Optimizing Continuous Integration for Software Development
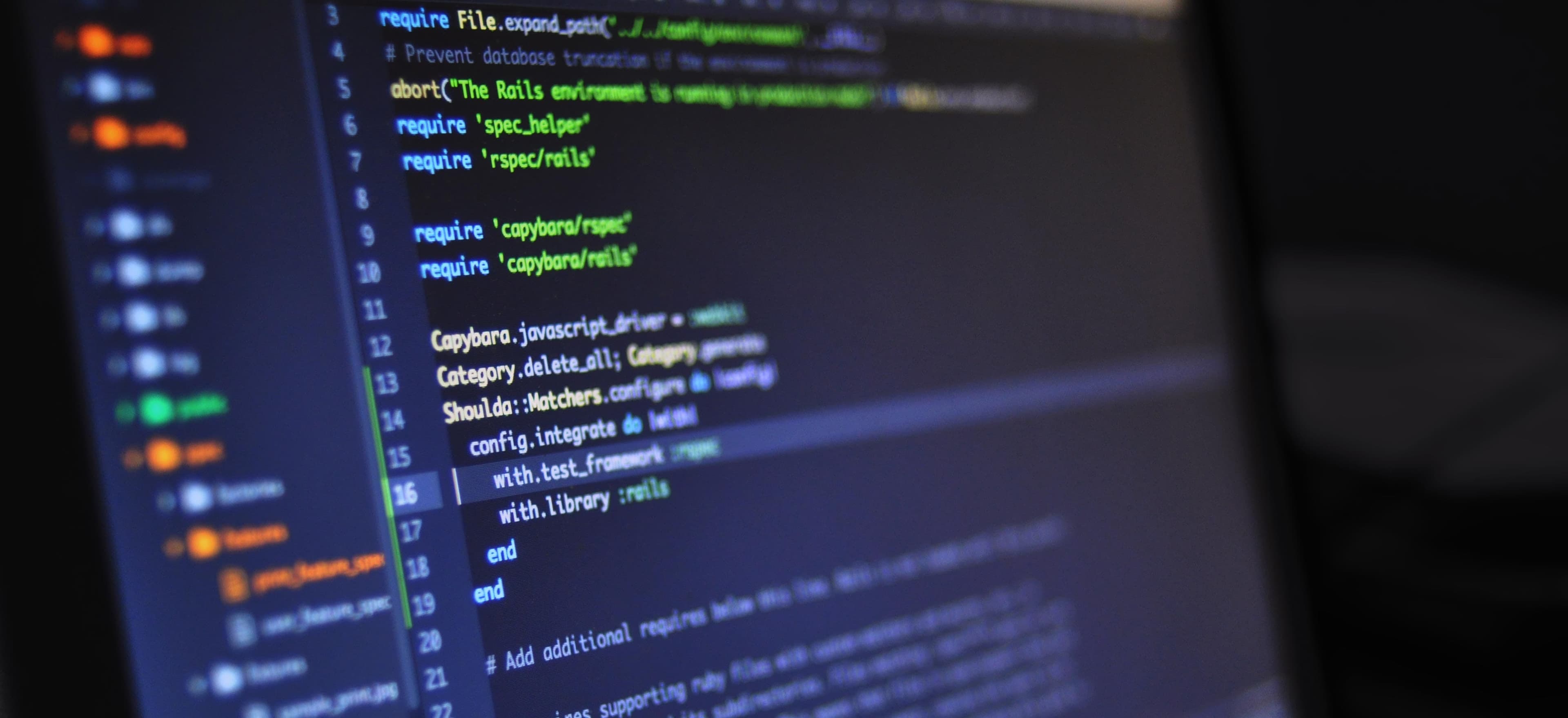
- Published on
Optimizing Continuous Integration for Software Development
In the fast-paced world of software development, continuous integration (CI) has become an essential practice. It allows development teams to integrate their code into a shared repository frequently, detecting errors early and enabling quicker identification and resolution of integration issues. In this blog post, we will explore how to optimize continuous integration using Java, a popular and versatile programming language.
Understanding Continuous Integration
At its core, continuous integration is about frequently integrating code changes into a central repository. This process is automated, allowing teams to detect issues early and consistently. As a result, it reduces the overhead of fixing integration problems and enables teams to deliver high-quality software at a rapid pace.
Setting Up Continuous Integration with Java
Choose the Right CI Tool
There are several Continuous Integration tools available, such as Jenkins, Travis CI, and CircleCI. For the purpose of this post, let's consider Jenkins, as it is highly versatile and widely used in Java projects.
Writing Automated Tests
One of the key elements of continuous integration is automated testing. With Java, popular testing frameworks such as JUnit and TestNG provide robust support for creating automated tests. Writing comprehensive test suites ensures that code changes can be validated automatically.
Integrating a Version Control System
A version control system like Git is essential for effective continuous integration. It allows developers to work collaboratively, while also providing a history of changes. Tools like GitHub and Bitbucket offer seamless integration with Jenkins, enabling automated builds triggered by code commits.
Optimizing Continuous Integration in Java
Parallel Test Execution
By leveraging parallel test execution, teams can significantly reduce the overall test execution time. In Java, the TestNG framework provides native support for parallel test execution, allowing tests to run concurrently and expedite the feedback loop.
@Test
public class ParallelTestExecution {
@Test
public void testMethod1() {
// Test logic
}
@Test
public void testMethod2() {
// Test logic
}
}
Continuous Inspection and Code Quality
Incorporating static code analysis tools like Checkstyle, FindBugs, or PMD into the CI pipeline can help maintain code quality standards. These tools analyze the codebase for potential issues, style violations, and best practice adherence, ensuring that the codebase remains clean and maintainable.
Gradle and Maven Build Optimization
Utilizing build tools such as Gradle or Maven can streamline the build process in Java projects. By optimizing the build scripts and dependencies, teams can enhance the efficiency of the CI pipeline, resulting in faster build times and more reliable builds.
The Bottom Line
Continuous Integration is a fundamental practice that empowers development teams to iterate faster and deliver high-quality software. By optimizing continuous integration in Java projects through parallel test execution, code quality checks, and build optimization, teams can significantly enhance their development workflow. Embracing these best practices not only fosters a culture of quality and reliability but also accelerates the delivery of value to end-users.
In the constantly evolving landscape of software development, continuous integration remains a cornerstone practice, and its effective implementation is crucial for success in today's competitive market.
By following the best practices and leveraging the right tools, Java developers can ensure that their CI pipeline is not just efficient, but also robust and reliable. As development teams continue to push the boundaries of innovation, optimizing continuous integration will play a pivotal role in shaping the future of software development.