Mastering Functional Interfaces in Java 8
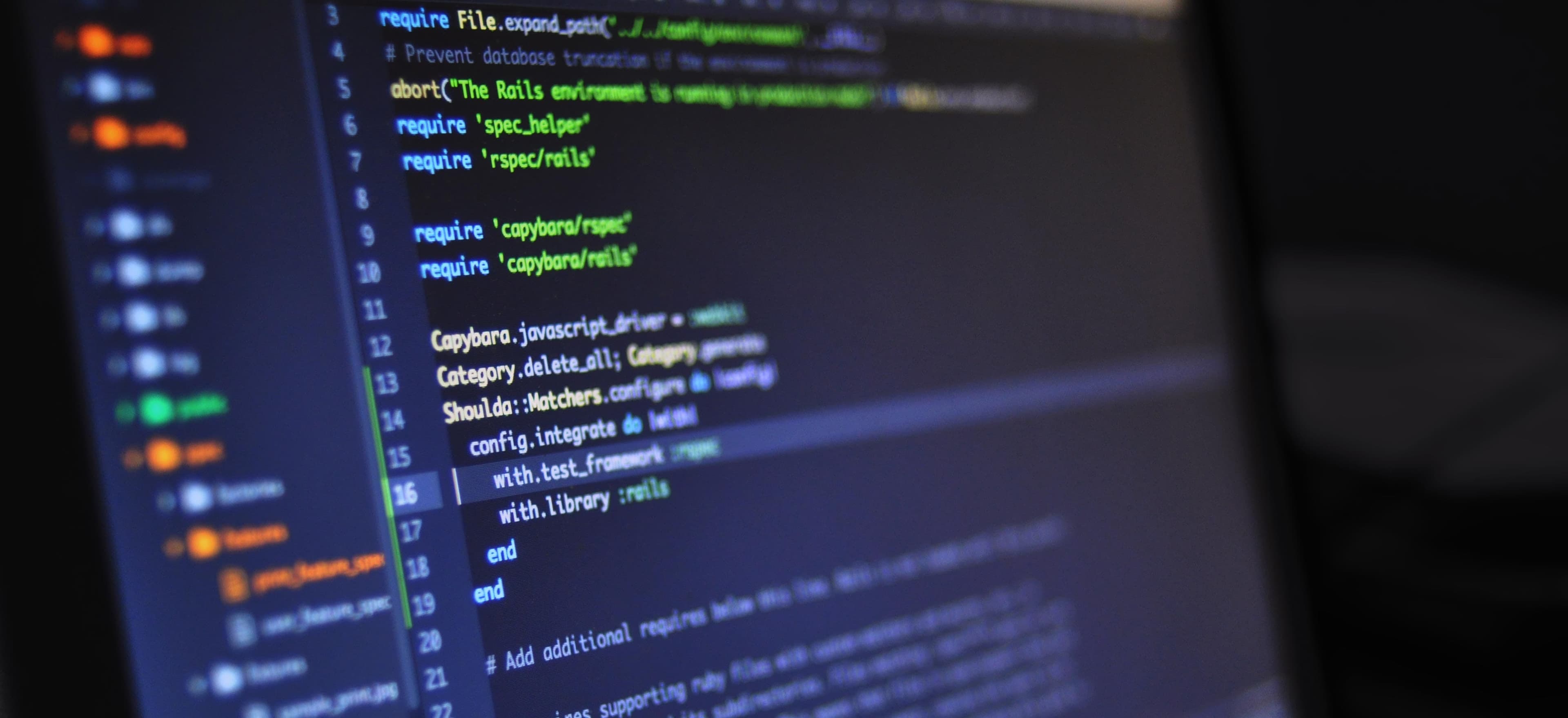
- Published on
Mastering Functional Interfaces in Java 8
Java 8 has revolutionized the way developers write code with the introduction of functional interfaces. This blog post will explore the concepts of functional interfaces, lambda expressions, and how they are used to write more concise and expressive code in Java.
Understanding Functional Interfaces
In Java, a functional interface is an interface that contains only one abstract method. This single method forms the basis of the interface's functionality. Although a functional interface can have default methods or static methods, it must have only one abstract method to be classified as a functional interface.
