Struggling to Manage Pull Requests?
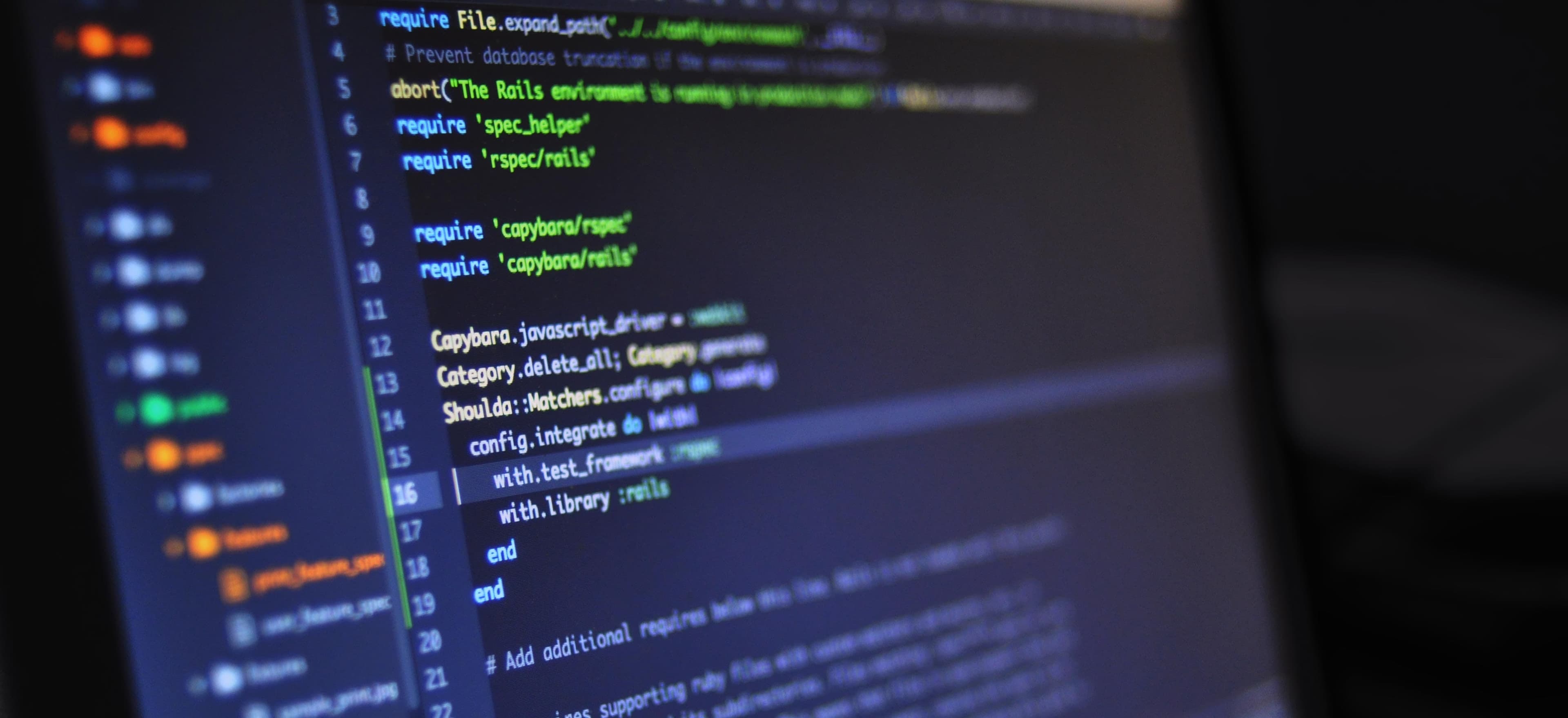
- Published on
Are you finding it challenging to manage and review pull requests effectively in your Java projects? Pull requests are a fundamental aspect of the software development process, allowing teams to collaborate, review code, and maintain the quality of their codebase. However, without the right strategies and tools, pull request management can become burdensome and inefficient. In this blog post, we will explore some best practices and tools that can help you streamline the pull request process in your Java projects, saving time and enhancing code quality.
