Optimizing Java Development for Reliable Software Deployment
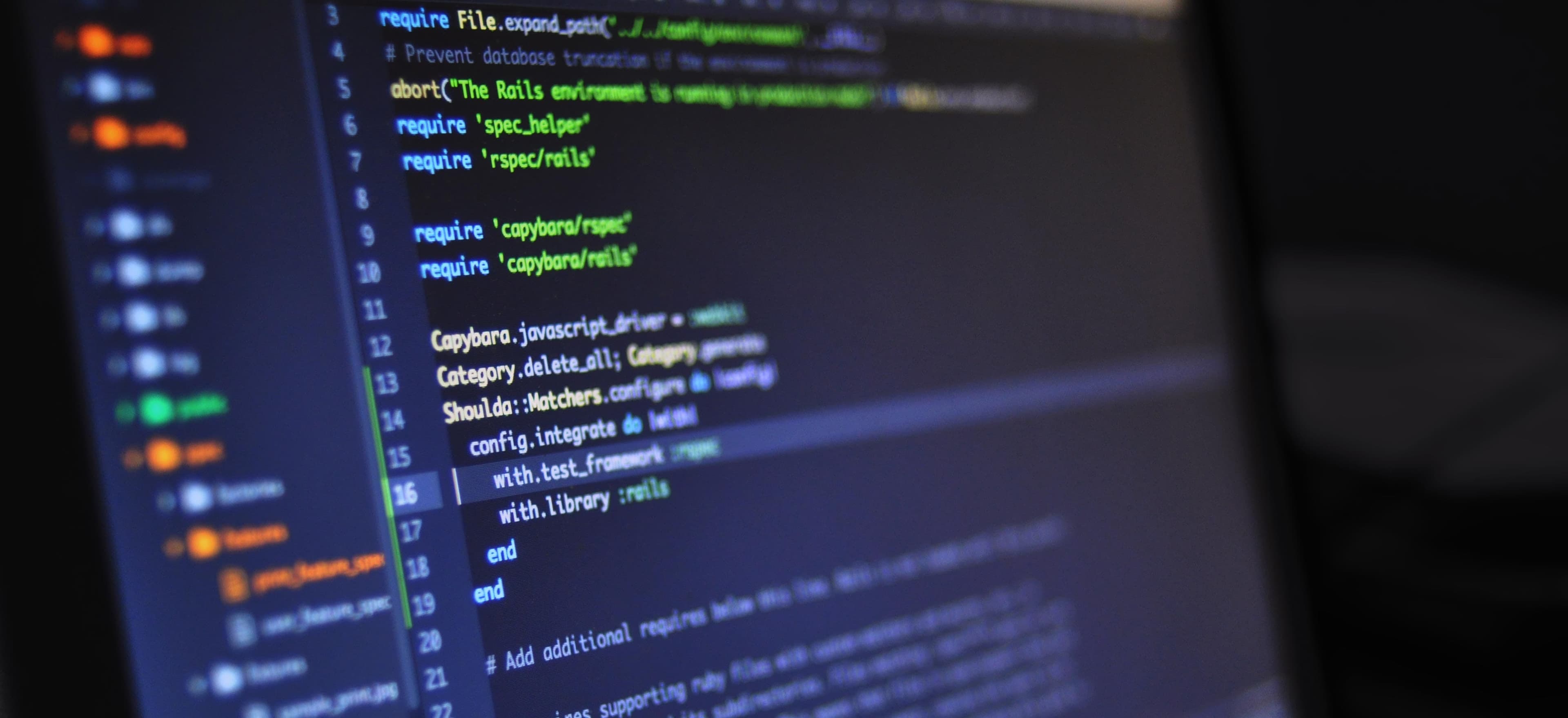
- Published on
Optimizing Java Development for Reliable Software Deployment
When it comes to developing and deploying software with Java, there are a plethora of techniques and best practices to ensure that the end product is robust, reliable, and efficient. In this article, we will explore some key strategies and methodologies to optimize Java development for reliable software deployment.
