintain their readability and effectiveness. Keep the tests clean and maintainable.
In conclusion, effective unit testing in Java plays a crucial role in delivering high-quality software. By following these best practices, developers can ensure that their unit tests are clear, maintainable, and provide valuable feedback on the behavior of the code under test.
Remember, writing effective unit tests is a skill that improves with practice and experience. By incorporating these best practices into your workflow, you can elevate the quality of your code and streamline the development process.
For further reading on unit testing in Java, check out JUnit's official documentation and Mockito's official site.