Understanding Pull Requests
Before diving into pull request management, let's briefly understand what pull requests are and their significance in the development workflow.
In a nutshell, a pull request is a request to merge a feature branch into the main branch of a repository. It serves as a mechanism for code review, where team members can examine the proposed changes, provide feedback, and discuss any potential issues before incorporating the code into the project.
Best Practices for Pull Request Management in Java Projects
Effective pull request management involves a combination of efficient processes and the right set of tools. Here are some best practices to consider when managing pull requests in your Java projects.
1. Use a Consistent Coding Style
Maintaining a consistent coding style is crucial for improving code readability and maintainability. Consider using tools like CheckStyle or Google Java Style Guide to enforce a consistent coding style across your Java codebase. Integrating these tools into your build process can automatically flag style violations, making it easier for developers to adhere to the defined coding standards.
// Example of a method violating the Google Java Style Guide
public void myMethod() {
// Method body
}
Using Google Java Style Guide, the method should be formatted as:
// Corrected method formatting according to Google Java Style Guide
public void myMethod() {
// Method body
}
2. Automated Code Analysis
In addition to style consistency, automated code analysis tools like FindBugs and PMD can help identify potential bugs, performance issues, and other code quality issues in your Java codebase. Integrating these tools into your build process or IDE can provide developers with instant feedback, leading to early detection and resolution of potential issues.
3. Continuous Integration and Pull Requests
Integrate a robust continuous integration (CI) system such as Jenkins or Travis CI with your repository to automatically build and test code changes for each pull request. This ensures that new changes do not introduce build or test failures, and it provides an additional layer of assurance before merging code into the main branch.
4. Utilize Code Reviews Effectively
Code reviews are essential for maintaining code quality, sharing knowledge, and catching potential issues early on. Encourage thorough and constructive code reviews within your team. Tools like GitHub or Bitbucket provide features for inline commenting, facilitating discussions and feedback exchange during code reviews.
Streamlining Pull Request Management with Tools
While following best practices is crucial, leveraging the right tools can significantly streamline the pull request management process in your Java projects.
1. GitHub Pull Request Workflow
If your Java projects are hosted on GitHub, utilizing GitHub's native pull request workflow can be immensely beneficial. GitHub provides a seamless interface for creating, reviewing, and managing pull requests, along with integrations with various CI systems and code analysis tools. Additionally, GitHub Actions allows you to automate workflows, such as running tests or code analysis, based on pull request events.
2. Bitbucket Pull Request Insights
For teams using Bitbucket for Java development, Bitbucket offers pull request insights, which provide valuable metrics and visualizations for pull request activity, reviewer turnaround times, and merge frequencies. These insights can help identify potential bottlenecks in the review process and improve overall efficiency.
3. IDE Integrations
Many popular Integrated Development Environments (IDEs) like IntelliJ IDEA, Eclipse, and NetBeans offer integrations with version control systems like Git, providing seamless support for creating and reviewing pull requests directly within the IDE. This allows developers to stay within their familiar development environment while engaging in code reviews and managing pull requests.
My Closing Thoughts on the Matter
Efficient pull request management is essential for fostering collaboration and maintaining code quality in Java projects. By adhering to best practices, leveraging automated tools, and utilizing platform-specific workflows and integrations, teams can streamline the pull request process and ensure that code changes are thoroughly reviewed and tested before being merged.
Managing pull requests effectively not only improves code quality but also fosters a culture of collaboration and knowledge sharing within the development team. Investing time and effort into optimizing your pull request management process can yield substantial benefits in the long run.
In conclusion, pull request management in Java projects is not just about merging code changes; it's about facilitating constructive feedback, promoting code quality, and ultimately delivering reliable software products.
If you want to take your pull request management to the next level, feel free to explore the tools and best practices mentioned in this post and adapt them to fit your team's specific needs and workflows. Happy coding and collaborating!