Troubleshooting Connectivity Issues with Hawt.io and Apache jclouds
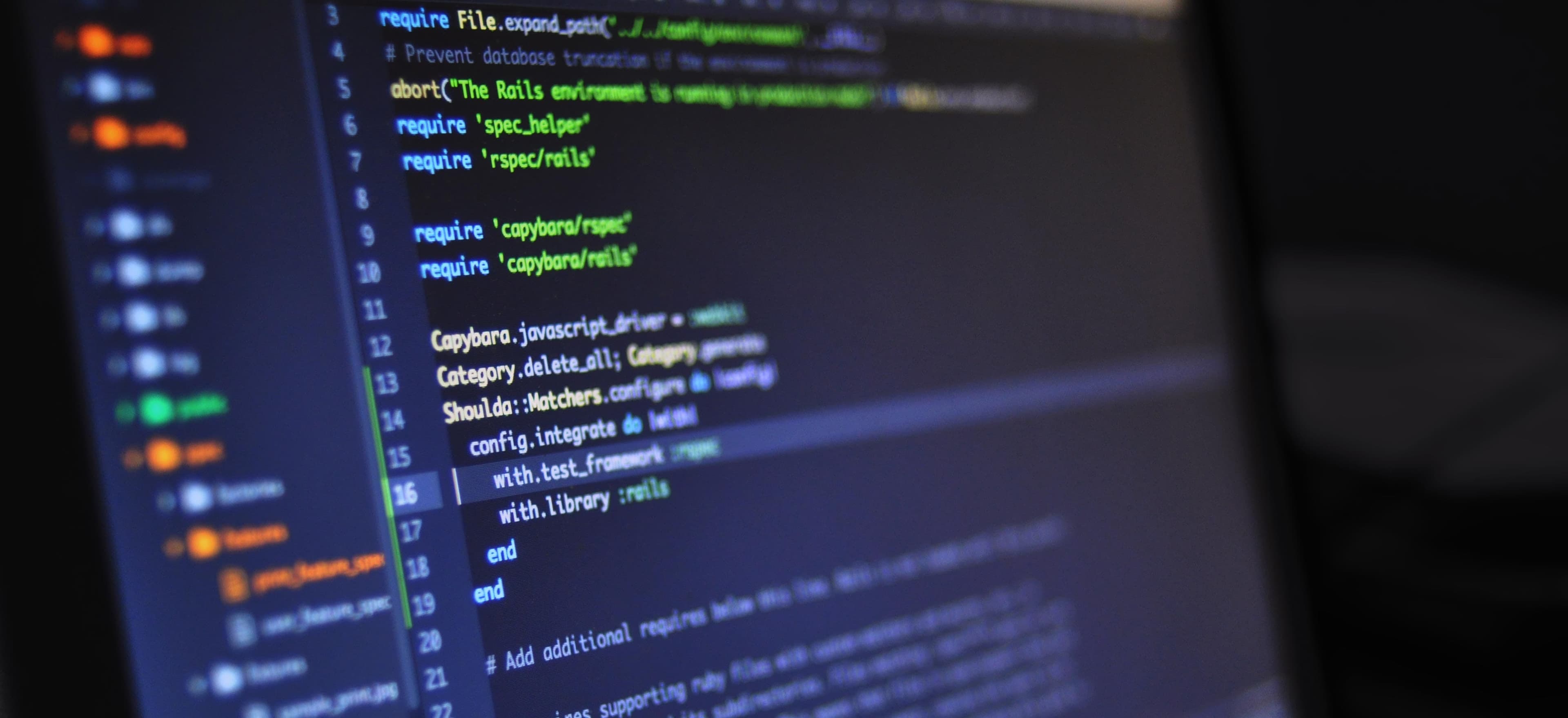
- Published on
Troubleshooting Connectivity Issues with Hawt.io and Apache jclouds
In this article, we will explore common connectivity issues that may arise when working with Hawt.io and Apache jclouds in a Java environment. Both Hawt.io and Apache jclouds are powerful tools, but sometimes troubleshooting connectivity problems can be challenging. We'll walk through some common issues and provide solutions to help you overcome these obstacles.
