Managing Microservices in DevOps: Minimizing Change Costs
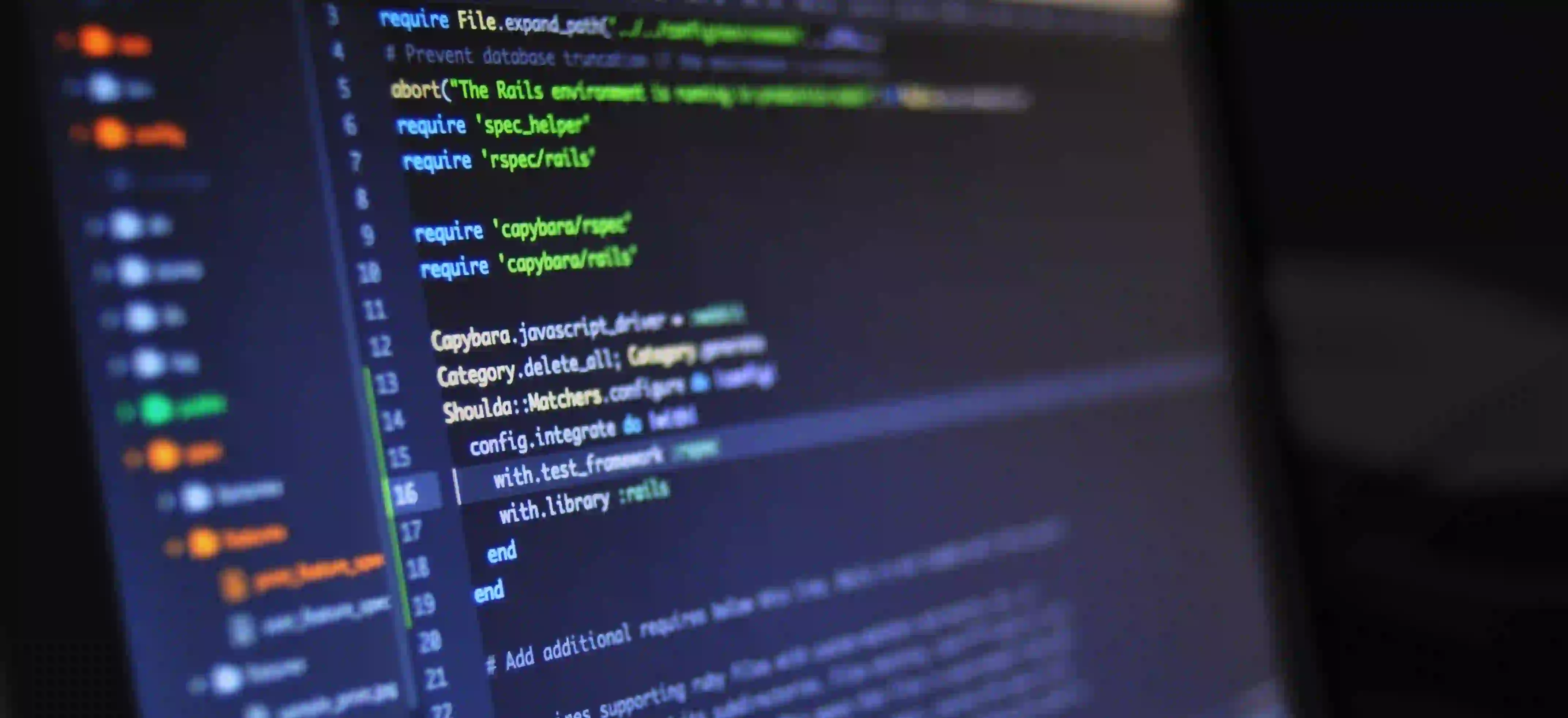
Managing Microservices in DevOps: Minimizing Change Costs
In the fast-paced world of DevOps, managing microservices can be a daunting task. With the ever-changing nature of microservices and the need to continuously deploy updates, it's important to minimize change costs to ensure a seamless and efficient development process.
Understanding Microservices in DevOps
Microservices have gained popularity in the software development industry due to their ability to break down monolithic applications into smaller, independently deployable services. This approach allows for greater flexibility, scalability, and agility in the development and deployment of applications.
In a DevOps environment, microservices play a crucial role in enabling teams to work independently on different services, leading to faster development cycles and quicker time-to-market. However, with the benefits of microservices come challenges, particularly in managing the changes and updates across multiple services.
Minimizing Change Costs in Microservices
To effectively manage microservices in a DevOps environment and minimize change costs, several best practices and strategies can be employed.
Version Control and Dependency Management
Version control systems, such as Git, play a crucial role in managing the codebase of microservices. By maintaining a clear versioning strategy and effectively managing dependencies, teams can minimize the impact of changes and updates on interconnected services.
// Example of versioning in build.gradle for a Java microservice
dependencies {
implementation 'com.example:serviceA:1.0.0'
}
In the above example, 'serviceA' is referenced with a specific version (1.0.0), ensuring that updates to 'serviceA' do not unexpectedly impact dependent services.
Automated Testing and Continuous Integration
Implementing automated testing and continuous integration pipelines is essential in minimizing change costs. By automated testing, teams can quickly identify any regressions or issues caused by updates, ensuring the stability and reliability of microservices.
// Example of a JUnit test for a microservice endpoint
@Test
public void testEndpointSuccess() {
// perform the test and assert the expected outcome
// ...
}
Continuous integration pipelines can automatically build, test, and deploy microservices, providing immediate feedback on the impact of changes.
Service Contracts and API Design
Defining clear service contracts and maintaining stable API designs can significantly reduce change costs in microservices. By adhering to a contract-first approach, teams can ensure that changes to service interfaces are backward compatible, minimizing disruptions to dependent services.
// Example of a RESTful API contract using OpenAPI Specification (Swagger)
openapi: '3.0.0'
info:
title: Example API
version: 1.0.0
By documenting and adhering to a standardized API design, teams can minimize the need for widespread changes when updating microservices.
Monitoring and Observability
Implementing robust monitoring and observability practices is essential in identifying potential issues and performance bottlenecks caused by changes in microservices. By proactively monitoring the health and performance of services, teams can quickly address any issues that arise from updates, minimizing the overall impact on the system.
To Wrap Things Up
In a DevOps environment, managing microservices effectively is crucial in minimizing change costs and ensuring a smooth development and deployment process. By implementing version control and dependency management, automated testing and continuous integration, clear service contracts and API design, and robust monitoring and observability, teams can mitigate the impact of changes in microservices.
Ultimately, minimizing change costs in microservices is a continuous effort that requires a combination of best practices, tools, and a proactive approach to ensure the seamless operation of microservices in a DevOps environment.
Incorporating these strategies and best practices can enable teams to unleash the full potential of microservices while minimizing the disruptions caused by changes and updates.
For further reading, check out how Kubernetes can streamline the management of microservices in a DevOps environment. Additionally, learn about Continuous Delivery practices to optimize the deployment of microservices.
Remember, effective management of microservices in DevOps is key to achieving agility and innovation while minimizing change costs.