Troubleshooting Java Thread at Runnable State
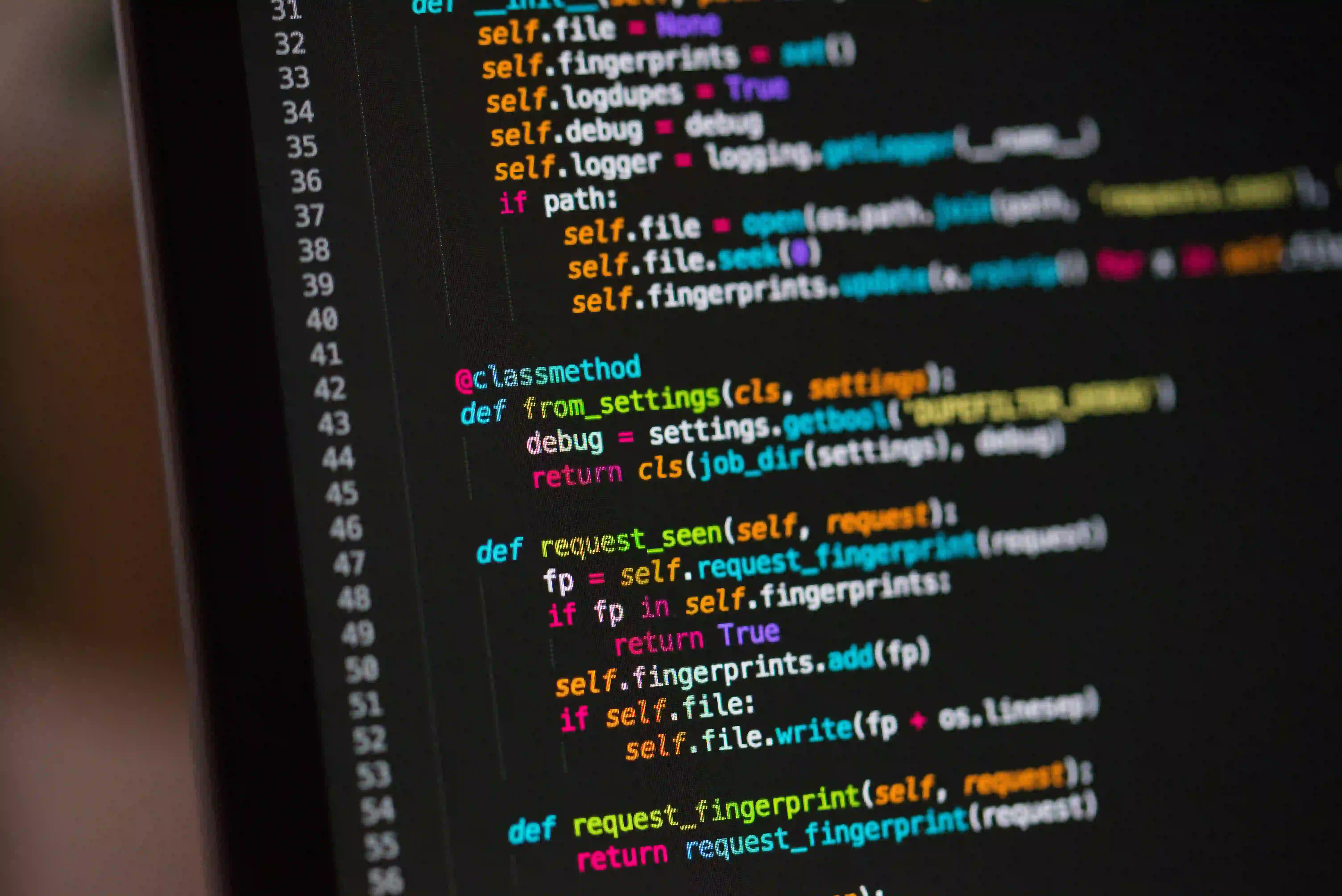
Troubleshooting Java Thread at Runnable State: A Comprehensive Guide
Java is a powerful and versatile programming language that allows for the creation and management of multithreaded applications. Threads in Java can exist in various states, and one of the most crucial states is the runnable state. It's important to understand how to troubleshoot a Java thread when it is in this state to ensure optimal performance and stability of your application.
