Troubleshooting Common Issues in Spring Boot Integration Tests
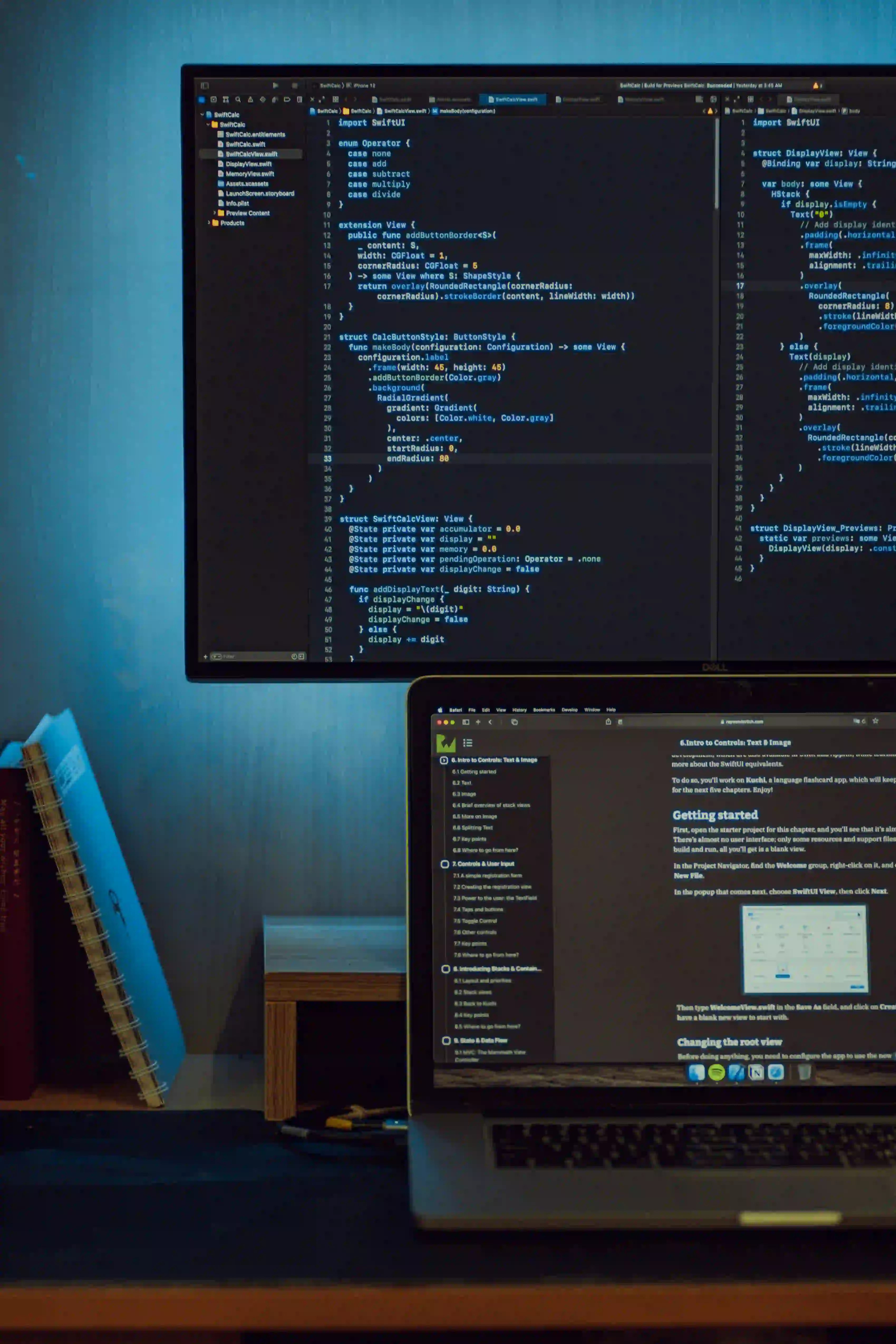
Troubleshooting Common Issues in Spring Boot Integration Tests
Integration testing is an important aspect of software development as it ensures that various parts of the system work together as expected. In the context of Java development, Spring Boot provides a powerful framework for building and testing applications. However, integration testing in Spring Boot can sometimes present challenges that require troubleshooting. In this blog post, we will discuss some common issues that developers may encounter when writing integration tests in Spring Boot and how to troubleshoot them.
Issue 1: Database Configuration
Problem:
When running integration tests that involve database interactions, you may encounter issues related to database configuration, such as connection errors or data inconsistencies.
Troubleshooting:
- Ensure that the database configuration in the test environment is consistent with the production environment.
- Use an in-memory database like H2 for integration tests to avoid interference with the development or production databases.
- Verify that the database schema is created and initialized properly before running the tests.
Example:
@SpringBootTest
@AutoConfigureTestDatabase
class MyIntegrationTest {
@Test
void shouldSaveAndRetrieveData() {
// Test logic here
}
}
In this example, @AutoConfigureTestDatabase
annotation configures the test database based on the environment, allowing for seamless integration testing without impacting the actual database.
Issue 2: External Dependencies
Problem:
Integration tests often require interactions with external services or dependencies, such as REST APIs, message queues, or third-party services. Issues related to connectivity or compatibility with these external dependencies can arise.
Troubleshooting:
- Mock the external dependencies using tools like Mockito to isolate the tests from external factors.
- Use tools like WireMock to create stubs of REST APIs for predictable responses.
- Verify the compatibility of external services with the testing environment, such as network connectivity and access permissions.
Example:
@Test
void shouldRetrieveDataFromExternalService() {
// Mock the external service using Mockito
when(externalService.getData()).thenReturn(someTestData);
// Test logic that relies on the external service
}
By mocking the external service, the integration test focuses solely on the functionality of the component being tested, without being affected by the availability or behavior of the actual external service.
Issue 3: Environment Configuration
Problem:
Differences in environment configuration between development, testing, and production environments can lead to unexpected behavior or failures in integration tests.
Troubleshooting:
- Use Spring profiles to define specific configurations for different environments.
- Provide test-specific configuration overrides to ensure consistent behavior during integration tests.
- Verify that environment-specific properties, such as credentials or endpoints, are correctly configured for the test environment.
Example:
@SpringBootTest
@ActiveProfiles("test")
class MyIntegrationTest {
@Value("${test.api.endpoint}")
private String apiEndpoint;
// Test methods here
}
By activating the "test" profile and using test-specific property values, the integration tests can run with an environment tailored for testing without impacting the production configuration.
The Last Word
Integration testing in Spring Boot can be a complex task, especially when dealing with external dependencies and environment-specific configurations. By being aware of common issues and applying the troubleshooting techniques discussed in this blog post, developers can effectively address challenges and ensure the reliability of their integration tests.
Remember, troubleshooting is an essential skill in software development, and understanding how to diagnose and resolve issues in integration tests will ultimately lead to more robust and reliable applications.
For more in-depth information about integration testing in Spring Boot, you can refer to the official Spring documentation.
Happy testing!
By addressing common issues in Spring Boot integration tests and providing troubleshooting tips, this blog post serves as a practical guide for developers looking to improve their testing practices. The structured format, concise yet informative sections, and relevant code examples make the content accessible and actionable.