Streamlining Blue-Green Deployments on Amazon EC2
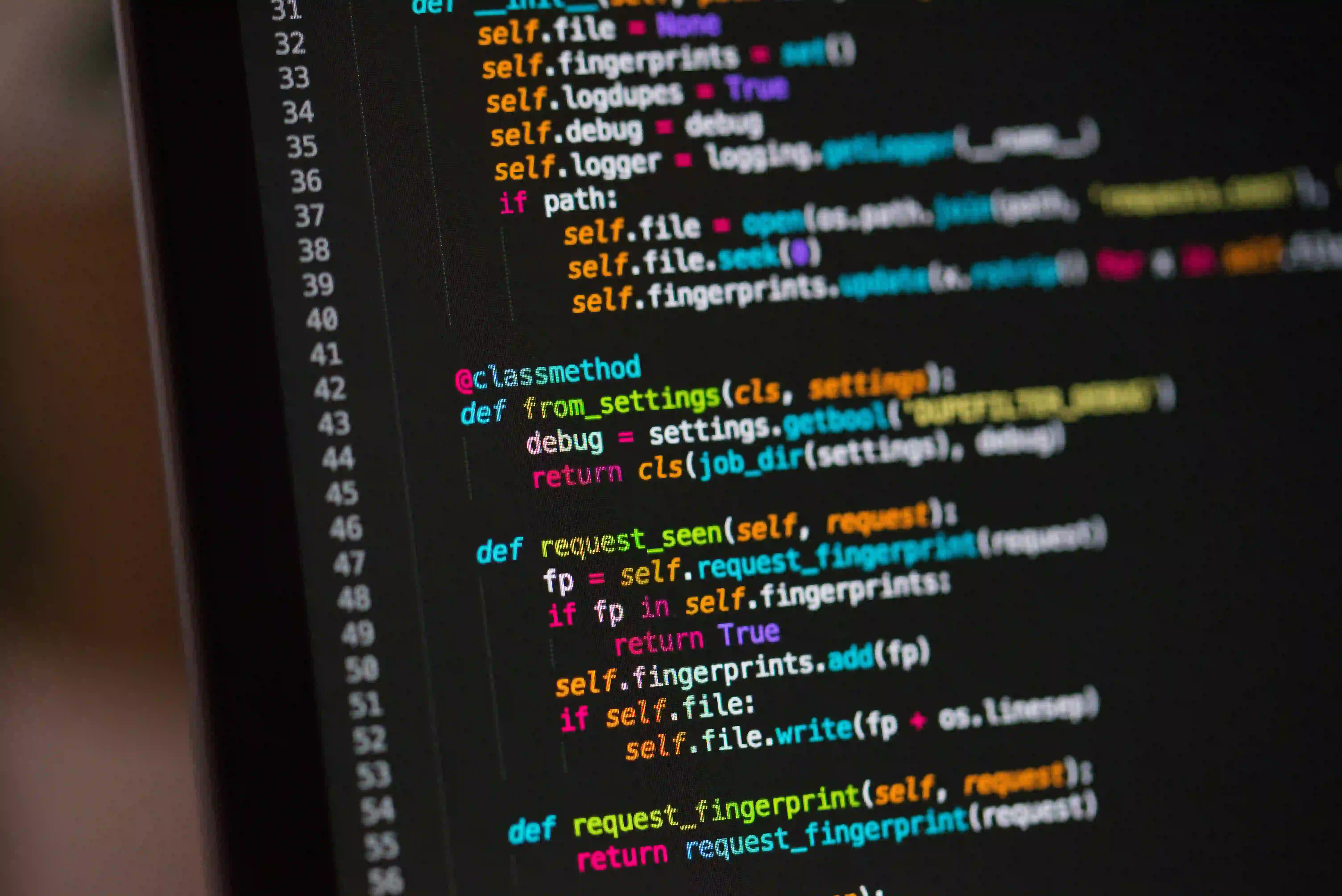
Blue-green deployments are a popular technique used to minimize downtime and mitigate risks during software releases. This approach involves running two identical production environments, where one is live (blue) and the other is idle (green). The idle environment can be used to test and stage the new version of the software, and when ready, the traffic is switched to it. In this blog post, we'll explore how to streamline blue-green deployments on Amazon EC2 using Java and best practices.
Why Blue-Green Deployments?
A blue-green deployment ensures minimal downtime when rolling out updates or testing new features. By having two identical environments, continuous delivery and continuous integration are simplified – allowing a seamless transition from the old environment to the new one without disrupting the end users.
Blue-green deployments also provide a quick and reliable rollback mechanism. If issues arise with the new deployment, it's easy to switch back to the previous environment, keeping the system running with minimal impact on users.
Setting Up the Environment
Before diving into the deployment process, it's essential to provision two identical environments. In this example, we'll use Amazon EC2 instances for our blue-green deployment.
Using AWS SDK for Java
The AWS SDK for Java simplifies working with Amazon EC2 instances. It provides APIs for launching, terminating, and managing instances. Let's take a look at how to use the AWS SDK for Java to set up the initial environments.
