Managing Complexity in Nested Classes
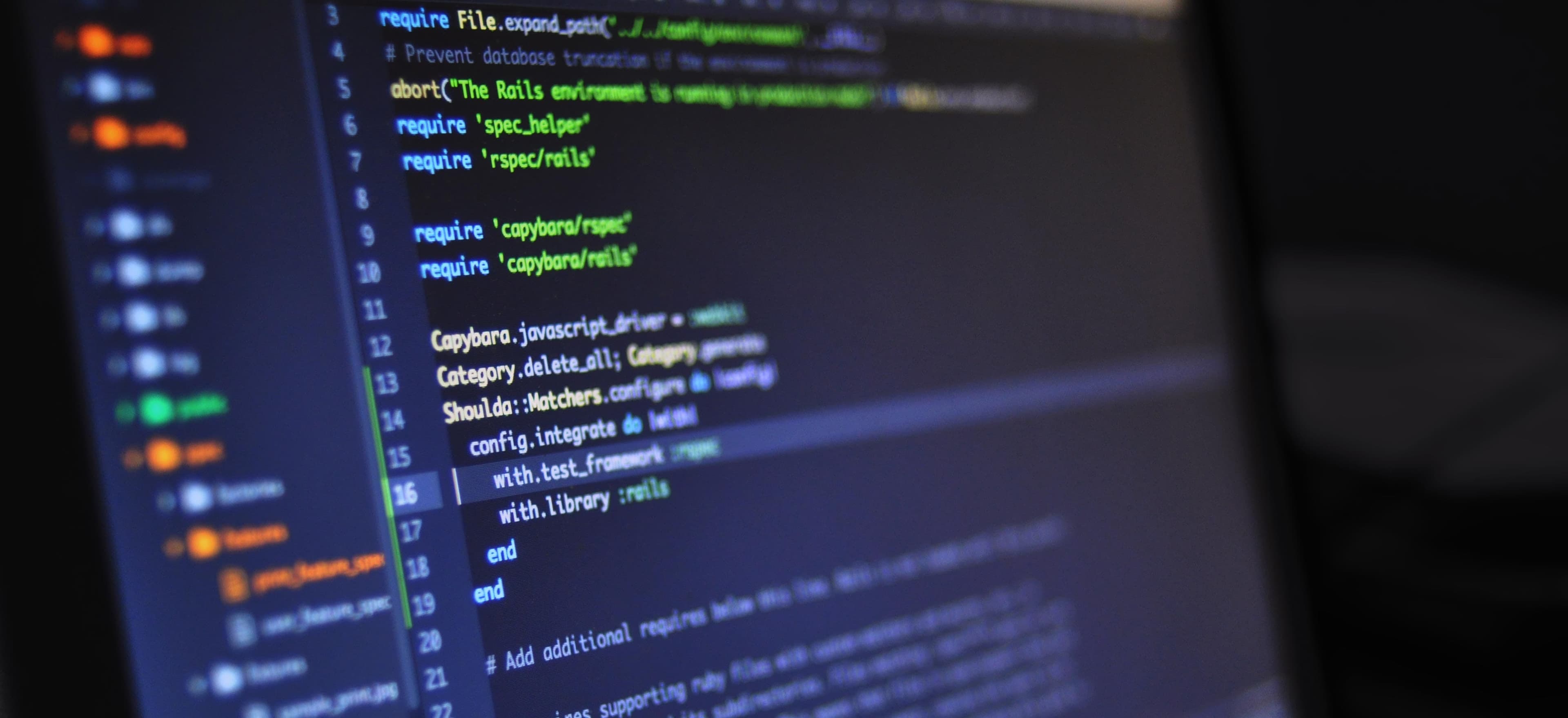
- Published on
When developing a Java application, it's essential to maintain clean, organized, and maintainable code. One area that can quickly become complex is dealing with nested classes. While nesting classes can sometimes be a convenient way to encapsulate functionality, improper use can lead to code that's difficult to read, understand, and maintain. In this blog post, we'll explore best practices for managing complexity in nested classes in Java, and how to use them effectively to improve code readability and maintainability.
Understanding Nested Classes
In Java, a nested class is a class defined within another class. There are four types of nested classes: static nested classes, non-static nested classes (also known as inner classes), local classes, and anonymous classes. Each type has its own use cases and implications for code complexity.
Best Practices for Using Nested Classes
1. Use Static Nested Classes for Encapsulation
Static nested classes are used to logically group classes that are only used in one outer class. They can increase encapsulation, as they can be hidden from the outside world, and do not have access to instance variables of the outer class. This can reduce complexity by clearly defining the scope of the nested class.
public class Outer {
private static class Nested {
// ...
}
}
2. Limit the Scope of Inner Classes
Inner classes have access to the instance variables of the outer class, which can lead to increased complexity and coupling. Limit the use of inner classes to cases where they are tightly coupled with the outer class and are not intended to be used outside of it.
public class Outer {
private class Inner {
// ...
}
}
3. Use Local Classes Sparingly
Local classes are classes defined within a method. They should be used sparingly, as they can make code harder to understand and maintain due to their close proximity to the logic they are used in. Consider using them only when a class is needed for a small, specific portion of code.
public class Outer {
public void doSomething() {
class Local {
// ...
}
}
}
4. Prefer Lambda Expressions over Anonymous Classes
Anonymous classes can significantly increase complexity, especially when used for event handling or small tasks. Consider using lambda expressions instead, as they are more concise and can lead to cleaner, more readable code.
Managing Complexity in Nested Classes
When dealing with nested classes, it's important to prioritize code readability and maintainability. Here are some strategies for managing complexity in nested classes:
1. Keep Nested Classes Short and Focused
Just like any other class, nested classes should have a single responsibility and be kept short and focused. Splitting a large nested class into smaller, more focused classes can reduce complexity and improve readability.
2. Avoid Deep Nesting
Avoid deeply nested classes, as they can quickly become difficult to understand and maintain. Consider refactoring deeply nested classes into separate top-level classes or using static nested classes to flatten the structure.
3. Use Descriptive Names
Naming nested classes descriptively can help in understanding their purpose and usage. A clear and concise name can make the intent of the nested class apparent without needing to delve into its implementation details.
4. Document the Purpose and Usage
Provide clear documentation for nested classes, especially when they are complex or have intricate relationships with the outer class. Comments and Javadoc can help other developers understand the role and usage of nested classes without needing to decipher the code.
Final Considerations
Nested classes can be a powerful tool for organizing code and improving encapsulation. However, if used improperly, they can lead to increased complexity and decreased code maintainability. By following best practices and strategies for managing complexity, you can effectively use nested classes to write clean, readable, and maintainable Java code.
By understanding the type of nested class that best fits your use case and implementing strategies to manage complexity, you can harness the power of nested classes without compromising the readability and maintainability of your codebase. Whether you're working with static nested classes, inner classes, local classes, or anonymous classes, keeping these best practices in mind will help you write better Java code.
Start implementing these best practices in your Java projects to improve code quality and make your codebase more maintainable. Happy coding!
For more in-depth information, visit Oracle's official documentation on nested classes.
Remember, clean and organized code is key to a maintainable application. Keep learning, keep improving!