In this guide, we will delve into the runnable state of Java threads, explore common issues that may arise, and provide detailed troubleshooting steps to resolve these issues effectively.
Understanding the Runnable State
In Java, a thread enters the runnable state when it is eligible to run but the scheduler has not selected it to be the running thread. This means the thread is ready to execute and is waiting for the opportunity to do so. The transition to the running state occurs when the scheduler selects the thread to run.
Common Issues in the Runnable State
Issue 1: Thread Starvation
Thread starvation can occur when a thread is in the runnable state but is not being selected by the scheduler to enter the running state. This can lead to performance degradation and slow processing of tasks.
Issue 2: Synchronization Problems
When multiple threads are in the runnable state and accessing shared resources without proper synchronization, it can lead to data inconsistency and potential deadlock situations.
Issue 3: Priority Inversion
In a scenario where a high-priority thread is waiting for a resource held by a lower-priority thread in the runnable state, priority inversion can occur, impacting the overall performance of the system.
Troubleshooting Steps
Step 1: Thread Dump Analysis
A thread dump provides valuable insights into the state of all threads in a Java application. It enables the identification of threads in the runnable state and helps uncover potential synchronization issues and thread contention.
// Example: Generating a thread dump programmatically
ThreadMXBean threadMXBean = ManagementFactory.getThreadMXBean();
ThreadInfo[] threadInfos = threadMXBean.dumpAllThreads(true, true);
for (ThreadInfo info : threadInfos) {
System.out.println(info.getThreadName() + " : " + info.getThreadState());
}
Why: Analyzing the thread dump allows you to pinpoint threads stuck in the runnable state and identify any potential bottlenecks in the application's threading model.
Step 2: Synchronization and Lock Analysis
Review the sections of code where shared resources are accessed by multiple threads. Ensure proper synchronization mechanisms such as synchronized
blocks, locks, or concurrent data structures are utilized to prevent data races and ensure thread safety.
// Example: Using synchronized block for thread-safe access to shared resource
synchronized (sharedResource) {
// Access and modify the shared resource
}
Why: Implementing proper synchronization mechanisms is crucial to mitigate synchronization problems and prevent data inconsistencies when threads are in the runnable state.
Step 3: Priority Management
Evaluate the thread priorities in the application and ensure they are set appropriately to prevent priority inversion issues. Consider the use of thread pools and executor services to manage thread priorities effectively.
// Example: Setting thread priority
Thread highPriorityThread = new Thread(runnable);
highPriorityThread.setPriority(Thread.MAX_PRIORITY);
Why: Proper management of thread priorities is essential to mitigate priority inversion and ensure efficient utilization of system resources.
Best Practices for Maintaining Threads in the Runnable State
-
Use Thread Pools: Employing thread pools via
ExecutorService
helps manage and reuse threads efficiently, minimizing the time threads spend in the runnable state. -
Optimize Synchronization: Review and optimize synchronization mechanisms to reduce contention and enhance the responsiveness of threads in the runnable state.
-
Monitor Thread Activity: Implement monitoring and logging of thread activity to identify any irregularities and proactively address potential issues related to the runnable state.
The Closing Argument
Understanding the nuances of the runnable state of Java threads is crucial for developing robust and high-performance multithreaded applications. By addressing common issues and following best practices for troubleshooting and thread management, developers can ensure the smooth execution and optimal utilization of threads in the runnable state.
By applying the troubleshooting steps outlined in this guide and adhering to best practices, developers can effectively mitigate issues related to Java threads in the runnable state and maintain the stability and efficiency of their applications.