Understanding Hawt.io and Apache jclouds
Hawt.io is a web-based tool for visualizing and managing Java components, while Apache jclouds is an open-source library that provides an abstraction layer for cloud service providers. When these two tools are used together, they can provide a powerful platform for managing and interacting with cloud resources.
Common Connectivity Issues
Issue 1: Authentication Failure
One common issue when working with Apache jclouds is authentication failure when trying to connect to a cloud provider. This can be due to incorrect credentials or misconfiguration.
Solution:
First, double-check your credentials to ensure they are correct. If you are using environment variables or configuration files for authentication, make sure they are properly set up.
Issue 2: Network Connectivity Problems
Another common issue is network connectivity problems. This can manifest as timeouts or connection refused errors when trying to communicate with cloud services via jclouds.
Solution:
Check your network settings and ensure that the necessary ports are open for communication with the cloud provider. Additionally, verify that there are no firewall restrictions preventing outbound connections.
Issue 3: SSL/TLS Handshake Failures
SSL/TLS handshake failures can occur when there are issues with the SSL certificate validation or when the server's SSL/TLS configuration is not compatible with the client's.
Solution:
If you encounter SSL/TLS handshake failures, make sure that the SSL certificates are up to date and properly configured. Additionally, check the SSL/TLS configuration of the server and ensure it is compatible with the client's configuration.
Troubleshooting Steps
Here are some general troubleshooting steps you can take to address connectivity issues when working with Hawt.io and Apache jclouds:
-
Check Logs: Review the logs for any error messages or stack traces that may provide insight into the connectivity issues.
-
Verify Credentials: Double-check the credentials used for authentication and ensure they are accurate.
-
Network Configuration: Validate the network configuration, including firewall settings and port access.
-
SSL/TLS Configuration: Verify the SSL/TLS configuration and ensure it is compatible with the server's settings.
Example Code
Let's take a look at an example of code using Apache jclouds to create a connection to an AWS EC2 instance and retrieve its information:
import org.jclouds.ContextBuilder;
import org.jclouds.ec2.EC2Api;
import org.jclouds.ec2.EC2ApiMetadata;
import org.jclouds.ec2.domain.Instance;
import org.jclouds.ec2.features.InstanceApi;
import com.google.common.collect.ImmutableSet;
import com.google.common.io.Closeables;
public class EC2Example {
public static void main(String[] args) {
String accessKey = "your-access-key";
String secretKey = "your-secret-key";
EC2Api ec2Api = ContextBuilder.newBuilder("aws-ec2")
.credentials(accessKey, secretKey)
.buildApi(EC2ApiMetadata.class);
InstanceApi instanceApi = ec2Api.getInstanceApi().get();
for (Instance instance : instanceApi.describeInstancesInRegion(null, ImmutableSet.of())) {
System.out.println(instance);
}
Closeables.closeQuietly(ec2Api);
}
}
In this example, we use Apache jclouds to connect to the AWS EC2 API and retrieve information about instances. The ContextBuilder
is used to create a connection to the EC2 API, and the credentials are provided using the credentials
method.
Key Takeaways
Troubleshooting connectivity issues with Hawt.io and Apache jclouds can be a complex task, but by understanding common problems and following structured troubleshooting steps, you can effectively diagnose and resolve connectivity issues in your Java applications. Remember to verify credentials, check network and SSL/TLS configurations, and review logs for error messages to identify and address connectivity problems. Keep in mind that thorough understanding of the underlying concepts and libraries is crucial for successful troubleshooting.
By following the tips and steps outlined in this article, you'll be better equipped to tackle connectivity issues and ensure smooth interactions with cloud resources using Hawt.io and Apache jclouds in your Java projects. Happy troubleshooting!