Writing Clean and Maintainable Code
One of the fundamental principles of developing reliable Java software is to write clean and maintainable code. This involves adhering to coding conventions, using meaningful variable and method names, and following best practices such as the Single Responsibility Principle and the DRY (Don't Repeat Yourself) principle.
// Example of clean and maintainable code
public class OrderService {
public void processOrder(Order order) {
validateOrder(order);
calculateOrderTotal(order);
saveOrderToDatabase(order);
}
private void validateOrder(Order order) {
// Validation logic
}
private void calculateOrderTotal(Order order) {
// Calculation logic
}
private void saveOrderToDatabase(Order order) {
// Database interaction logic
}
}
In the above code snippet, the OrderService
class follows the Single Responsibility Principle by encapsulating the logic related to processing an order. The methods within the class have clear and descriptive names, making the code easy to understand and maintain.
Leveraging Automated Testing
Automated testing plays a crucial role in ensuring the reliability of Java software. By writing unit tests, integration tests, and end-to-end tests, developers can validate the functionality of their code and catch potential issues early in the development cycle.
// Example of a unit test using JUnit
public class OrderServiceTest {
@Test
public void testProcessOrder() {
Order order = createTestOrder();
OrderService orderService = new OrderService();
orderService.processOrder(order);
assertTrue(order.isProcessed());
}
private Order createTestOrder() {
// Test data initialization
}
}
In the above test case written with JUnit, the testProcessOrder
method validates that the processOrder
method of the OrderService
class correctly processes an order. Automated tests provide a safety net for refactoring and making changes to the codebase, ensuring that the software remains reliable throughout its lifespan.
Embracing Continuous Integration and Deployment (CI/CD)
Adopting a CI/CD pipeline is essential for streamlining the deployment process and ensuring the continuous delivery of reliable software. Tools such as Jenkins, Travis CI, and GitLab CI/CD enable automating build, test, and deployment processes, allowing for rapid iteration and reliable releases.
By integrating automated testing into the CI/CD pipeline, developers can ensure that new code changes do not introduce regressions and that the software remains in a deployable state at all times.
Managing Dependencies with Maven or Gradle
A reliable software deployment depends on effectively managing project dependencies. Tools like Apache Maven and Gradle facilitate the management of Java libraries and external dependencies, ensuring that the correct versions are used and that the build process is reproducible.
Using a declarative approach, Maven and Gradle allow developers to define project dependencies, plugins, and build configurations in a structured manner. This not only simplifies the build process but also contributes to the reliability of the deployment by reducing dependency-related issues.
Securing Sensitive Information
In the context of software deployment, securing sensitive information such as credentials, API keys, and encryption keys is of paramount importance. Utilizing tools like Java KeyStore for managing cryptographic keys and libraries like Apache Commons Configuration for handling configuration properties can enhance the security and reliability of the deployed software.
// Example of accessing sensitive information using Apache Commons Configuration
String databaseUsername = config.getString("database.username");
String databasePassword = config.getString("database.password");
// Use the credentials securely
By centralizing and encrypting sensitive information, developers can mitigate the risk of unauthorized access and maintain the reliability of the deployed software.
Monitoring and Logging
Effective monitoring and logging are crucial for identifying and diagnosing issues in a deployed Java application. Integrating logging frameworks such as Log4j or SLF4J allows developers to capture relevant information about the application's behavior and detect potential errors or performance bottlenecks.
Furthermore, leveraging monitoring tools like Prometheus and Grafana enables proactive insights into the application's health and performance, contributing to the overall reliability of the deployed software.
Closing the Chapter
In conclusion, optimizing Java development for reliable software deployment entails a multifaceted approach that encompasses clean code practices, automated testing, CI/CD integration, dependency management, security considerations, and robust monitoring. By adhering to these best practices and leveraging the appropriate tools, developers can ensure the consistent reliability and performance of their deployed Java software.
To delve deeper into Java development best practices, you may find the Java documentation and Baeldung to be valuable resources. Happy coding!
Remember, in the world of Java development, reliability is not a feature, but a necessity!