Let's create a basic functional interface to understand its structure:
@FunctionalInterface
interface MyFunctionalInterface {
void myMethod();
}
We use the @FunctionalInterface
annotation to ensure that the interface meets the criteria of a functional interface. This annotation is optional, but it can help prevent accidental addition of abstract methods in the future.
Introducing Lambda Expressions
Lambda expressions were introduced in Java 8 to provide a concise way to represent an anonymous function. In the context of functional interfaces, lambda expressions can be used to provide the implementation of the single abstract method defined in the interface.
Consider the following example where we use a lambda expression to implement the MyFunctionalInterface
we defined earlier:
public class Main {
public static void main(String[] args) {
MyFunctionalInterface myLambda = () -> System.out.println("Executing myMethod");
myLambda.myMethod();
}
}
In this example, the lambda expression () -> System.out.println("Executing myMethod")
serves as the implementation of the myMethod
defined in the MyFunctionalInterface
. This concise syntax makes the code more readable and eliminates the need for verbose anonymous inner classes.
Functional Interface Utility: java.util.function Package
Java 8 provides a set of functional interfaces in the java.util.function
package to cover common functional shapes such as predicates, functions, consumers, and suppliers. These interfaces are essential in functional programming and are heavily used in streams and lambda expressions.
Let's explore some of the key functional interfaces in the java.util.function
package:
1. Predicate
The Predicate
interface represents a predicate (boolean-valued function) of one argument. It is often used for filtering elements in a collection or stream.
@FunctionalInterface
interface Predicate<T> {
boolean test(T t);
}
The test
method accepts an argument and returns true
or false
based on the predicate's evaluation.
2. Function
The Function
interface represents a function that accepts one argument and produces a result. It is used to transform data in a functional manner.
@FunctionalInterface
interface Function<T, R> {
R apply(T t);
}
The apply
method accepts an argument of type T
and returns a result of type R
.
3. Consumer
The Consumer
interface represents an operation that accepts a single input argument and returns no result. It is often used to perform operations such as printing, updating, or processing data.
@FunctionalInterface
interface Consumer<T> {
void accept(T t);
}
The accept
method performs the operation on the given input.
4. Supplier
The Supplier
interface represents a supplier of results. It has no input arguments and provides a result upon invocation.
@FunctionalInterface
interface Supplier<T> {
T get();
}
The get
method returns a result of type T
.
Method References
In addition to lambda expressions, Java 8 introduces method references, which provide a way to refer to methods or constructors without invoking them. Method references are often used when a lambda expression just calls an existing method.
There are four types of method references:
- Reference to a static method
- Reference to an instance method of a particular object
- Reference to an instance method of an arbitrary object of a particular type
- Reference to a constructor
Let's illustrate the concept of method references using a simple example:
import java.util.List;
public class Main {
public static void main(String[] args) {
List<String> names = List.of("Alice", "Bob", "Charlie");
names.forEach(System.out::println);
}
}
In this example, System.out::println
is a method reference that refers to the println
method of the out
object in the System
class. This concise syntax enhances code readability and reduces the verbosity of lambda expressions.
Default Methods and Static Methods in Functional Interfaces
In Java 8, functional interfaces can have default methods and static methods without affecting their status as functional interfaces. This enables us to provide default implementations or utility methods within the functional interface itself.
Let's enhance our MyFunctionalInterface
by adding a default method:
@FunctionalInterface
interface MyFunctionalInterface {
void myMethod();
default void myDefaultMethod() {
System.out.println("Executing default method");
}
}
Now, the MyFunctionalInterface
contains both an abstract method (myMethod
) and a default method (myDefaultMethod
). The presence of the default method does not violate the rules of being a functional interface.
Bringing It All Together
In conclusion, mastering functional interfaces in Java 8 opens up new possibilities for writing clear, expressive, and concise code. Understanding how to use functional interfaces, lambda expressions, and the utility of the java.util.function
package is crucial for taking advantage of functional programming features in Java.
By leveraging lambda expressions, method references, and functional interfaces, developers can write more elegant and maintainable code, while also embracing the functional programming paradigm in Java.
To further explore the topic of functional programming in Java, check out the official Java documentation and the book "Java 8 in Action" by Raoul-Gabriel Urma, Mario Fusco, and Alan Mycroft.
Start mastering functional interfaces in Java 8 today and elevate your coding skills to the next level!