// Create an AmazonEC2 client
AmazonEC2 ec2 = AmazonEC2Client.builder().withRegion("us-west-2").build();
// Launch a new EC2 instance (blue)
RunInstancesRequest runRequest = new RunInstancesRequest()
.withImageId("ami-1234abcd")
.withInstanceType("t2.micro")
.withMinCount(1)
.withMaxCount(1);
RunInstancesResult result = ec2.runInstances(runRequest);
// Create a tag for the instance
CreateTagsRequest tagRequest = new CreateTagsRequest()
.withResources(result.getReservation().getInstances().get(0).getInstanceId())
.withTags(new Tag("Name", "blue-instance"));
ec2.createTags(tagRequest);
In this example, we're using the AWS SDK for Java to launch an EC2 instance with the specified Amazon Machine Image (AMI) and instance type, and then creating a tag to identify it as the blue environment.
Zero Downtime Deployment with Elastic Load Balancing
Elastic Load Balancing (ELB) plays a crucial role in blue-green deployments by enabling zero downtime deployment. By configuring ELB to distribute traffic across the blue and green environments, we can seamlessly switch traffic from one environment to the other with no impact on end users.
Managing ELB with Java
Using the AWS SDK for Java, we can manage ELB resources, including setting up and configuring load balancers. Let's see how to create an ELB and register instances with it.
// Create an Elastic Load Balancing client
AmazonElasticLoadBalancing elb = AmazonElasticLoadBalancingClient.builder().withRegion("us-west-2").build();
// Create a load balancer
CreateLoadBalancerRequest lbRequest = new CreateLoadBalancerRequest()
.withLoadBalancerName("my-load-balancer")
.withListeners(new Listener("HTTP", 80, 80))
.withAvailabilityZones("us-west-2a");
CreateLoadBalancerResult lbResult = elb.createLoadBalancer(lbRequest);
// Register instances with the load balancer
RegisterInstancesWithLoadBalancerRequest registerRequest = new RegisterInstancesWithLoadBalancerRequest()
.withLoadBalancerName("my-load-balancer")
.withInstances(new Instance("blue-instance"));
elb.registerInstancesWithLoadBalancer(registerRequest);
In this code snippet, we're using the AWS SDK for Java to create a load balancer and register the blue environment instance with it. It's important to note that the green environment instance should be launched and registered in the same way.
Automating the Deployment Process
To streamline the deployment process, automation tools like AWS CodeDeploy can be utilized. AWS CodeDeploy allows developers to automate the deployment of applications to Amazon EC2 instances, and it integrates seamlessly with blue-green deployments.
Integrating AWS CodeDeploy with Java
Using the AWS CodeDeploy SDK for Java, developers can automate the deployment process, enabling consistent, repeatable deployments with minimal human intervention.
// Create an AWS CodeDeploy client
AmazonCodeDeploy codeDeploy = AmazonCodeDeployClient.builder().withRegion("us-west-2").build();
// Create a deployment group
CreateDeploymentGroupRequest deploymentGroupRequest = new CreateDeploymentGroupRequest()
.withApplicationName("my-application")
.withDeploymentGroupName("my-deployment-group")
.withEc2TagSet(new EC2TagFilter().withKey("Name").withValue("blue-instance"))
.withServiceRoleArn("arn:aws:iam::123456789012:role/my-codedeploy-role");
CreateDeploymentGroupResult deploymentGroupResult = codeDeploy.createDeploymentGroup(deploymentGroupRequest);
// Create a deployment
CreateDeploymentRequest deploymentRequest = new CreateDeploymentRequest()
.withApplicationName("my-application")
.withDeploymentGroupName("my-deployment-group")
.withRevision(new RevisionLocation().withRevisionType(RevisionLocationType.S3)
.withS3Location(new S3Location().withBundleType(BundleType.TAR)
.withBucket("my-bucket")
.withKey("my-app.zip")));
CreateDeploymentResult deploymentResult = codeDeploy.createDeployment(deploymentRequest);
In this example, we're using the AWS CodeDeploy SDK for Java to create a deployment group and initiate a deployment. The SDK provides a convenient way to automate the deployment process, reducing the risk of errors and ensuring consistency across deployments.
My Closing Thoughts on the Matter
In conclusion, blue-green deployments on Amazon EC2 can be streamlined using Java and various AWS SDKs. By leveraging the AWS SDK for Java, developers can effectively manage EC2 instances, Elastic Load Balancing, and AWS CodeDeploy, automating the entire deployment process.
Implementing blue-green deployments not only minimizes downtime and reduces the risk of deployment failures but also provides a reliable and straightforward way to roll back changes if necessary.
By following best practices and utilizing the AWS SDK for Java, developers can ensure a seamless and efficient deployment process, ultimately providing a better experience for end users while maintaining system stability and reliability.
For further reading on blue-green deployments, refer to the AWS documentation.