Optimizing Java Application Performance: Survey Highlights
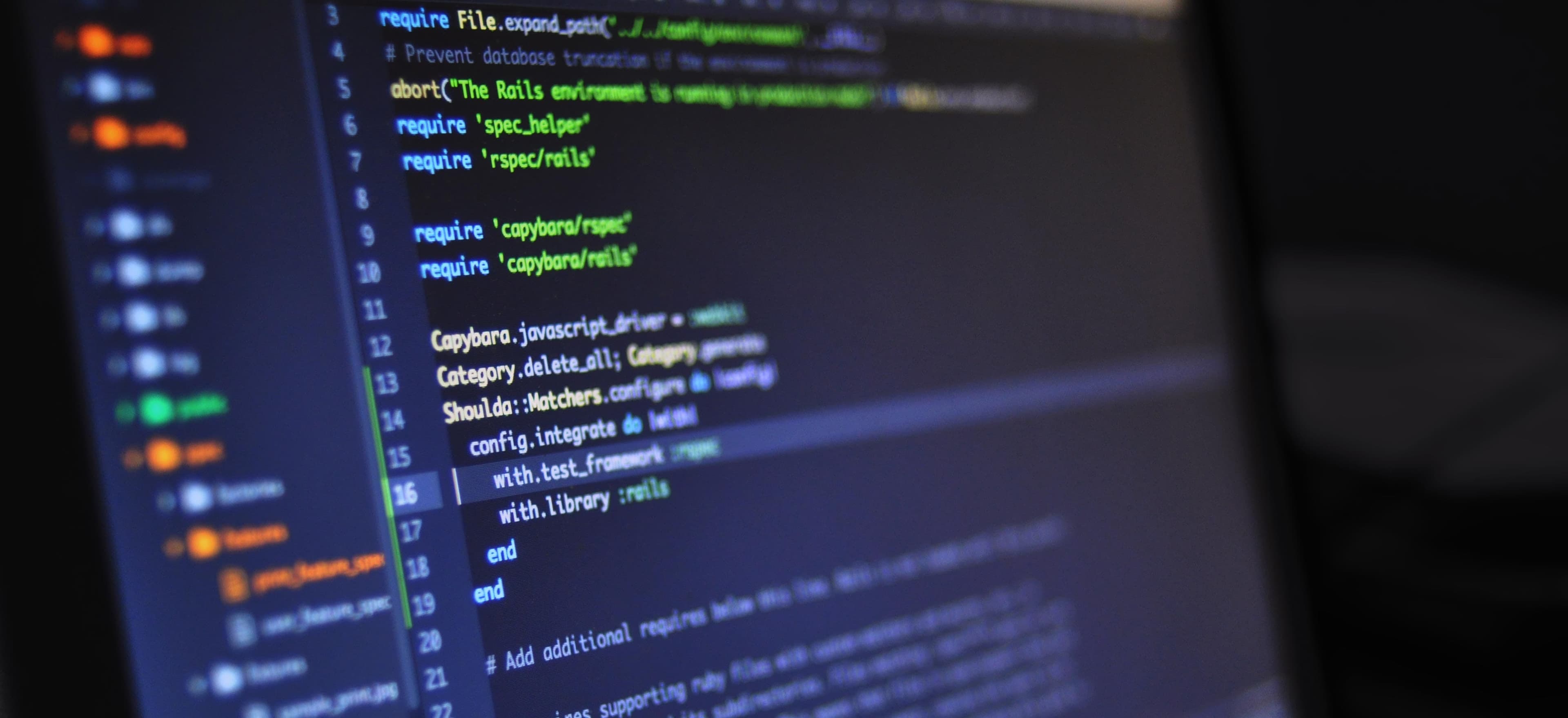
- Published on
Optimizing Java Application Performance: Survey Highlights
Java has been one of the most widely used programming languages for decades, and with its versatility and robustness, it powers a myriad of applications across various domains. However, as applications scale and evolve, performance optimization becomes crucial to ensure seamless user experience and efficient resource utilization. In a recent survey of Java developers and performance engineers, several key strategies and best practices emerged for optimizing Java application performance. In this post, we delve into the survey highlights and explore actionable insights for maximizing Java application performance.
1. Profiling and Monitoring
One of the fundamental findings of the survey was the paramount importance of profiling and monitoring in optimizing Java application performance. Utilizing tools such as JProfiler, YourKit Java Profiler, and VisualVM enables developers to identify performance bottlenecks, memory leaks, and resource-intensive code segments. By pinpointing these inefficiencies, developers can strategically focus on optimizing critical areas, ultimately enhancing the overall performance of the application.
2. Efficient Memory Management
Java's automatic memory management system, facilitated by garbage collection, is a double-edged sword. While it relieves developers from manual memory allocation and deallocation, improper memory management can lead to performance degradation. The survey revealed that optimizing memory usage through techniques such as object pooling, minimizing object creation, and using memory profiling tools like Eclipse MAT significantly impacts Java application performance. By minimizing memory overhead and reducing garbage collection pressure, applications can operate more efficiently.
3. Multithreading and Concurrency
Multithreading plays a vital role in Java application performance optimization, especially in scenarios involving parallel processing and I/O operations. The survey highlighted the significance of utilizing concurrent data structures, thread pooling, and asynchronous programming to leverage the full potential of multicore processors. Additionally, employing tools like Java Concurrency Tools and Project Loom can streamline multithreading and concurrency management, thereby enhancing application responsiveness and throughput.
ExecutorService executor = Executors.newFixedThreadPool(4);
for (int i = 0; i < n; i++) {
executor.execute(new Task(i));
}
executor.shutdown();
The above code snippet demonstrates the usage of a thread pool to efficiently execute multiple tasks concurrently, thereby improving overall performance by parallelizing workload.
4. Just-In-Time (JIT) Compilation and Optimization
The Java Virtual Machine (JVM) incorporates JIT compilation to dynamically optimize hotspots in the code during runtime. The survey emphasized the critical role of JIT compilation in enhancing Java application performance. By profiling and analyzing JIT compiler reports, developers can identify code segments that are ripe for optimization. Furthermore, exploring advanced JIT optimization techniques, such as method inlining and loop unrolling, can yield substantial performance improvements in CPU-bound operations.
5. Efficient Data Structures and Algorithms
The choice of data structures and algorithms significantly impacts the efficiency of Java applications. Utilizing the appropriate data structures, such as HashMap, ConcurrentHashMap, and TreeMap, based on specific use cases can mitigate performance bottlenecks. Furthermore, employing algorithmic optimizations, like reducing time complexity and minimizing unnecessary iterations, can bolster application performance.
Final Considerations
In a landscape where user expectations for application responsiveness and efficiency are continually rising, optimizing Java application performance is imperative. The survey findings underscore the significance of profiling and monitoring, efficient memory management, multithreading, JIT compilation, and judicious use of data structures and algorithms in maximizing Java application performance. By integrating these insights into development practices, Java developers can elevate the performance of their applications, ensuring they deliver a seamless and responsive user experience.
Optimizing Java application performance is an ongoing journey, and staying abreast of emerging tools, techniques, and best practices is paramount. Embracing a performance-oriented mindset from the outset of development and continuously refining the application based on profiling results and benchmarks are integral to achieving optimal performance. As Java continues to evolve, the quest for superior application performance remains a dynamic and pivotal aspect of software development.